Application Layer¶
Since the micro stack can support multiple functionalities (broadcaster, observer, connection monitor), it is up to the application layer to define the behavior of the system. The following sections will discuss the TI provided implementations of the broadcaster and monitor roles.
micro_ble_cm.c
: Connection monitor applicationmicro_eddystone_beacon.c
: Broadcaster application implementing the Eddystone protocol
Connection Monitor (CM) Application¶
The connection monitor application is built on top of the uGAP layer operating in monitor mode and is responsible for implementing the high level connection tracking feature. This includes:
- Initializing connection parameters for a connection to monitor
- Performing the initial scan to find a connection event
- Scheduling subsequent scans to continue following the connection
The following sections will describe the above list in detail.
Initializing CM Session¶
In order to follow a connection, the CM needs to know the connection parameters that were exchanged during the connection process between master and slave. These include:
- access address
- connection interval
- hop value
- next channel
- channel map
- CRC initialization value
These parameters can be obtained from a BLE(5)-Stack application by calling
the HCI_EXT_GetActiveConnInfoCmd
command. Once they are obtained,
they should be shared (via an out of band mechanism such as UART, LIN, CAN etc)
with the CM device. The CM device then can use ubCM_startExt()
to start the
initial scan.
Starting a Monitor Session¶
In order to start tracking a connection the connection monitor needs to perform an initial wide scan in order to catch a connection event. This scan should be at worst case the connection interval times the number of active channels in order to ensure the connection can be detected.
The logic trace below was generated by enabling the RF observable pins on master and slave device in the connection (RX, TX) as well as the connection monitor (RX). It shows the initial scan.

Following a connection¶
Once a packet is received during the initial scan the CM will setup smaller scans based on the next expected event. Since it is now following the connection, it can calculate the channel and instant (adjusted for master and slave sleep clock accuracy) to listen for the next event.
The core of connection following is based on
monitor_indicationCB
: Invoked when a packet is receivedmonitor_completeCB
: Invoked when a scan window has completed.The logic trace below shows the CM actively tracking a connection

Micro Eddystone Beacon App¶
Note
The Micro Eddyston Beacon app is not available in all SDKs such as CC26x2/CC13x2. It can be found in the CC2640R2 SDK.
The Micro Eddystone Beacon app implements the Google Eddystone. Protocol. The details of the protocol are outside the scope of this document. Instead, this section will focus on the primary elements of the broadcaster role.
The key parts of the broadcaster role includes:
- Initializing the device address
- Starting and stopping advertising
- Processing the change of advertising data
- Consuming the uGAP broadcast callbacks
Device Address¶
The micro stack will use a the public address that is burned into the device.
A static address can be used if FEATURE_STATIC_ADDR
is defined.
The micro-stack will be initialized with the device address using
uble_stackInit()
.
Start/Stop Advertising¶
The application will control the start and stop of advertising based on button
press. Starting advertisement involves setting the TX power and calling
ugap_bcastStart()
Changing Advertising data¶
The advertisement data can be changed on the fly with a call to uble_setParameter
with the parameter ID UBLE_PARAM_ADVDATA
. The beacon will do so as specified
by the Eddystone protocol.
Processing Micro GAP Advertising Callbacks¶
There are three callbacks that are of interest to the broadcaster role
ugapBcastStateChangeCb_t
: The Micro GAP has changed statesugapBcastAdvPrepareCb_t
: The Micro GAP is preparing an advertisementugapBcastAdvDoneCb_t
: The Micro GAP has completed an advertisement
State change¶
The states of interest to the application are:
UGAP_BCAST_STATE_INITIALIZED
: The micro stack has been initialized and is ready for commandsUGAP_BCAST_STATE_IDLE
: The micro stack is idle and not performing any functionUGAP_BCAST_STATE_ADVERTISING
: The micro stack is actively advertisingUGAP_BCAST_STATE_WAITING
: The micro stack is idle during an off duty cycle period
Advertisement Prepared¶
This callback is invoked when the micro stack is setting up the next advertisement event. The micro Eddystone beacon uses this to switch the advertisement payload as needed by the Eddystone protocol.
