Debugging¶
This chapter covers important debugging features such as breakpoints, memory optimization and how to utilize the debug interface with the CCS IDE.
External Resources¶
- Debugging common application problems with TI-RTOS video presentation on training.ti.com.
Debug Interfaces¶
The CC26X2R1 platform supports both the cJTAG and JTAG debug interfaces. Debug probes that support cJTAG, like the TI XDS110 and XDS100v3, can work natively with the CC26X2R1. Other Debug probes that only support JTAG, like the IAR I-Jet and Segger J-Link, need to inject a cJTAG sequence to enable JTAG functionality. The hardware resources included on the devices for debugging are listed as follows. Not all debugging functionality is available in all combinations of debug probe and IDE.
- Flash Patch and Breakpoint Unit (FPB) - 6 instruction comparators, 2 literal comparators
- Data Watchpoint and Trace Unit (DWT) - 5 watchpoints on memory access
- Instrumentation Trace Macrocell (ITM) - 32 x 32 bit stimulus registers
- Trace Port Interface Unit (TPIU) - serialization and time-stamping of DWT and ITM events
XDS110 Debug Probe¶
The LAUNCHXL-CC26X2R1 LaunchPads have an on-board XDS110, and this is the assumed debug probe for most development.
The XDS110 is the latest entry level debug probe (emulators) for TI embedded processors. Designed to be a complete solution that delivers JTAG and SWD connectivity at a low cost, the XDS110 is the debug probe of choice for entry-level debugging of TI microcontrollers, processors and SimpleLink devices. Also, both Core Processor and System Trace are available for all ARM and DSP devices that support Embedded Trace Buffer (ETB).
Configuring Debugger in CCS¶
If only one debug probe is attached, CCS will automatically select the
connected debug probe when a debug session is started. You can start a debug
session by clicking the debug icon on the toolbar.
If more than one debug probe is attached when a debug session is started, CCS will prompt you to select a debug probe as shown below. CCS will save the selected debug probe in the target configuration for the project.
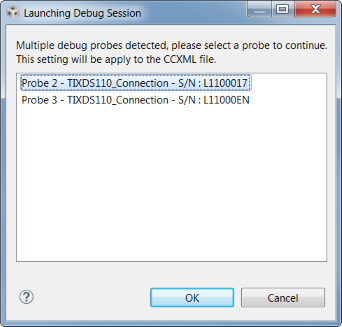
To set or change the selected debug probe for a project, it is necessary to set the serial number for the probe in the project’s target configuration.
Find The Serial Number¶
To find the serial number for XDS110 debug probe, open a command prompt and run
the command
c:\ti\ccs_7_3_0_00019\ccsv7\ccs_base\common\uscif\xds110\xdsdfu.exe -e
.
This will enumerate all the attached XDS110 debug probes. This should result in
output like the following.
C:\>c:\ti\ccs_7_3_0_00019\ccsv7\ccs_base\common\uscif\xds110\xdsdfu.exe -e
USB Device Firmware Upgrade Utility
Copyright (c) 2008-2015 Texas Instruments Incorporated. All rights reserved.
Scanning USB buses for supported XDS110 devices...
<<<< Device 0 >>>>
VID: 0x0451 PID: 0xbef3
Device Name: XDS110 Embed with CMSIS-DAP
Version: 2.3.0.9
Manufacturer: Texas Instruments
Serial Num: L1100017
Mode: Runtime
<<<< Device 1 >>>>
VID: 0x0451 PID: 0xbef3
Device Name: XDS110 Embed with CMSIS-DAP
Version: 2.3.0.9
Manufacturer: Texas Instruments
Serial Num: L11000EN
Mode: Runtime
Found 2 devices.
C:\>
For XDS100 series debug probes, open a command prompt and run the command
c:\ti\ccs_7_3_0_00019\ccsv7\ccs_base\common\uscif\xds100serial.exe
. This
should result in output like the following.
C:\>c:\ti\ccs_7_3_0_00019\ccsv7\ccs_base\common\uscif\xds100serial.exe
Scanning for XDS100 emulators...
VID/PID Type Serial # Description
0403/a6d1 XDS100v3 06EB12213144 Texas Instruments XDS100v3
C:\>
Configure Serial Number¶
It may be necessary to set or unset the selected debug probe. Use the following steps to do this.
|
![]() |
|
|
|
![]() |
|
![]() |
Breakpoints¶
Comparators in the FPB of the CC26X2R1 are used to break on an instruction fetch. This can be used to patch a function as it is fetched from instruction memory. Or these comparators can be used to supply a Breakpoint (BKPT) instruction to the CPU. These instructions halt the processors operation, waiting for the debug probe.
