Generic Access Profile (GAP)¶
The GAP layer of the Bluetooth Low Energy protocol stack is responsible for connection functionality. This layer handles the access modes and procedures of the device including device discovery, link establishment, link termination, initiation of security features, and device configuration. See GAP State Diagram. for more details.
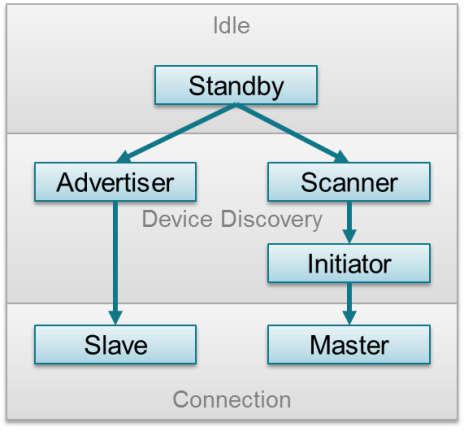
Figure 66. GAP State Diagram.
Based on the role for which the device is configured, GAP State Diagram. shows the states of the device. The following describes these states.
- Standby: The device is in the initial idle state upon reset.
- Advertiser: The device is advertising with specific data letting any initiating devices know that it is a connectable device (this advertisement contains the device address and can contain some additional data such as the device name).
- Scanner: When receiving the advertisement, the scanning device sends a scan request to the advertiser. The advertiser responds with a scan response. This process is called device discovery. The scanning device is aware of the advertising device and can initiate a connection with it.
- Initiator: When initiating, the initiator must specify a peer device address to which to connect. If an advertisement is received matching that address of the peer device, the initiating device then sends out a request to establish a connection (link) with the advertising device with the connection parameters described in GAP Connection State.
- Slave/Master: When a connection is formed, the device functions as a slave if it is the advertiser and a master if it is the initiator.
Bluetooth devices operate in one or more GAP roles based on the application use case. The GAP roles utilize one or more of the GAP states. Based on this configuration, many Bluetooth Low Energy protocol stack events are handled directly by the main application task and sometimes routed from the GAP Bond Manager. The application can register with the stack to be notified of certain events.
GAP Roles¶
Based on the configuration of the device, the GAP layer always operates in one of four (4) roles as defined by the specification:
- Broadcaster - The device is an advertiser that is non connectable.
- Observer - The device scans for advertisements but cannot initiate connections.
- Peripheral - The device is an advertiser that is connectable and operates as slave in a single link-layer connection.
- Central - The device scans for advertisements and initiates connections and operates as a master in a single or multiple link-layer connections.
The Bluetooth Core Specification Version 5.1 allows for certain combinations of multiple roles, which are supported by the Bluetooth Low Energy protocol stack. For configuration of the Bluetooth Low Energy stack features, see Developing a Custom Application
Note
Bluetooth 5 also introduces new features including Extended Advertising. Bluetooth 5 supports legacy advertising maintaining backwards compatibility with previous versions. The following sections will describe how to operate the device for various GAP roles along with how to use the new Extended Advertising features with the new APIs.
The following sections will describe how to use the LE Advertising Extension feature. As its name suggests, the amount of advertising data that can be sent over the air has been extended from 31 bytes to 1650 bytes. Also multiple advertising sets can be created using a mix of legacy and extended advertisements. Extended advertisements introduces use the secondary advertising channels which uses the data channels to send extensive data in addition to the primary advertising channels (Ch. 37, 38, and 39) used in legacy advertisement.
GAP Constraints¶
- Periodic Advertisement feature is not supported in this release.
- GAP Scanner can only scan one PHY per scanning cycle.
- The data length of the advertising data and the scan response data for Extended Advertising is limited to 1650 bytes. The connectable undirected advertising data is limited to 212 bytes. The connectable directed advertising data is limited to 206 bytes. For more information, refer to the Host Advertising Data section ([Vol 6], Part B, Section 2.3.4.9) of the Bluetooth Core Specification Version 5.1.
GAP Advertiser¶
The application and profiles can directly call GAP API functions to perform Bluetooth Low Energy-related functions such as advertising or connecting. The GAP layer functionality is mostly defined in library code. The function headers can be found in gap.h. The advertising specific implementation is found in gap_advertiser.h. Most of these functions can be called directly.
The GAP Advertiser module lets you create advertisement sets. The application can then enable and disable the sets. This way, the application can quickly change the advertisement parameters and advertisement data. For example, an application can create an advertisement set for legacy advertising and one for long range advertising. If both sets are enabled, the application advertises both on the primary advertising channels with legacy advertising, and on a LE Coded PHY with the long range set. With long range advertising, more advertising data can be sent and connections can be formed from larger distances compared to legacy advertising.
Note
The advertisement data is de-coupled from the advertisement sets, so multiple sets can use the same advertisement data.
Multiple active advertising sets can set different advertising intervals. The
figure below illustrate how two advertising sets with different interval are
scheduled. The intervals does not need to be multiples of each other. In the
examples the advertising interval can be set in the .primIntMin
and
primIntMax
Advertisement Params (GapAdv_params_t
) member before the
advertisement set is enabled or during advertising as described in
Changing advertising parameters.