Advertisement done¶
Can be used to manage the duty cycle of advertisement or count the number of completed advertisements.
Micro Stack Parameters¶
The micro stack has a small layer that is responsible for setting up the various
parameters used across the stack. This is encapsulated in uble.c
.
In addition to parameter management, the uble layer defines callbacks, a message format, and event proxy function that is used to build and post events from higher priority context (e.g. RF driver callback) to the micro stack. The uble layer is responsible for address management.
Important uble parameters are described below.
Priority¶
Micro BLE Stack has an overall stack priority that is applied to every issued radio command.
The stack priority is intended to give Micro BLE Stack a relative priority against the other
RF clients. The stack priority is saved in RFPriority through uble_setParameter()
.
UBLE_RF_PRI_NORMAL
, UBLE_RF_PRI_HIGH
, and UBLE_RF_PRI_HIGHEST
are
translated into RF_PriorityNormal
, RF_PriorityHigh
, and RF_PriorityHighest
respectively when the Micro BLE Stack calls RF_scheduleCmd()
.
If Micro BLE Stack’s priority is higher than another RF clients, every Micro BLE
Stack’s radio command gets privileged unless the priority of other RF client
command which has been scheduled is RF_PriorityHighest
.
Note that the priority of strictly time-critical and crucial commands, such as
CMD_PROP_RX_ADV
for beacon reception in TI-15.4-Stack, tends to be set to
RF_PriorityHighest regardless of the stack priority.
Time Critically¶
Time Criticality is how important a radio command being executed at an exact time is.
Time Criticality is saved in RFTimeCriticality through ub_uble_setParam().
For example, if advertising events absolutely cannot be missed, and it is okay
for the micro stack to preempt other RF activity, then
rfPriority
should be set to RF_TIME_CRITICAL
.
Otherwise, rfPriority
should be set to RF_TIME_RELAXED
.
Scheduling¶
When the Micro BLE Stack schedules a command, Priority and Time Criticality
are passed to RF_scheduleCmd()
. These parameters are contained in the
priority element of RF_ScheduleCmdParams and pastTrig
field in startTrigger
element of the RF driver command struct.
If rfPriority
is RF_TIME_CRITICAL
so the command has to start at a
designated time, a failure returned if the radio is unavailable to schedule
the command at the moment requested. RF_scheduleCmd()
rejects the command
if any portion of the desired time period reserved by the same- or
higher-priority operation of other RF clients or the RF driver command queue is
full.
If rfPriority
is RF_TIME_RELAXED
so the command can be delayed if the
radio is unavailable at moment requested; that command is scheduled with
pastTrig=1
. Once the command with pastTrig=1
is scheduled successfully,
it will start at the desired start time if the radio is available, or when the
radio is available after the start time.
The RF Event RF_EventRadioAvailable needs to be activated since it is used for rescheduling
Micro RF interface¶
The micro RF interface or urfi
is responsible for instantiating and
configuring various RF commands used by the micro stack.
The following RF commands are used by the micro stack
rfc_CMD_BLE_ADV_COMMON_t
: Advertiser command, used by broadcasterrfc_CMD_BLE_SCANNER_t
: Scanner command, used by observerrfc_CMD_BLE_GENERIC_RX_t
: Generic RX command, used by connection monitor
Micro Link Layer¶
The uLL is mainly responsible for maintaining the device state and scheduling, pre- and post-processing, the radio operations to send and/or receive ADV packets. The whitelist filter policy without privacy is supported.
Radio Initialization¶
The uLL has a direct interface to the RF Driver. It opens a connection to the RF
Driver as an independent client using RF_open()
with setup parameters for
BLE PHY. The setup parameters referenced to interface with the RF Driver are
defined in the Micro RF interface. These parameters are dependent on the features enabled
in Micro BLE Stack.
Note: Some Setup Parameters are defined by the application, ubParams, ubBDAddr, and rfTimeCrit.