Considerations¶
While breakpoints are a useful tool for debugging code online, they have the possibility of altering the execution flow of a piece of code.
Breakpoints and Timing¶
Synchronous RF protocols are timing sensitive. Breakpoints can easily halt the execution long enough to lose network timing and break the link.
To still be able to debug, place breakpoints as close as possible to where the relevant debug information can be read or step through the relevant code segment to debug.
After you hit a breakpoint and read out the necessary debug information, it is recommended that you reset the device and re-establish the connection.
Breakpoints and Optimization¶
When compiler optimizations are enabled, toggling a breakpoint on a line of C code may not result in the expected behavior. Some examples include the following.
- Code is removed or not compiled in
- Toggling a breakpoint in the IDE results in a breakpoint somewhere other than the intended line. Some IDEs disable breakpoints on nonexistent code.
- Code block is part of a common subexpression
- A breakpoint might be trigged from a function or piece of code near the marked line. This might have been due to the compiler reusing sections.
- An if clause is represented by a conditional branch in assembly
- A breakpoint inside an if clause always breaks on the conditional statement, even when the condition is not true.
TI recommends selecting an optimization level as low as possible when debugging. See Optimizations for information on modifying optimization levels.
Breakpoints in CCS¶
Note
CCS reserves one hardware breakpoint for instruction stepping.
To toggle a breakpoint, do any of the following.
- Double-click the area to the left of the line number.
- Press
Ctrl
+Shift
+B
. - Right-click on the line.
A breakpoint set on line 207 looks like the following.
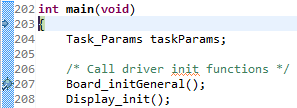
Figure 75. Breakpoint on line 207. Debugger halted at start of main().
For an overview of the active and inactive breakpoints, click on View
→ Breakpoints
.

Figure 76. List of breakpoints. Right-click to edit options, or de-select to deactivate.
To set a conditional break, do as follows.
- Right-click the breakpoint in the overview.
- Choose Properties.
When debugging, Skip Count and Condition can help skip a number of breaks or only break if a variable is a certain value.
Note
Conditional breaks require a debugger response and may halt the processor long enough to break an active RF connection, or otherwise disrupt timing on the debug target.
Watching Variables and Registers¶
Debuggers offer several ways of viewing the state of a halted program. Global variables are statically placed during link-time and can end up anywhere in the RAM or Flash of the chip. These variables can be viewed when then target is halted by the debugger through the Watch and Expression windows.
Unless removed due to optimizations, global variables are always available in these views. Local variables or variables that are only valid inside a limited scope are only viewable in that scope. Such variables can also be viewed with the Watch or Expression views, and may also be automatically displayed when breaking or stepping through code.
Considerations¶
Local variables are often placed in CPU registers and not on the stack. These variables also have a limited lifetime even within the scope in which they are valid. Depending on the optimization performed, a variable placed in a register may not have a cohesive view of the current state of the variable. Some possible solutions are:
- Move the variable to global scope, so it remains accessible in RAM.
- Make the variable volatile, so the compiler doesn’t place the value in a register.
- Make a shadow copy of the variable that is global and volatile.
Variables in CCS¶
You can view Global Variables by doing either of the following.
- Select
View
→Expressions
. - Select a variable name in code.
- Right-click and select Add Watch Expression.
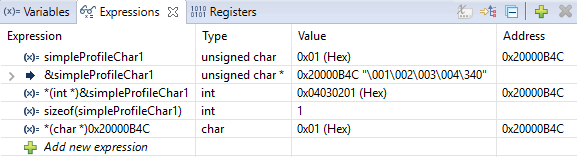
Figure 77. Variable watch window. Note that you can cast values, get address and sizeof, etc.
- Select
View
→Variables
toauto-variables
that are present at the current location when stepping through code.
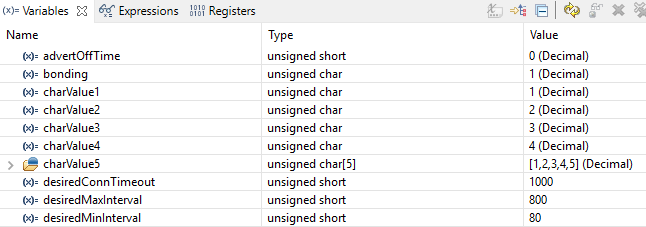
Figure 78. Local variables. This screenshot is taken during execution of the Simple Peripheral init function.
