ISRC¶
Description¶
Current source (for cap. touch and temperature measurement)
The ISRC peripheral is used to source a configurable current from its output node, which is connected to the positive input of the COMPA peripheral. Different current values can be selected by combining the defined current values, BV_ISRC_CURR_XYZ
.
The ISRC output pin is selected by compaSelectGpioInput()
, which also selects the input for the COMPA peripheral.
The primary application for the ISRC peripheral is capacitive touch sensing, where it is used together with the COMPA and TDC peripherals. ISRC will then drive an internal reference voltage for COMPA, nominally 0.8 V, while charging the capacitance that is also connected to the COMPA input. The time from start of charging until the COMPA output goes high is measured using the TDC.
When enabled, the ISRC output is either clamped to ground, or released to start sourcing the configured current.
For capacitive touch sensing, two different types of clamping can be selected:
- Analog + digital, using Analog + open-drain pins pins: Clamping is fast, pins remain clamped while not selected for measurement.
- Analog only, using Analog pins pins: Clamping is slow, pins float while not selected for measurement. Delay must be inserted between changing pin selection and releasing the clamp.
Temperature Dependency and Temperature Measurement¶
Note that the ISRC output is temperature dependent, and that this effect only cancels out when ISRC is also used as 0.8 V internal reference voltage for COMPA, as described above.
The temperature dependency can be used to measure temperature by running the ISRC output through an internal resistor between 2 and 100 kOm, and sample the resulting voltage using the ADC. See the example below for details, and note the following limitations:
- Gain, offset, and non-linearities vary from chip to chip and must be calibrated during production.
- None of the analog peripherals (ADC, COMPA, COMPB and ISRC) can be used for other purposes while measuring temperature.
Block Diagram¶
The block diagram shows signal routing for COMPA and ISRC (with I/O pins for 7x7 RGZ package):
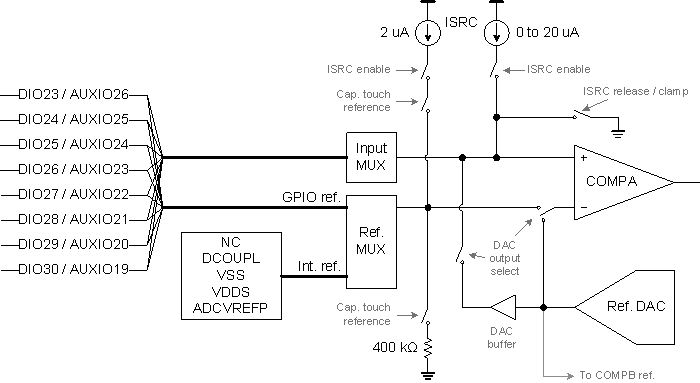
Examples¶
Capacitive Touch Sensing¶
// Enable COMPA, including 2 uA through 400 kOhm = 0.8 V reference voltage for COMPA
compaEnableWithCapTouchRef();
// Enable ISRC (6.5 uA)
U16 current = BV_ISRC_CURR_2P0U | BV_ISRC_CURR_4P5U;
isrcEnable(current);
// Select 2 x 48 MHz from RCOSC_HF as TDC counter clock source
tdcSetCntSource(TDC_CNTSRC_96M_RCOSC);
// Enable the TDC with start trigger on ISRC reset release and stop trigger on COMPA
tdcSetTriggers(TDC_STARTTRIG_ISRC_RELEASE, TDC_STOPTRIG_COMPA_HIGH, 0);
tdcEnable();
// For each pin ...
for (U16 n = 0; n < PIN_COUNT; n++) {
// Select COMPA input/ISRC output
compaSelectGpioInput(cfg.pAuxioAxdCapTouch[n]);
// Prepare the TDC and trigger start of measurement
tdcArm(TDC_START_ASYNC);
isrcRelease(cfg.pAuxioAxdCapTouch[n]);
// Wait for the TDC stop trigger for 80 us
tdcWaitUs(80);
// Re-clamp the pin to ground
isrcClamp(cfg.pAuxioAxdCapTouch[n]);
// Get the TDC counter value
tdcGetValue(output.pTdcValueH[n], output.pTdcValueL[n]);
}
// Disable COMPA, ISRC and TDC
tdcDisable();
isrcDisable();
compaDisable();
Temperature Measurement¶
// Enable ISRC with 11.75 uA output, and then enable temperature measurement with a
// 50 kOhm resistor. This gives a nominal output voltage of 587.5 mV, which will vary with
// temperature.