Application Parameters¶
The uLL maintains parameters used for advertising. Most of the
parameters can be accessed by uGAP through uble_setParameter()
and
uble_setParameter()
functions. See local and global variables defined
uble.c
in the stack folder for Application parameters.
Micro Link Layer States¶
There are 4 states in the uLL: Standby, Advertising, Scanning, and Monitoring. The uLL changes the state from one to another at the uGAP’s request. Interfacing to the BLE Micro Stack should be done through uGAP APIs.
Standby State¶
This is the default state in the uLL. The uLL doesn’t send any packets in this state. The uLL may leave this state to enter the any of the other states.
Advertising State¶
In this state, the uLL sends advertising PDUs in advertising events.
Each advertising event is composed of one or more advertising PDUs sent on the channels specified in UB_PARAM_DFLT_ADVCHANMAP. The advertising event will be closed after one advertising PDU has been sent on each of the channels specified in UB_PARAM_DFLT_ADVCHANMAP or it can be closed earlier if requested by the uGAP.
The time between two consecutive advertising events is specified in
UBLE_PARAM_DFLT_ADVINTERVAL
. The actual interval will be
UBLE_PARAM_DFLT_ADVINTERVAL
+ AdvDelay, where AdvDelay is a pseudo-random
value ranging from 0 ms to 10 ms generated by the uLL for each advertising
event.
An advertising event is limited to the following type in this version of design:
- A non-connectable undirected event: ADV_NONCONN_IND PDU is used. No response PDU is expected.
Per each advertising event, the following notifications will be delivered to the uGAP before and after the event. Note that these notifications are conveyed based on the application task’s priority since they are following the paths illustrated in Figure 125.
UGB_EVT_ADV_PREPARE
: This notification event is generatedUBLE_PARAM_DFLT_TIMETOADV
ms prior to every advertising event. The purpose of this event is to let the application take time to update the advertising data with up-to-date information if necessary. IfUBLE_PARAM_DFLT_TIMETOADV
is 0, this notification event won’t happen.UGB_EVT_ADV_POSTPROCESS
: This notification event is generated at the completion of every advertising event.UGB_EVT_STATE_CHANGE
: This notification event is generated when state has changed
Monitoring State¶
In this state, the uLL will scan a particular channel with specified scan
parameters. An access address filter is applied based on the accessAddr
parameter. The uGAP will supply all the necessary parameters and initialize the
scan. Each scan has an associated monitorHandle
which
maintains the session. The scan will perform over the specified channels by
monitorChan
.
The monitor session currently scanning is determined by the
startTime
which accounts for when the next connection event of the
monitored connection should start. Furthermore the crcInit
of the connection
to follow must be input.
If a PDU is detected prior to the scan duration, monitorDuration
,
during an active scan, the uLL will call the callback registered for indications.
The application is then notified with a UGAP_MONITOR_EVT_MONITOR_INDICATION
event.
One the scan duration as elapsed, the application is notified with
UGAP_MONITOR_EVT_MONITOR_COMPLETE
.
If there are additional pending scans, the uGAP will begin the next channel scan.
If there is a state change, the application is notified with
UGAP_MONITOR_EVT_STATE_CHANGE
.
RF Callbacks¶
Each RF event is processed differently depending on the mode that the micro stack is operating in (broadcaster, observer, connection monitor). These RF events are consumed through callbacks that are plugged to the RF driver via the uLL. See the table below for a summary of how the RF events are processed by the uLL.
Mode | RF callback | Events Processed | Events generated |
Broadcaster | ull_advDoneCb | RF_EventLastCmdDone | ULL_EVT_ADV_TX_SUCCESS |
RF_EventCmdAborted, RF_EventCmdStopped, RF_EventCmdPreempted, RF_EventCmdCancelled | ULL_EVT_ADV_TX_FAILED | ||
Observer | ull_scanDoneCb | RF_EventRxEntryDone | ULL_EVT_SCAN_RX_SUCCESS |
RF_EventLastCmdDone, RF_EventInternalError | ULL_EVT_SCAN_RX_FAILED or ULL_EVT_SCAN_RX_BUF_FULL (depending on status from RF driver) | ||
Connection Monitor | ull_monitorDoneCb | RF_EventRxEntryDone | ULL_EVT_MONITOR_RX_SUCCESS |
RF_EventLastCmdDone | ULL_EVT_MONITOR_RX_WINDOW_COMPLETE or ULL_EVT_MONITOR_RX_BUF_FULLBLE_ERROR_RXBUF (depending on status from RF driver) |
||
RF_EventInternalError | ULL_EVT_MONITOR_RX_FAILED |
For more information regarding the RF driver see the RF Driver API Driver API Reference.