Memory Watchpoints¶
The DWT module contains four memory watchpoints that allow breakpoints on memory access. The hardware match functionality looks only at the address. If intended for use on a variable, the variable must be statically allocated.
Note
If a data watchpoint with value match is used, two of the four watchpoints are used.
Watchpoints in CCS¶
- Right-click on a global variable.
- Select
Breakpoint
→Hardware Watchpoint
. - Go to the list of breakpoints (
View
→Breakpoints
). - Right-click and edit the Breakpoint Properties to configure the watchpoint.
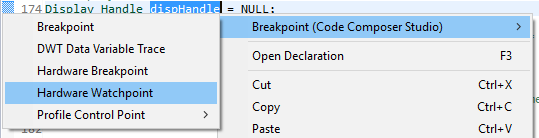
Figure 79. Adding a watchpoint on a variable.
This example configuration ensures that if 0x42 is written to the memory location for Characteristic 1 in the Bluetooth low energy simple_peripheral example project the device halts execution.
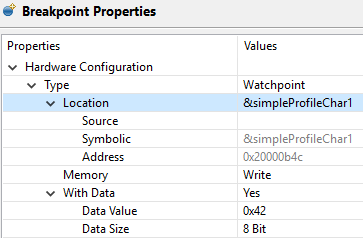
Figure 80. Configuring a hardware watchpoint to break on 8-bit write with value 0x42.
TI-RTOS Object Viewer¶
Debuggers may include the RTOS Object Viewer (ROV) plug-in that provides insight into the current state of TI-RTOS, including task states, stacks, and so forth.
This section discusses some ROV views useful for debugging and profiling. More details can be found in the TI-RTOS User’s Guide, including documentation on how to add log events to application code.
Scanning the BIOS for Errors¶
The BIOS Scan for errors
view goes through the available ROV modules and
reports on errors. This functionality can be a good point to start if anything
has gone wrong. This scan only shows errors related to TI-RTOS modules and only
the errors it can catch.
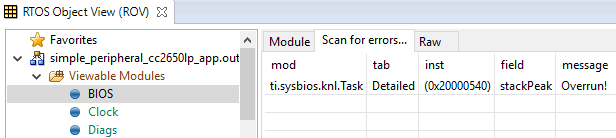
Figure 81. Scan for errors. Here a Task Stack has been overrun.
Viewing the State of Each Task¶
The Task Detailed
view is useful for seeing the state of each task and its
related runtime stack usage. This example shows the state the first time the
user-thread is called. Figure 82. shows the Bluetooth low
energy stack task, represented by its ICall proxy, the Idle task, the
simple_peripheral task and the GAPRole task.

Figure 82. Detailed view of the Tasks. Notice the address of the overrun task matches the instance id from Scan for errors.
The following list explains the column in Figure 82.
- address
- This column shows the memory location of the
Task_Struct
instance for each task. - priority
- This column shows the TI-RTOS priority for the task.
- mode
- This column shows the current state of the task.
- fxn
- This column shows the name of the entry function of the task.
- arg0, arg1
- These columns show arbitrary values that can be given to entry function of the task. In the image, the ICall_taskEntry is given 0xb001, which is the flash location of the entry function of the RF stack image and 0x20003a30 (the location of bleUserCfg_t user0Cfg, defined in main()).
- stackPeak
- This column shows the maximum run-time stack memory used based on watermark in RAM, where the stacks are prefilled with 0xBE and there is a sentinel word at the end of the run-time stack.
Note
Function calls may push the stack pointer out of the run-time stack, but not actually write to the entire area. A stack peak near stackSize but not exceeding it may indicate stack overflow.
- stackSize
- This column shows the size of the runtime stack, configured when instantiating a task.
- stackBase
- This column shows the logical top of the runtime stack of the task (usage starts at stackBase + stackSize and grows down to this address).
Viewing the System Stack¶
The Hwi Module
view allows profiling of the system stack used during boot
or for main(), Hwi execution, and Swi execution.

Figure 83. Viewing the System Stack in Hwi
The hwiStackPeak, hwiStackSize, and hwiStackBase can be used to check for system stack overflow.
ROV in CCS¶
To access the ROV while in a debug session in CCS:
- Click the Tools menu.
- Click RTOS Object View (ROV).