Listing 32. simple_peripheral.c :: SimplePeripheral_processGapMessage() :: GAP_DEVICE_INIT_DONE_EVENT - Change advertising interval before advertisment enable¶
1 2 3 4 advParams1.primIntMin = 160; // 100 ms advParams1.primIntMax = 160; // 100 ms advParams2.primIntMin = 800; // 500 ms advParams2.primIntMax = 800; // 500 ms
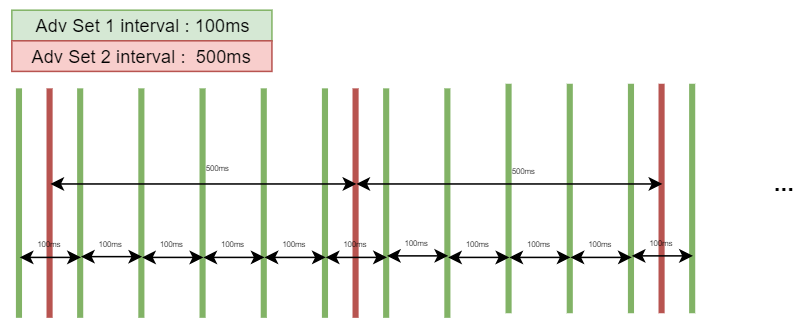
Figure 67. Multiple AE Advertising sets with different interval.
The following list describes how to configure the GAP layer for advertising. The simple peripheral example project will be used as an example.
Create Advertisement set with GapAdv_create(). The return value of GapAdv_create() is a handle for the advertisement set. The create function takes a GapAdv_params_t variable, so the first thing to do is to create this variable. The Tx Power of legacy advertisements cannot be individually set.
Note: The advertisement set creation can be done using SysConfig.
Some GapAdv_params_t variables are defined in gap_advertiser.h. In this example we will use one of them,
GAPADV_PARAMS_LEGACY_SCANN_CONN
. You can of course define your own GapAdv_params_t variable or modify an existing GapAdv_params_t variable.Listing 33. simple_peripheral.c :: SimplePeripheral_processGapMessage() :: GAP_DEVICE_INIT_DONE_EVENT - Creating an advertising parameters variable.¶1 2
// Temporary memory for advertising parameters. These will be copied by the GapAdv module GapAdv_params_t advParamLegacy = GAPADV_PARAMS_LEGACY_SCANN_CONN;
1 2
// Advertising handle static uint8 advHandleLegacy;
Listing 35. simple_peripheral.c :: SimplePeripheral_processGapMessage() :: GAP_DEVICE_INIT_DONE_EVENT - Create advertisement set and assign handle¶1 2
// Create Advertisement set and assign handle status = GapAdv_create(&SimplePeripheral_advCallback, &advParamLegacy, &advHandleLegacy);
Set Advertiser’s virtual address set with GapAdv_setVirtualAdvAddr(). The handle for the advertisement set, returned from GapAdv_create(), can also be used to create a custom private address for that advertisement set. This allows different advertising sets to have different addresses. This is only applicable for legacy non-connectable and non-scannable advertising sets.
Listing 36. simple_peripheral.c :: SimplePeripheral_processGapMessage() :: GAP_DEVICE_INIT_DONE_EVENT - Set advertiser’s virtual address¶1 2
// Set virtual address status = GapAdv_setVirtualAdvAddr(advHandleLegacy, &bdAddr);
Load Advertisement and Scan Response data. Advertising and scan response data is decoupled from the advertising set. That is, it is possible for multiple advertising sets to use the same memory for advertising/scan response data. An advertising set could also use the same memory for its advertising and scan response data. (Note that this requires diligence as there are some data types that are only allowed once in the advertisement and scan response data. See the Bluetooth Core Specification Supplement (CSSv7) for specifics.)
If your advertisement set is not scannable, you can of course skip the last step (load scan response data). Example advertising and scan response data is shown in Listing 38. and Listing 39.