U16 current = BV_ISRC_CURR_11P75U;
isrcEnable(current);
isrcEnableTempMeasWithAdc(ISRC_TEMP_MEAS_RES_50_KOHM);
// For better precision, we can disable the ADC input scaling if this does not violate the
// ADC's maximum input rating. See the datasheet for details.
adcDisableInputScaling();
// Enable the ADC
adcEnableSync(ADC_REF_FIXED, ADC_SAMPLE_TIME_42P6_US, ADC_TRIGGER_MANUAL);
// Sample the temperature dependent voltage using the ADC. For better precision, perform
// multiple ADC measurements and calculate the sum or average of the resulting ADC values.
adcGenManualTrigger();
adcReadFifo(output.tempMeasAdcValue);
// Disable ADC, temperature measurement and ISRC. Note that:
// - isrcDisableTempMeasWithAdc() MUST always be called after temperature measurement
// - isrcDisableTempMeasWithAdc() MUST be called before isrcDisable()
adcDisable();
isrcDisableTempMeasWithAdc();
isrcDisable();
Procedures Overview¶
Name | Brief description |
compaSelectGpioInput() |
Selects a GPIO pin as input for COMPA. More … |
isrcClamp() |
Clamps the ISRC output to ground. More … |
isrcDisable() |
Disables the ISRC peripheral. More … |
isrcDisableTempMeasWithAdc() |
Disables temperature measurement using the temperature dependent output of ISRC. More … |
isrcEnable() |
Enables the ISRC peripheral with configuration of the output current. More … |
isrcEnableTempMeasWithAdc() |
Enables temperature measurement using the temperature dependent output of ISRC. More … |
isrcRelease() |
Releases the ISRC output (removing the clamp to ground). More … |
Constants¶
Name | Description |
BV_ISRC_CURR_0P25U |
Adds 0.25 uA to the current source output |
BV_ISRC_CURR_0P5U |
Adds 0.5 uA to the current source output |
BV_ISRC_CURR_11P75U |
Adds 11.75 uA to the current source output |
BV_ISRC_CURR_1P0U |
Adds 1.0 uA to the current source output |
BV_ISRC_CURR_2P0U |
Adds 2.0 uA to the current source output |
BV_ISRC_CURR_4P5U |
Adds 4.5 uA to the current source output |
ISRC_TEMP_MEAS_RES_100_KOHM |
Temperature measurement: Run ISRC current through 100 kOhm resistor |
ISRC_TEMP_MEAS_RES_10_KOHM |
Temperature measurement: Run ISRC current through 10 kOhm resistor |
ISRC_TEMP_MEAS_RES_20_KOHM |
Temperature measurement: Run ISRC current through 20 kOhm resistor |
ISRC_TEMP_MEAS_RES_2_KOHM |
Temperature measurement: Run ISRC current through 2 kOhm resistor |
ISRC_TEMP_MEAS_RES_50_KOHM |
Temperature measurement: Run ISRC current through 50 kOhm resistor |
Global Variables¶
None.
Procedures¶
compaSelectGpioInput¶
Prototype: compaSelectGpioInput(auxio)
Selects a GPIO pin as input for COMPA.
The same pin is used as output for ISRC.
Parameter value(s)¶
- auxio : External input selection (index of AUX I/O pin)
isrcClamp¶
Prototype: isrcClamp(auxio)
Clamps the ISRC output to ground.
Parameter value(s)¶
- auxio : The pin to be clamped (index of AUX I/O pin)
isrcDisableTempMeasWithAdc¶
Prototype: isrcDisableTempMeasWithAdc()
Disables temperature measurement using the temperature dependent output of ISRC.
This procedure must only be called when ISRC is enabled.
isrcEnable¶
Prototype: isrcEnable(current)
Enables the ISRC peripheral with configuration of the output current.
This procedure must only be called when the ISRC is disabled. To change the ISRC current, call irscDisable()
before re-enabling the ISRC.
Parameter value(s)¶
- current : Current control bits (logical OR of BV_ISRC_CURR_XYZ)
isrcEnableTempMeasWithAdc¶
Prototype: isrcEnableTempMeasWithAdc(resistor)
Enables temperature measurement using the temperature dependent output of ISRC. The ISRC output (and COMPA input) node is connected internally to a 2-100 kOhm resistor to ground and to the ADC input.
Do not call isrcClamp()
, isrcRelease()
, compaSelectGpioInput()
, adcSelectIntInput()
or adcSelectGpioInput()
while temperature measurement is enabled.
This procedure must only be called when ISRC is enabled. Call isrcDisableTempMeasWithAdc()
before disabling ISRC.
Parameter value(s)¶
- resistor : Internal resistor to run the ISRC current through (ISRC_TEMP_MEAS_RES_XYZ)