Warning
When using autosized heap, the ROV may display errors when accessing modules such as Task and Heap for ROV Classic. In order to use ROV with an autosized heap you can use ROV2, or do the following:
- Apply the following patch to the
function init()
in\kernel\tirtos\packages\ti\sysbios\heaps\package.xs
. The lines highlited below should be added.
function init()
{
/* Add HeapMem's primaryHeap addresses range to ROV's memory sections */
if (xdc.om.$name == "rov") {
var HeapMem = xdc.module('ti.sysbios.heaps.HeapMem');
// if (xdc.om['ti.sysbios.heaps'].HeapMem.$used) {
var Program = xdc.useModule('xdc.rov.Program');
var HeapMemCfg = Program.getModuleConfig(HeapMem.$name);
if (HeapMemCfg.primaryHeapBaseAddr != null) {
var base = Program.getSymbolValue(HeapMemCfg.primaryHeapBaseAddr.substr(1));
var end = Program.getSymbolValue(HeapMemCfg.primaryHeapEndAddr.substr(1));
/* Retrieve the MemoryImage java object. */
var Model = xdc.module("xdc.rov.Model");
var memReader = Model.getMemoryImageInst();
/* retrieve the sections list and add a new section */
var sections = memReader.getSections();
sections.addSection(base, end-base);
}
// }
/* Retrieve the MemoryImage java object. */
var Model = xdc.module("xdc.rov.Model");
var memReader = Model.getMemoryImageInst();
/* retrieve the sections list and add a new section */
var sections = memReader.getSections();
sections.addSection(0x20000000, 0x20005000);
}
}
Using the Memory Browser¶
Debuggers are able to show a representation of the memory on the CC26X2R1. In CCS, you can index by address or by symbol name. As an example, consider the stack that was overrun in Figure 82.:
Simple Peripheral Task’s stack. Note BE watermark |
GAPRole Task’s stack. Note it’s completely filled. |
![]() |
![]() |
The solution in this case would be to increase the stack size for the failing
task and see what the stack peak really is. The stackPeak
reported is
relying on how many watermark bytes are overwritten, so it can’t know how much
the overrun amounts to.
Because stacks are utilized from higher addresses towards lower addressed (upwards in the picture), stacks that overrun will tend to overwrite data at locations immediately before the stack.
Optimizations¶
Compiler optimizations are great for saving space or speeding up execution. However, these optimizations can be very difficult to debug around. There are multiple levels at which optimization can be turned on or off.
Project-wide optimization settings are the most general. Sometimes, given the constraints of the device, it is impossible to lower the size optimization level. File-wide optimization settings can be used like project-wide optimizations to turn on or off certain settings. The most granular control is using compiler directives to control optimization at a function level.
Optimizations in CCS¶
Project-Wide Optimizations¶
Open the project optimization settings by going to Project Properties
→ CCS Build
→ ARM Compiler
→ Optimization
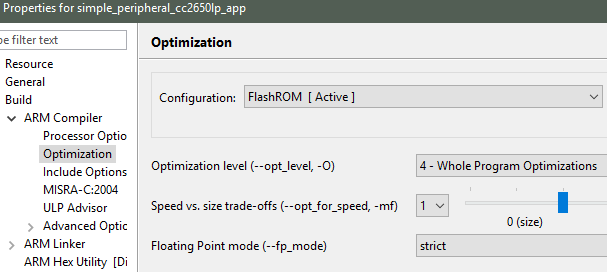
Figure 84. Project-level optimization setting in CCS
Single-File Optimizations¶
Note
Do single-file optimizations with care because this also overrides the project-wide preprocessor symbols.
- Right-click on the file in the Workspace pane.
- Choose Properties.
- Change the optimization level of the file using the same menu in the CCS project-wide optimization menu.
Single-Function Optimizations¶
Warning
Pragmas are very specific to the toolchain, and may lead to non-reusable code. Be careful where you use these.
#pragma FUNCTION_OPTIONS(myFunction, "--opt_level=0")
static void myFunction(int number)
{
// ...
return yourFunction(other_number);
}
#pragma GCC push_options
#pragma GCC optimize ("O0")
static void myFunction(int number)
{
// ...
return yourFunction(other_number);
}
#pragma GCC pop_options
Loading TI-RTOS in ROM Symbols¶
Some of the TI-RTOS kernel modules are included in ROM, and executed from ROM in order to save Flash space for the application. This can lead to some confusion, when only addresses are shown in the disassembly view and the call-stack view.
All TI-RTOS kernel code in ROM starts with address 0x1001xxxx
. In order to
make sense of the ROM’ed code, you need to include the symbol files in your
debug session.