1 2 3 4 5 6 7
// Load advertising data that is statically allocated by the app status = GapAdv_loadByHandle(advHandleLegacy, GAP_ADV_DATA_TYPE_ADV, sizeof(advertData), advertData); // Load scan response data that is statically allocated by the app status = GapAdv_loadByHandle(advHandleLegacy, GAP_ADV_DATA_TYPE_SCAN_RSP, sizeof(scanRspData), scanRspData);
// Advertisement data static uint8_t advertData[] = { 0x02, // length of this data GAP_ADTYPE_FLAGS, DEFAULT_DISCOVERABLE_MODE | GAP_ADTYPE_FLAGS_BREDR_NOT_SUPPORTED, // service UUID, to notify central devices what services are included // in this peripheral 0x03, // length of this data GAP_ADTYPE_16BIT_MORE, // some of the UUID's, but not all LO_UINT16(SIMPLEPROFILE_SERV_UUID), HI_UINT16(SIMPLEPROFILE_SERV_UUID) };
// Scan Response Data static uint8_t scanRspData[] = { // complete name 17, // length of this data GAP_ADTYPE_LOCAL_NAME_COMPLETE, 'S', 'i', 'm', 'p', 'l', 'e', 'P', 'e', 'r', 'i', 'p', 'h', 'e', 'r', 'a', 'l', // connection interval range 5, // length of this data GAP_ADTYPE_SLAVE_CONN_INTERVAL_RANGE, LO_UINT16(DEFAULT_DESIRED_MIN_CONN_INTERVAL), // 100ms HI_UINT16(DEFAULT_DESIRED_MIN_CONN_INTERVAL), LO_UINT16(DEFAULT_DESIRED_MAX_CONN_INTERVAL), // 1s HI_UINT16(DEFAULT_DESIRED_MAX_CONN_INTERVAL), // Tx power level 2, // length of this data GAP_ADTYPE_POWER_LEVEL, 5 // 5dBm };
Set which events to send to application. The events will be sent to the callback function given in GapAdv_create() (in this case
SimplePeripheral_advCallback()
).Listing 40. simple_peripheral.c :: SimplePeripheral_processGapMessage() :: GAP_DEVICE_INIT_DONE_EVENT - Setting advertising mask.¶1 2 3 4 5
// Set event mask status = GapAdv_setEventMask(advHandleLegacy, GAP_ADV_EVT_MASK_START_AFTER_ENABLE | GAP_ADV_EVT_MASK_END_AFTER_DISABLE | GAP_ADV_EVT_MASK_SET_TERMINATED);
Enable advertising. Calling GapAdv_enable() will start advertising.
Listing 41. simple_peripheral.c :: SimplePeripheral_processGapMessage() :: GAP_DEVICE_INIT_DONE_EVENT - Start advertising.¶1 2
// Enable advertising status = GapAdv_enable(advHandleLegacy, GAP_ADV_ENABLE_OPTIONS_USE_MAX , 0);
The above steps can be repeated to create multiple advertising sets. There is an upper limit of six advertising sets (defined in the controller). The heap may also restrict the number of advertising sets you can create in one application.
Changing advertising parameters¶
In order to change an individual parameter after advertising has been enabled, advertising must first be disabled. Re-enable advertising after the parameter is modified.
1 2 3 4 5 6 7 8 9 | // Stop advertising
GapAdv_disable(advHandleLegacy);
// Set a parameter
uint32_t newAdvInt = 200;
GapAdv_setParam(advHandleLegacy, GAP_ADV_PARAM_PRIMARY_INTERVAL_MIN, &newAdvInt);
// Re-enable advertising
GapAdv_enable(advHandleLegacy, GAP_ADV_ENABLE_OPTIONS_USE_MAX , 0);
|
Ending Advertisement¶
An advertising set can be disabled with GapAdv_disable() and deleted (such that all memory related to the set is freed) with GapAdv_destroy().
1 2 3 4 5 6 | // Stop advertising
GapAdv_disable(advHandleLegacy);
// Free all data related to advertising set (besides advertising / scan response data).
// Note that this can be called while advertising is still enabled
GapAdv_destroy(advHandleLegacy, GAP_ADV_FREE_OPTION_DONT_FREE);
|
The advertising and scan response data is statically allocated and is thus not freed as part of GapAdv_destroy().
Updating Advertisement/Scan Response Data¶
Again, since the advertising and scan response data is decoupled from the advertising set, it is possible for multiple advertising sets to use the same memory for advertising/scan response data. An advertising set could also use the same memory for its advertising and scan response data. The memory used for advertising/scan response data is referred to as a “buffer” throughout this section.
The preferred and safest method to update a buffer is to use the prepare/load latching mechanism. This will ensure the following:
- No buffer is freed if it is used by another advertising set.
- Advertising is always disabled before the buffer is modified, thus ensuring that no corrupted advertisement packets are transmitted.
- Prevent double-copying.
The following offers several ways to update the advertising and scan response data.
Update the Advertising/Scan response Data of a Single Handle¶
If the application wants to modify a few bytes of advertising/scan response data that is used by a single advertisement set, use GapAdv_prepareLoadByHandle() and GapAdv_loadByHandle().
1 2 3 4 5 6 7 8 | // Don't free anything since we're going to use the same buffer to re-load
GapAdv_prepareLoadByHandle(advHandleLegacy, GAP_ADV_FREE_OPTION_DONT_FREE);
// Sample buffer modification
advertData[3] = 0xAA;
// Reload buffer to handle
GapAdv_loadByHandle(advHandleLegacy, GAP_ADV_DATA_TYPE_ADV, ADV_DATA_LEN, advertData);
|
The GapAdv_prepareLoadByHandle() will perform the following here:
- Check that this buffer isn’t used by other handles. If GapAdv_prepareLoadByHandle() returns success, the application will know that it can now safely modify this buffer.
- Automatically disable advertising and mark it for re-enabling
GapAdv_loadByHandle() will automatically re-enable advertising with the updated buffer. This method can also be used to use a subset of the original advertising data. In this case, simply update the data length parameter of GapAdv_loadByHandle().
Load a New Buffer to a Single Advertising Handle¶
If the application wants to load a new buffer to a single advertising handle without double-copying, use GapAdv_prepareLoadByHandle() and GapAdv_loadByHandle(). This way, the advertising data exists in only one place in memory that is used by the GAP Advertiser. In this case we will free the buffer and allocate a new one.