Micro GAP¶
The uGAP sits between the uLL and the application and is responsible for controlling the uLL to set up and run profile roles. The application can indirectly configure the uLL through the uGAP and be notified of events from the uLL through uGAP callbacks.
Parameters Management¶
The uGAP maintains the following parameters that control its behavior
ugbNumAdvEvent¶
The number of advertising events to be done before the Broadcaster stops its
job. This is given when the application starts the Broadcaster by calling
ug_bcastStart(). If this parameter is set to 0, the Broadcaster will not go to
UGAP_BCAST_STATE_INITIALIZED
state once started unless it is requested to
stop.
ugbDutyOnTime¶
Time period during which the Broadcaster stays in UGAP_BCAST_STATE_ADVERTISING
state. The uLL stays in Advertising State as well. When this time period ends,
the Broadcaster state will transition to UGAP_BCAST_STATE_WAITING
and the uLL
will exit Advertising State. This parameter is effective only if Broadcaster
Duty Control is enabled. If Broadcaster Duty Control is disabled, transition
to other state from UGAP_BCAST_STATE_ADVERTISING
is not affected by this parameter.
A 100-ms time unit is used.
ugbDutyOffTime¶
Time period during which the Broadcaster stays in UB_BCAST_STATE_WAITING state.
The uLL cannot be in Advertising State during this period. When this time period
ends, the Broadcaster state will transition to UGAP_BCAST_STATE_ADVERTISING
and
the uLL will enter Advertising State. This parameter is effective only if
Broadcast Duty Control is enabled. If 0, Broadcaster Duty Control is disabled
and the Broadcaster will not enter UGAP_BCAST_STATE_WAITING
state.
A 100-ms time unit is used.
Role Management¶
The uGAP is the main interface to operate in various roles.
There are two distinct roles the uGAP supports:
- Broadcaster
- Monitor
The application must configure the uGAP to operate in the mode desired. This section goes over specifics of the individual roles.
Broadcaster Role¶
If the application configures the uGAP to operate Broadcaster role, the uGAP lets the uLL send advertising events as described in Advertising State in accordance with the parameters listed in Application Parameters.
The Broadcaster Role has 4 states:
UG_BCAST_STATE_INITIALIZED
: Broadcaster is initialized but has never started. The corresponding state of the uLL can be anything butULL_STATE_ADVERTISING
.UGAP_BCAST_STATE_ADVERTISING
: Broadcaster is advertising in this state. The corresponding state of the uLL isULL_STATE_ADVERTISING
. If Broadcaster Duty Control is enabled, the duty timer starts with the duration of BcastDutyOnTime when this state is entered. Then, the state switches toUGAP_BCAST_STATE_WAITING
when the duty timer expires. If 0 was passed to NumAdvEvent when ug_bcastStart() is called, ugbNumAdvEvent won’t have any effect on this state. Otherwise, the state switches to UG_BCAST_STATE_IDLE if requested through ug_bcastStop() or the total number of Advertising Events since ug_bcastStart() was called reaches ugbNumAdvEvent. If ug_bcastSuspend() is called, the state switches toUGAP_BCAST_STATE_SUSPENDED
, putting the duty timer on hold if Duty Control is enabled. The duty timer will resume when the state switches back to this state.UGAP_BCAST_STATE_WAITING
: Broadcaster started but is not advertising in this state because it’s in DutyOffTime period. The corresponding state of the uLL is UL_STATE_STANDBY. If Broadcaster Duty Control is enabled, the duty timer starts with the duration of BcastDutyOffTime when this state is entered. Then, the state switches toUGAP_BCAST_STATE_ADVERTISING
when the duty timer expires. The state switches to UG_BCAST_STATE_IDLE if requested through ug_bcastStop(). If ug_bcastSuspend() is called, the state switches toUGAP_BCAST_STATE_SUSPENDED
, putting the duty timer on hold if Duty Control is enabled. The duty timer will resume when the state switches back to this state.UGAP_BCAST_STATE_SUSPENDED
: Broadcaster started but is not advertising in this state. The corresponding state of the uLL can be anything butULL_STATE_ADVERTISING
. The former state shall be recorded when this state is entered. If the suspension is lifted through ug_bcastResume(), the state will switch back to the former state. The state switches to UG_BCAST_STATE_IDLE if ug_bcastStop() is called.