Import in CCS¶
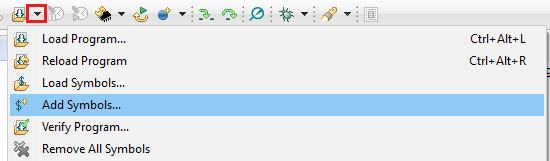
Figure 85. Adding symbol information for the BIOS kernel in ROM, via Add Symbols.
- While in debug mode, click the drop-down button next to the Load Program icon
- Select
Add Symbols ...
- Select
Browse ...
and find<SDK_INSTALL_DIR>\kernel\tirtos\packages\ti\sysbios\rom\cortexm\cc26xx\r2\golden\CC26xx\rtos_rom.xem3
Deciphering CPU Exceptions¶
Several possible exception causes exist. If an exception is caught, an exception handler function can be called. Depending on the project settings, this handler may be a default handler in ROM, which is just an infinite loop or a custom function called from this default handler instead of a loop.
When an exception occurs, the exception may be caught and halted in debug mode immediately, depending on the debugger. If the execution halted manually later through the Break debugger, it is then stopped within the exception handler loop.
Exception Cause¶
With the default setup using TI-RTOS, the exception cause can be found in the
System Control Space register group (CPU_SCS
) in the register CFSR
(Configurable Fault Status Register). The ARM Cortex-M3 User Guide describes
this register. Most exception causes fall into the following three categories.
- Stack overflow or corruption leads to arbitrary code execution.
- Almost any exception is possible.
- A NULL pointer has been dereferenced and written to.
- Typically (IM)PRECISERR exceptions
- A peripheral module (like UART, Timer, and so forth) is accessed
without being powered.
- Typically (IM)PRECISERR exceptions
The CFSR
register is available in View
→ Registers
.
When an access violation occurs, the exception type is IMPRECISERR because writes to flash and peripheral memory regions are mostly buffered writes.
If the CFSR:BFARVALID
flag is set when the exception occurs (typical for
PRECISERR), the BFAR
register in CPU_SCS
can be read out to find which
memory address caused the exception.
If the exception is IMPRECISERR, PRECISERR can be forced by manually disabling
buffered writes. Set [CPU_SCS:ACTRL:DISDEFWBUF
] to 1, by either manually
setting the bit in the register view in the debugger or by including
<hw_cpu_scs.h>
from Driverlib and calling the following.
#include <ti/devices/cc26x0r2/inc/hw_cpu_scs.h>
//..
int main()
{
// Disable write-buffering. Note that this negatively affect performance.
HWREG(CPU_SCS_BASE + CPU_SCS_O_ACTLR) = CPU_SCS_ACTLR_DISDEFWBUF;
// ..
}
Using TI-RTOS and ROV to Parse Exceptions¶
To enable exception decoding in the RTOS Object View (ROV) without using too much memory, use the Minimal exception handler in TI-RTOS. The default choice in the Z-Stack projects is to use no exception handler.
To set this up, change the section of the TI-RTOS configuration file that relates to M3Hwi so that it looks like the code below:
//m3Hwi.enableException = true;
m3Hwi.enableException = false;
//m3Hwi.excHandlerFunc = null;
m3Hwi.excHookFunc = "&execHandlerHook";
Then, make a function somewhere with the signature void
(*Hwi_ExceptionHookFuncPtr)(Hwi_ExcContext*);
such as the one below:
#include <ti/sysbios/family/arm/m3/Hwi.h>
// ...
volatile uintptr_t *excPC = 0;
volatile uintptr_t *excCaller = 0;
// ...
void execHandlerHook(Hwi_ExcContext *ctx)
{
excPC = ctx->pc; // Program counter where exception occurred
excCaller = ctx->lr; // Link Register when exception occurred
while(2);
}
Setting m3Hwi.enableException
to false enables the minimal handler, which
fills out the global Hwi_ExcContext
structure that the ROV looks at to show
the decoded exception. By setting up an excHookFunc, the minimal exception
handler will call this function and pass along a pointer to the exception
context for the user to work with. This structure is defined in
<ti/sysbios/family/arm/m3/Hwi.h>
.
When an exception occurs, the device should end up in that infinite loop.
Inspect the ROV
→ Hwi
→ Exception information
.