1 2 3 4 5 6 7 8 | // Free the buffer (to avoid double copying) since we're loading a new buffer
GapAdv_prepareLoadByHandle(advHandleLegacy, GAP_ADV_FREE_OPTION_ADV_DATA);
// Allocate new buffer (and then fill it as desired)
uint8_t *advertData2= ICall_malloc(ADV_DATA2_LEN);
// Load the new buffer to the advertisement set handle
GapAdv_loadByHandle(advHandleLegacy, GAP_ADV_DATA_TYPE_ADV, ADV_DATA2_LEN, advertData2);
|
GapAdv_prepareLoadByHandle() will perform the following here:
- Check that the buffer isn’t used by any other advertisement handles. If GapAdv_prepareLoadByHandle() returns success, the application will know that it can now safely modify this buffer.
- Automatically disable advertising and mark it for re-enabling
- Free the original buffer
The GapAdv_loadByHandle() will automatically re-enable advertising on the handle with the new buffer.
Update Advertising/Scan Response Data that is Used for Multiple Advertising Handles¶
This is the case where the application wants to modify a few bytes of advertising or scan response data that is shared among advertisement sets.
1 2 3 4 5 6 7 8 | // Don't free anything since we're going to use the same buffer to reload
GapAdv_prepareLoadByBuffer(advertData, FALSE);
// Sample buffer modification
advertData[3] = 0xAA;
// Reload the buffer to be used by the advertisement set handles
GapAdv_loadByBuffer(ADV_DATA_LEN, advertData);
|
GapAdv_prepareLoadByBuffer() will automatically disable advertising for all advertising handles that use this buffer. GapAdv_loadByBuffer() will automatically re-enable advertising for all handles that use this buffer.
Load a New Buffer To Multiple Advertising Handles¶
This is the case where the applications wants to load a new buffer to all advertisement set handles that are using this buffer.
1 2 3 4 5 6 7 8 | // Free buffer (to avoid double copying) since we're loading a new buffer
GapAdv_prepareLoadByBuffer(advertData, TRUE);
// Allocate new buffer (and then fill as desired)
uint8_t *advertData2= ICall_malloc(ADV_DATA2_LEN);
// Reload the buffer to be used by all the handles
GapAdv_loadByBuffer(ADV_DATA2_LEN, advertData2);
|
GapAdv_loadByBuffer() will perform the following here:
- Automatically disable advertising and mark it for re-enabling on all handles that use this buffer
- Free the original buffer
GapAdv_loadByBuffer() will automatically re-enable advertising on all handles that used the original buffer.
Directly Manipulating a Buffer While Advertising is Enabled¶
Since the application owns the advertising and scan response buffers and thus has access to the memory where the buffers are stored, there is nothing preventing it from directly modifying this memory. While discouraged, there are scenarios where this can be useful, such as updating the data after each advertisement in the most power-efficient manner. The main drawback to this is that there is no way to guarantee that this update won’t occur while an advertising packet is being transmitted that is using this buffer, potentially resulting in a corrupted advertising packet. This is especially true if multiple advertising sets are using the same buffer. If the application accepts the risk of potentially corrupting an advertising packet, there is a recommended way to do this with minimized risk.
The buffer should be updated directly in the advertising callback (in the stack context)
when the GAP_EVT_ADV_END
is received. Generally, it is recommended to
minimize processing in these callbacks and, instead, to post an event to process
in the application context. It should be noted that any processing in the stack
context has the potential to break the timing of the controller. This should not
be a problem if the only processing is to update the buffer. Also, for both this
reason and because it will lead to a semaphore that never gets posted due to
calling an ICall API from the stack context, it should be noted that no stack
API calls can be made from the stack context. To reiterate for clarity, no BLE-Stack APIs
can be called from the callback (stack) context.
1 2 3 4 5 6 7 8 9 10 | // Callback passed into GapAdv_create(). This code is running in the stack context.
static void SimplePeripheral_advCallback(uint32_t event, void *pBuf, uintptr_t arg)
{
// Advertisement just ended so it should be safe to update buffer here
// without corrupting an advertisement.
if (event == GAP_EVT_ADV_END)
{
advertData[3] = 0xAA;
}
}
|
Note that this can not be guaranteed to work all the time without corrupting an
advertisement. Also, if the buffer is used by multiple advertisement handles, the
application would need to track the GAP_EVT_ADV_ENDs
across all of these handles to
find an ideal time to update the buffer. If this processing is added to the callback
in the stack context, this increases the risk of breaking the timing of the
controller. Also, remember to include GAP_EVT_ADV_END
in the event mask
passed in GapAdv_setEventMask().
Limited Advertising¶
The above code sets the advertising interval for limited and general
advertising modes. By default, the peripheral advertises in general
discoverable mode. To use limited discoverable mode, the
corresponding fields inside the advertising data packet should be
changed by defining DEFAULT_DISCOVERABLE_MODE
to
GAP_ADTYPE_FLAGS_LIMITED
. The application is responsible for setting the advertising
data and advertising duration.