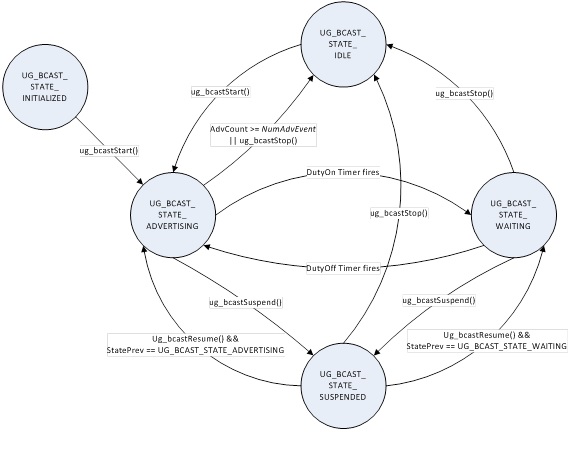
Figure 128. Broadcaster states
The BLE specification doesn’t allow Broadcaster to have Limited Discoverable Mode. However, the uGAP provides a duty control means similar to Limited Discoverable Mode to save power consumption. The duty control can be implemented with timers based on BcastDutyOnTime and BcastDutyOffTime explained in Parameters Management. Broadcaster’s Advertising State corresponds to the uLL’s Advertising State.
The typical life cycle of the Broadcasting function encompassing the application down to the uLL is illustrated in Figure 129.
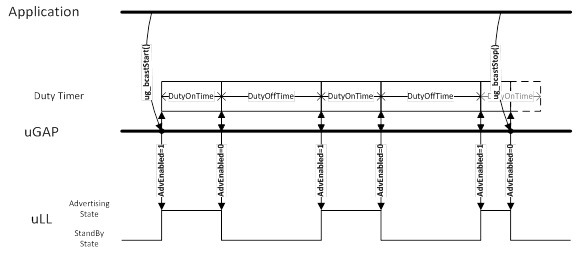
Figure 129. Life Cycle of Broadcaster Function
Monitor Role¶
The Monitor Role is not an official BLE Specification role. This section is to describe how the uGAP operates when using the Monitor Feature.
This role is tested in stand alone condition only. No other uBLE Stack feature should be used in conjunction.
Monitor role is designed to follow an active BLE connection if given connection information such as access address, hop increment, and connection interval. With this information the Monitor role sets up uGAP and uBLE.
The Monitor Role has 3 States:
UGAP_MONITOR_STATE_INITIALIZED
: The monitor is initialized but is not monitoring.UGAP_MONITOR_STATE_IDLE
: The monitor is not monitoring in this state. This corresponds to UL_STATE_STANDBY in the uLL.UGAP_MONITOR_STATE_MONITORING
: The monitor is scanning. This corresponds to UL_STATE_MONITORING in the uLL.
When a packet is detected with the during a scan with the Connection
Parameters passed in from the ‘uble.c’ source file a UGAP_MONITOR_EVT_MONITOR_INDICATION
event is generated.
When a scan is complete, a UGAP_MONITOR_EVT_MONITOR_COMPLETE
event is generated.
If there are pending scans, the uGAP will start the next scheduled scan.
Each time the Monitor switches states, a UGAP_MONITOR_EVT_STATE_CHANGE
event is generated.