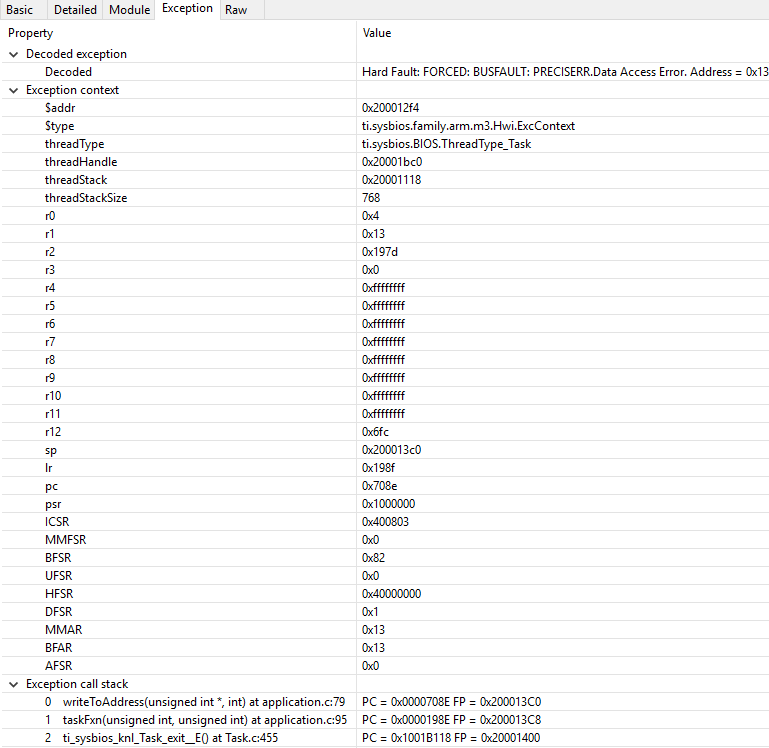
Figure 86. Decoded exception, intentional write to address 0x0013 which is illegal. Note that writebuffering has been disabled to get a precise error location, and that m3Hwi.enableException has been set to false to get the decoding.
In this case, a bus fault was forced in the function writeToAddress by dereferencing address 0x0013 and trying to write to it:
void writeToAddress(uintptr_t *addr, int val)
{
*(int *)addr = val;
}
// ..
void taskFxn(...)
{
// ..
writeToAddress( (void*)19, 4 ); // Randomly chosen values
}
The write instruction was placed on line 79 of application.c, as indicated. To get a precise location, the write buffer was disabled as described earlier.
It can be instructive to look at the disassembly view for the locations specified by PC (program counter) and LR (link register). PC is the presumed exception location, and LR is normally the location the failing function should have returned to. As an example, the PC at this exception:
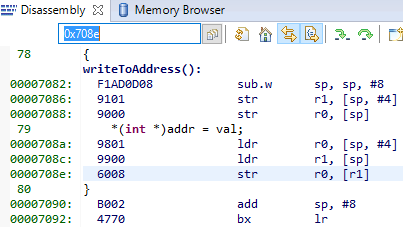
Figure 87. Here the pc
from the decoded exception was looked up in the disassembly
view.
Some forensics is required here. We have from the Hwi decoding in ROV (and from
the exception context in the exception hook) that the program counter was
0x708e
when the exception occurred.
At that location there is a store instruction str r0, [r1]
meaning, store
in R0 the value of what the memory address in R1 points to. The business with
SP
in the figure above is related to optimization being turned off, so all
local variables are stored on the stack, even though in this case R0 and R1
could have been used directly from the caller.
Now we know that the exception occurred because someone called
writeToAddress
with an invalid address.
Thanks to the exception decoder we can easily find the call site by looking at
the call stack, but if the call stack isn’t helpful, we can look at lr
,
which is seen in the exception decoder to be 0x198f
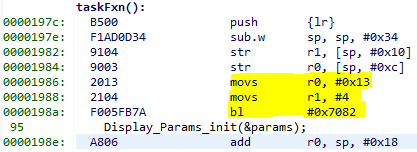
Figure 88. Call site as specified in lr
. Note that lr is the instruction after the
call to writeToAddress
because execution would have resumed here.
We can see here that R0 and R1 are initialized with constants. This means that some programmer has intentionally called the write function with an address that causes a busfault.
Most often the reason for a bus-fault is that a pointer is not initialized and
a function like writeToAddress
gets the pointer, assumes it’s valid and
dereferences the pointer and writes to the invalid address.
Application exits prematurely¶
When an application is built with TI-RTOS kernel instrumentation enabled, it is
possible that one of its runtime checks might cause the application to terminate
prematurely. Typically when this occurs, the application will halt at a
breakpoint symbol called loader_exit
(in CCS) or __exit
(in IAR).
Various available runtime checks are enabled via the TI-RTOS .cfg
configuration file. To determine what checks are enabled, you can see the
documentation included in the .cfg
file or refer to the TI-RTOS kernal
User Guide directly.