GAP Peripheral¶
The peripheral role is demonstrated in simple_peripheral.c and simple_peripheral.h. The Peripheral role demonstrates the use of the advertising and connection states. The steps to use this role are as follows:
Initialize the GAP parameters. This initialization should occur in the application initialization function, for example in
SimplePeripheral_init
as shown below.1 2 3 4 5 6 7
// Configure GAP { uint16_t paramUpdateDecision = DEFAULT_PARAM_UPDATE_REQ_DECISION; // Pass all parameter update requests to the app for it to decide GAP_SetParamValue(GAP_PARAM_LINK_UPDATE_DECISION, ¶mUpdateDecision); }
Initialize the application task for the Peripheral Role and register to receive GAP events.
Listing 50. simple_peripheral.c :: SimplePeripheral_init() - Initialize the GAP layer and register for GAP events.¶1 2
//Initialize GAP layer for Peripheral role and register to receive GAP events GAP_DeviceInit(GAP_PROFILE_PERIPHERAL, selfEntity, addrMode, NULL);
Now you can send commands from the application. The following is an example of the application initiating PHY change using HCI_LE_SetPhyCmd().
1 2
// Send PHY Update HCI_LE_SetPhyCmd(connHandle, allPhys, txPhy, rxPhy, phyOpts);
The following shows the software flow when the user chooses to set the PHY preference from the terminal in simple_peripheral example:
Figure 68. Context Diagram of Application Updating PHY.¶
As shown in the diagram above, the actual PHY change is returned asynchronously and is passed to the application with event code HCI_BLE_PHY_UPDATE_COMPLETE_EVENT.
The application task processes most of the GAP-related events passed to it from the Bluetooth Low Energy protocol stack. For example, when a link is terminated, the application automatically restarts advertising. The following code snippet can be found in
simple_peripheral.c
:Listing 52. simple_peripheral.c :: SimplePeripheral_processGapMessage() - Restart advertising after disconnect¶1 2 3 4 5 6 7 8 9 10 11 12
static void SimplePeripheral_processGapMessage(gapEventHdr_t *pMsg) { //....... case GAP_LINK_TERMINATED_EVENT: { //....... // Restart advertising since there is now an active connection GapAdv_enable(advHandleLegacy, GAP_ADV_ENABLE_OPTIONS_USE_MAX , 0); //....... break;
GAP Scanner¶
The GAP Scanner performs Extended Scanning and Legacy Scanning operations as defined by the Bluetooth Core Specification Version 5.1. It controls the Scanner GAP state (see GAP State Diagram.). The Central and Observer role uses the scanning state implemented by the GAP Scanner. The GAP Scanner is demonstrated in the simple central and simple observer example projects. See the BLE Stack API Reference for the full GAP Scanner API including commands, configurable parameters, events, and callbacks. The steps to use this module are listed in the following, along with example code from simple central.
Start a Central or Observer GAP role. In this case we will use the GAP Central role.
// Initialize GAP layer for Central role and register to receive GAP events GAP_DeviceInit(GAP_PROFILE_CENTRAL, selfEntity, addrMode, NULL);
Set up a callback function for scanner events and register to gap_advertiser.h. Since the callback (in this case
SimpleCentral_scanCb()
) is called from the stack, as little processing as possible should happen in it.Listing 54. simple_central.c :: SimpleCentral_processGapMsg() :: GAP_DEVICE_INIT_DONE_EVENT - Initialize the GAP central role.¶// Register callback to process Scanner events GapScan_registerCb(SimpleCentral_scanCb, NULL);
Set which events to pass to application.
Listing 55. simple_central.c :: SimpleCentral_processGapMsg() :: GAP_DEVICE_INIT_DONE_EVENT - Set which events to pass to the callback function.¶// Set Scanner Event Mask GapScan_setEventMask(GAP_EVT_SCAN_ENABLED | GAP_EVT_SCAN_DISABLED | GAP_EVT_ADV_REPORT);
Set scan parameters. It’s worth noting that the parameters are split into PHY-related parameters (set with GapScan_setPhyParams()) and non-PHY-related parameters (set with GapScan_setParam()). Remember to set both.
Listing 56. simple_central.c :: SimpleCentral_processGapMsg() :: GAP_DEVICE_INIT_DONE_EVENT - Set PHY-related GAP scanner parameters.¶// Set Scan PHY parameters GapScan_setPhyParams(DEFAULT_SCAN_PHY, SCAN_TYPE_ACTIVE, SCAN_PARAM_DFLT_INTERVAL, SCAN_PARAM_DFLT_INTERVAL);
Set which advertising report fields to send to the application (to the callback function, in this case
SimpleCentral_scanCb()
).Listing 57. simple_central.c :: SimpleCentral_processGapMsg() :: GAP_DEVICE_INIT_DONE_EVENT - Set GAP scanner parameter.¶// Set Advertising report fields to keep temp16 = SC_ADV_RPT_FIELDS; GapScan_setParam(SCAN_PARAM_RPT_FIELDS, &temp16);
Enable filter to remove duplicate advertising reports.
Listing 58. simple_central.c :: SimpleCentral_processGapMsg() :: GAP_DEVICE_INIT_DONE_EVENT - Set GAP scanner parameter.¶// Set LL Duplicate Filter temp8 = SCAN_FLT_DUP_ENABLE; GapScan_setParam(SCAN_PARAM_FLT_DUP, &temp8);
Set which PHY to scan on.