Some applications may contain a pair of Debug and Release build configurations. When such build configurations exist, the Debug configuration takes advantage of utilizing a suite of runtime checks. These runtime check can include but are not limited to:
- Task stack overrun checks
- System stack overrun checks
- Various asserts (Tasks, Swi, Hwi, etc...)
- Loggers (UIA loggers)
- Kernel policy checks (Checking if runtime creation/deletions are permitted)
When an runtime check detects an anomaly, the kernel responds by capturing and
printing the fault condition and halting the system at the exit
or loader_exit
symbol.
loader_exit
in CCS¶
If a fault condition causes an application to exit prematurely in CCS, the
target will halt at the loader_exit
symbol - as shown
in Figure 89.. The cause for the fault condition is typically
displayed in the console window.
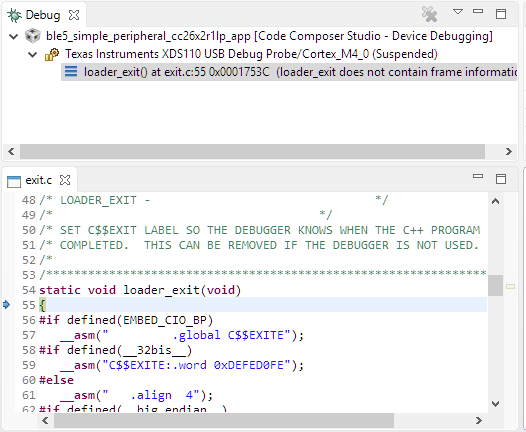
Figure 89. A sample view when a CCS application terminates.
If the console window is not already open, CCS will open the console window for you and print the cause for the termination. Alternatively, you can also use ROV to determine the cause for the fault.
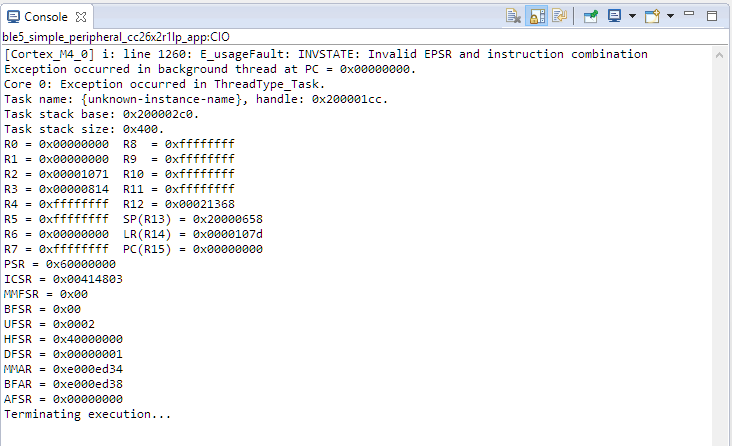
Figure 90. Sample output of an exception onto the CCS console.
Memory Management¶
The flash is split into erasable pages of 8kB. The various sections of flash and their associate linker files are as follows.
- Simple NV (SNV) Area: used for nonvolatile memory storage by the GAP Bond Manager (for BLE applications) and also available for use by the application.
- Customer Configuration Area (CCA): the last sector of flash used to store customer-specific chip configuration (CCFG) parameters. The unused space of the CCA sector is allocated to the application project.
Customer Configuration Area¶
The Customer Configuration Area (CCA) occupies the last page of flash and lets a customer configure various chip and system parameters in the Customer Configuration (CCFG) table . The CCFG table is defined in ccfg_app_ble.c, which can be found in the Startup folder of the application project. The last 88 (sizeof(ccfg_t)) bytes of the CCA sector are reserved by the system for the CCFG table. By default, the linker allocates the unused flash of the CCA sector to the application image for code and data use. The linker can be modified to reserve the entire sector for customer parameter data (for example, board serial number and other identity parameters).
The CCA region is defined linker file of the application by the FLASH_LAST_PAGE symbol. Placement is based on the IDE:
For CCS:
MEMORY { ... // CCFG Page, contains .ccfg code section and some application code. FLASH_LAST_PAGE (RX) : origin = FLASH_LAST_PAGE_START, length = FLASH_PAGE_LEN ... } SECTIONS { ... .ccfg : > FLASH_LAST_PAGE (HIGH) ... }
See the CC26x2 Technical Reference Manual for details on CCFG fields and related configuration options, including how to set the CCFG to disable access to internal flash memory contents.
RAM¶
Similar to flash, the RAM is shared between the application and stack projects. The RAM sections are configured in their respective linker files.
- Application Image: RAM space for the application and shared heaps. This image is configured in the linker configuration file of the application: cc26xx_app.icf (IAR) and cc26xx_app.cmd (CCS).
- Stack Image: RAM space for the .bss and .data sections of the stack. This image is configured in the linker configuration file of the stack: cc26xx_stack.icf (IAR) and cc26xx_stack.cmd (CCS).
System Stack¶
Besides the RTOS and ICall heaps, consider other sections of memory. As described in Tasks, each task has its own runtime stack for context switching. Another runtime stack is used by the RTOS for main(), HWIs, and SWIs. This system stack is allocated in the application linker file to be placed at the end of the RAM of the application.
For CCS, the RTOS system stack is defined by the Program.stack parameter in the appBLE.cfg RTOS configuration file:
/* main() and Hwi, Swi stack size */
Program.stack = 1024;
and placed by the linker in the RAM space of the application:
/* Create global constant that points to top of stack */
/* CCS: Change stack size under Project Properties */
__STACK_TOP = __stack + __STACK_SIZE;
Dynamic Memory Allocation¶
The system uses two heaps for dynamic memory allocation. The application designer must understand the use of each heap to maximize the use of available memory.
The RTOS is configured with a small heap in the app_ble.cfg RTOS configuration file:
var HeapMem = xdc.useModule('xdc.runtime.HeapMem');
BIOS.heapSize = 1668;
This heap (HeapMem) is used to initialize RTOS objects and allocate the task runtime stack of the protocol stack. TI chose this size of this heap to meet the system initialization requirements. Due to the small size of this heap, TI does not recommend allocating memory from the RTOS heap for general application use. For more information on the TI-RTOS heap configuration, see the Heap Implementations section of the TI-RTOS SYS/BIOS Kernel User’s Guide.
The application must use a separate heap. The ICall module uses an area of application RAM which can be used by the various tasks. The size of this ICall heap is defined by the HEAPMGR_SIZE preprocessor definition in the application project. Using a non-zero value sets the ICall heap to the specified value, while a HEAPMGR_SIZE value of zero (0) auto sizes the heap to a size equal to the amount of available free RAM not allocated by the linker. By default, the simple_peripheral project uses the auto size feature. Although the ICall heap is defined in the application project, this heap is also shared with the Bluetooth low energy protocol stack. APIs that allocate memory (such as GATT_bm_alloc()) allocate memory from the ICall heap.
To profile the amount of ICall heap used, define the HEAPMGR_METRICS preprocessor symbol in the application project.
Note
The auto heap size feature does not determine the amount of heap needed for the application. The system designer must ensure that the heap has the required space to meet the application’s runtime memory requirements.
The following is an example of dynamically allocating a variable length (n) array using the ICall heap:
//define pointer
uint8_t *pArray;
// Create dynamic pointer to array.
if (pArray = (uint8_t*)ICall_malloc(n*sizeof(uint8_t)))
{
//fill up array
}
else
{
//not able to allocate
}
The following is an example of freeing the previous array:
ICall_free(pMsg->payload);
Debugging RF Output¶
As mentioned in the CC26x2 Technical Reference Manual, the RF output can be mapped to pins on the LaunchPad for RF signal debugging. These pins are intended to be used when connecting an RF range extender. However, they can also help in instances where it is unclear if the device is transmitting or receiving in the right window.
To enable RF output debugging, you will need to map the RF Core LNA enable and PA enable output signals to a GPIO. See Enable RF Observables for an example of using DIO6 to output the LNA enable signal (logic high corresponds to an Rx) and DIO7 to output the PA signal (logic high corresponds to a Tx). These pins correspond to the Red (DIO6) and Green (DIO7) CC26x2R1 LaunchPad™ LEDs and if the jumpers are connected, these LEDs will flicker to indicate RF activity. If these pins are unavailable in your project, you can select any available DIO.
/******************************************************************************* * INCLUDES */ #include <driverlib/ioc.h> ... int main() { //Add the following after Board_initGeneral(); // Map RFC_GPO0 to DIO6 IOCPortConfigureSet(IOID_6, IOC_PORT_RFC_GPO0, IOC_IOMODE_NORMAL); // Map RFC_GPO1 to DIO7 IOCPortConfigureSet(IOID_7, IOC_PORT_RFC_GPO1, IOC_IOMODE_NORMAL); ... }
For more details, see the “External Signaling” chapter of the CC26x2 Technical Reference Manual.