Listing 59. simple_central.c :: SimpleCentral_processGapMsg() :: GAP_DEVICE_INIT_DONE_EVENT - Set GAP scanner parameter.¶// Set Scanning Primary PHY temp8 = DEFAULT_SCAN_PHY; GapScan_setParam(SCAN_PARAM_PRIM_PHYS, &temp8);
Set scan filter to filter by PDU type.
// Set PDU type filter - // Only 'Connectable' and 'Complete' packets are desired. // It doesn't matter if received packets are // Scannable or Non-Scannable, Directed or Undirected, // Scan_Rsp's or Advertisements, and whether they are Legacy or Extended. temp16 = SCAN_FLT_PDU_CONNECTABLE_ONLY | SCAN_FLT_PDU_COMPLETE_ONLY; GapScan_setParam(SCAN_PARAM_FLT_PDU_TYPE, &temp16);
Start scanning. Remember to reset the number of scan results every time you start scanning.
// Scanning for DEFAULT_SCAN_DURATION x 10 ms. // The stack does not need to record advertising reports // since the application will filter them by Service UUID and save. // Reset number of scan results to 0 before starting scan numScanRes = 0; GapScan_enable(0, DEFAULT_SCAN_DURATION, 0);
The return status from the protocol stack call of GapScan_enable() indicates only whether or not the attempt to perform device discovery was successful. The actual device discovered is returned asynchronously as a
SC_EVT_ADV_REPORT
forwarded through the GAP Scanner callbacks registered by the application (here:SimpleCentral_scanCb()
). This is described below.The GAP Scanner performs some processing on the GAP events it receives from the protocol stack. The task also forwards some events to the application. Figure 69. shows this and how the
SC_EVT_ADV_REPORT
is processed from the protocol stack to the application.Figure 69. Context Diagram of Application using GapScan_enable()¶
Note that during scanning, individual advertisements and scan responses are
returned as SC_EVT_ADV_REPORT
.This is defined by the Bluetooth Core Specification Version 5.1. By
default, duplicate reports are filtered such that only one event is returned to
the application per peer device BDA. This can be configured via the
SCAN_PARAM_FLT_DUP GAP Scanner parameter.
SC_EVT_SCAN_ENABLED
indicates the start of scanning. After the scan
has completed, a summary of discovered reports will be returned to the
application with SC_EVT_SCAN_DISABLED
. You can see the implementation
of this in SimpleCentral_processAppMsg()
.
The maximum amount of scan responses that can be discovered during one scan can
be set with the DEFAULT_MAX_SCAN_RES
parameter that is passed into the maxNumReport
parameter of GapScan_enable().
In an environment saturated with advertisements and scan responses, this can have a drastic impact on heap usage to the point of potentially breaking the stack. Therefore, it is essential to profile your application for the worst-case scenario where the maximum amount of scan responses are discovered during a scan.
You can change scanning parameters with GapScan_setParam(). Note that some scanning parameters will not be updated before scanning has been disabled and re-enabled. This is true for the following parameters:
- SCAN_PARAM_FLT_PDU_TYPE - Filter by PDU Type
- SCAN_PARAM_FLT_MIN_RSSI - Filter by Minimum RSSI
- SCAN_PARAM_FLT_DISC_MODE - Filter by Discoverable Mode
- SCAN_PARAM_RPT_FIELDS - Advertising Report Fields
Advertising Report Recording¶
In addition to the advertising report, the advertising report recording feature can be used to record only a certain part of the Advertising Report information without the data payload. The application can specify which fields of the Advertising Report information by using GapScan_setParam() with the parameter ID SCAN_PARAM_RPT_FIELDS and the associate bitmap. This is useful in the use case where the application is not interested in the payload of Advertising Reports thus doesn’t want to get GAP_EVT_ADV_REPORT event from GAP Scanner but needs some specific information such as address type, address, RSSI, etc.. To prepare recording, GAP Scanner allocates necessary amount of memory in GapScan_enable(). Then whenever GAP Scanner gets a new packet, it puts only the specified fields of the information in the Advertising Report List in packed form. The list is kept in RAM even after the scanning ends until the application calls GapScan_discardAdvReportList() or a new scanning starts. While the list is available, the application can retrieve the information by using GapScan_getAdvReport(). When the list gets full, GAP Scanner issues SCAN_EVT_ADV_REPORT_FULL to the application to notify that no more Advertising Report information will be recorded. How many Advertising Reports’ information have been recorded can be found in the numReport field of the data buffer of type GapScan_Evt_End_t, coming with GAP_EVT_SCAN_END event. Alternatively, if the application didn’t mask GAP_EVT_SCAN_END and doesn’t get it, GapScan_getParam() with parameter ID SCAN_PARAM_NUM_ADV_RPT can be used.
Filtering¶
The GAP Scanner module provides 5 different types of filter to reduce the amount of advertising report notifications to the application and eventually save memory and power consumption. The result of the filtering affects both advertising reports and advertising report recording. The packets filtered out by the filter configurations are discarded and will neither be reported nor recorded. The filters are set by using the API GapScan_setParam() with the following parameter IDs:
- Filter by LL Filter Policy: SCAN_PARAM_FLT_POLICY
- Filter by PDU Type: SCAN_PARAM_FLT_PDU_TYPE
- Filter by Minimum RSSI: SCAN_PARAM_FLT_MIN_RSSI
- Filter by Discoverable Mode: SCAN_PARAM_FLT_DISC_MODE
- Filter by Duplicates: SCAN_PARAM_FLT_DUP.
All parameter IDs are described in the following sections. The GAP Scanner allows the application to apply any combination of the individual filters at the same time to narrow the scope of packets to receive.
Filter by LL Filter Policy¶
This filter is a link layer-level filter. The associated parameter ID used in GapScan_setParam() is SCAN_PARAM_FLT_POLICY. The parameter accompanying SCAN_PARAM_FLT_POLICY is passed to the link layer when GapScan_enable() is called before actually enabling scanning. Since the filtering is done by the link layer, the application only receives advertising report events that have passed the filter. The parameter value can be one of the following:
Name | Value | Description |
---|---|---|
SCAN_FLT_POLICY_ALL | 0 | Accept all Advs except directed Adv not addressed to this device. |
SCAN_FLT_POLICY_WL | 1 | Accept only Advs from devices where the advertiser’s address is in the white list. |
SCAN_FLT_POLICY_ALL_RPA | 2 | Accept all Advs except directed Adv not addressed to this device and any packet addressed to this device or addressed to a private resolvable address. |
SCAN_FLT_POLICY_WL_RPA | 3 | Accept only Advs from devices where the advertiser’s address is in the White List and any packet addressed to this device or addressed to a private resolvable address. |
Filter by PDU Type¶
This filter is based on the Event_Type
parameter coming with the LE Extended
Advertising Report Event. The associated parameter ID is
SCAN_PARAM_FLT_PDY_TYPE
. The parameter value specifies packets
classified in six categories:
- Connectable/Non-Connectable
- Scannable/Non-Scannable
- Directed/Undirected
- ScanRsp/Adv
- Legacy/Extended
- Complete/Incomplete.
Every incoming packet has exactly one attribute in each category. For example,
SCAN_FLT_PDU_NONSCANNABLE_ONLY and
SCAN_FLT_PDU_SCANNABLE_ONLY cannot be chosen together since they
represent scannable and non-scannable packets. Only either one can be used. If
neither type is selected in a set, the filter will not care about that category.
For example, if neither SCAN_FLT_PDU_NONCONNECTABLE_ONLY nor
SCAN_FLT_PDU_CONNECTABLE_ONLY is set in the parameter value, the GAP
Scanner will notify the application of both connectable packets and
non-connectable packets. It will also record both connectable packets and
non-connectable packets. The SCAN_PARAM_FLT_PDY_TYPE
parameter value
can be any combination of the following individual values (except individual
values that are mutually exclusive):
Name | Value | Description |
---|---|---|
SCAN_FLT_PDU_NONCONNECTABLE_ONLY | 0x0001 | Non-connectable packets only. Mutually exclusive with SCAN_FLT_PDU_CONNECTABLE_ONLY |
SCAN_FLT_PDU_CONNECTABLE_ONLY | 0x0002 | Connectable packets only. Mutually exclusive with SCAN_FLT_PDU_NONCONNECTABLE_ONLY |
SCAN_FLT_PDU_NONSCANNABLE_ONLY | 0x0004 | Non-scannable packets only. Mutually exclusive with SCAN_FLT_PDU_SCANNABLE_ONLY |
SCAN_FLT_PDU_SCANNABLE_ONLY | 0x0008 | Scannable packets only. Mutually exclusive with SCAN_FLT_PDU_NONSCANNABLE_ONLY |
SCAN_FLT_PDU_UNDIRECTED_ONLY | 0x0010 | Undirected packets only. Mutually exclusive with SCAN_FLT_PDU_DIRECTIED_ONLY |
SCAN_FLT_PDU_DIRECTED_ONLY | 0x0020 | Directed packets only. Mutually exclusive with SCAN_FLT_PDU_UNDIRECTED_ONLY |
SCAN_FLT_PDU_ADV_ONLY | 0x0040 | Advertisement packets only. Mutually exclusive with SCAN_FLT_PDU_SCANRSP_ONLY |
SCAN_FLT_PDU_SCANRSP_ONLY | 0x0080 | Scan Response packets only. Mutually exclusive with SCAN_FLT_PDU_ADV_ONLY |
SCAN_FLT_PDU_EXTENDED_ONLY | 0x0100 | Extended packets only. Mutually exclusive with SCAN_FLT_PDU_LEGACY_ONLY |
SCAN_FLT_PDU_LEGACY_ONLY | 0x0200 | Legacy packets only. Mutually exclusive with SCAN_FLT_PDU_EXTENDED_ONLY |
SCAN_FLT_PDU_TRUNCATED_ONLY | 0x0400 | Truncated packets only. Mutually exclusive with SCAN_FLT_PDU_COMPLETE_ONLY |
SCAN_FLT_PDU_COMPLETE_ONLY | 0x0800 | Complete packets only. Mutually exclusive with SCAN_FLT_PDU_TRUNCATED_ONLY |
Filter by Minimum RSSI¶
This filter is based on the RSSI parameter coming with the LE Extended Advertising Report Event. The associated parameter ID used in GapScan_setParam() is SCAN_PARAM_FLT_MIN_RSSI. The GAP Scanner will discard LE Extended Advertising Report Event whose RSSI parameter value is smaller than the minimum RSSI value given by the application. The available range is -128 dBm to 127 dBm.
Filter by Discoverable Mode¶
This filter is based on the Flags AD
type value in the payload (in
the Data
parameter) of the LE Extended Advertising Report Event. The
associated parameter ID used in GapScan_setParam() is
SCAN_PARAM_FLT_DISC_MODE. This filter is applied after the GAP
Scanner reassembles all the fragmented payloads delivered by multiple LE
Extended Advertising Report Events. The GAP Scanner will discard the
defragmented packet if the found Flags AD type value doesn’t match the parameter
value given by the application. The parameter value can be one of the following:
Name | Value | Description |
---|---|---|
SCAN_FLT_DISC_NONE | 0 | Accept only Non-Discoverable mode |
SCAN_FLT_DISC_GENERAL | 1 | Accept only General Discoverable mode |
SCAN_FLT_DISC_LIMITED | 2 | Accept only Limited Discoverable mode |
SCAN_FLT_DISC_ALL | 3 | Accept both General and Limited Discoverable mode |
SCAN_FLT_DISC_DISABLE | 4 | Discoverable Mode Filter Off (Don’t care about the Flags AD type value) |
Filter by Duplicates¶
Like the Link Layer Scanner Filter Policy, this filter is a link layer-level filter.
The associated parameter ID used in GapScan_setParam() is
SCAN_PARAM_FLT_DUP. The parameter accompanying the
SCAN_PARAM_FLT_DUP parameter ID is passed to the link layer when
GapScan_enable() internally calls LE_SetExtScanEnable
to
enable scanning. Since the filtering is done by the link layer, GAP Scanner
receives only the LE Extended Advertising Report Events that have passed the
filter. The parameter value can be one of the following:
Name | Value | Description |
---|---|---|
SCAN_FLT_DUP_DISABLE | 0 | Disable duplicate filtering. |
SCAN_FLT_DUP_ENABLE | 1 | Enable duplicate filtering. |
SCAN_FLT_DUP_RESET | 2 | Enable duplicate filtering. Reset for each scan period. |
GAP Initiator¶
The initiator module is used to initiate the connection to a peripheral device. An initiator generally scans for advertisements then connects to a specific device. The initiator is a short lived state that transitions to the Master Role after a connection is established. Unlike the GAP Advertiser and GAP Scanner modules, GAP Initiator doesn’t have any callback function to call or to be called.
Only a device initiated with the GAP Central Role can become an initiator.
1 2 3 4 5 6 7 8 | void SimpleCentral_init(void)
{
// Register with GAP for HCI/Host messages (for RSSI)
GAP_RegisterForMsgs(selfEntity);
// Initialize GAP layer for Central role and register to receive GAP events
GAP_DeviceInit(GAP_PROFILE_CENTRAL, selfEntity, addrMode, NULL);
}
|
The following API is used to initiate connection to a peer device, see GapInit_connect()
1 2 3 4 5 6 7 8 9 10 11 12 13 | GapScan_Evt_AdvRpt_t advRpt;
GapScan_getAdvReport(index, &advRpt);
/*
* The initiating timeout is in milliseconds and will automatically cancel
* connection initiation if the peer is not connected. This can be set to
* 0 to wait indefinitely.
*/
uint16_t initTimeout = 500;
GapInit_connect(advRpt.addrType & MASK_ADDRTYPE_ID,
advRpt.addr, DEFAULT_INIT_PHY, initTimeout);
|
If the on-going connection attempt is intended to be canceled by either timeout or a user request (the application calling GapInit_cancelConnect()), the stack notifies the application of the cancellation completion with a GAP_CONNECTING_CANCELLED_EVENT.
The initiator task will use the parameters saved for the PHY specified in the
call to GapInit_connect(). These may be set with
GapInit_setPhyParam(). Defaults are shown in gap_initiator.h
GAP Central¶
The GAP Central role is demonstrated in simple_central.c and simple_central.h. The Central role demonstrates the use of the scanning and initiating states and supports connections to peripheral devices. See the simple_central example project for an example of implementing the Central role. The steps to use this module are as follows.
Initialize the GAP and GATT parameters. This initialization should occur in the application initialization function (for example in
SimpleCentral_init
). GAP parameters can also be set in this initialization function.static void SimpleCentral_init(void) { // Initialize GATT Client VOID GATT_InitClient(); ... // Accept all parameter update requests GAP_SetParamValue(GAP_PARAM_LINK_UPDATE_DECISION, GAP_UPDATE_REQ_ACCEPT_ALL); }
Start the GAP Central role and register to receive events in the application task.
// Initialize GAP layer for Central role and register to receive GAP events GAP_DeviceInit(GAP_PROFILE_CENTRAL, selfEntity, addrMode, NULL);
GAP Connection State¶
A BLE device can be either in the Master (Central) or Slave (Peripheral) role in the Connection State. The following are relevant parameters for the both roles in the Connection State.
Connection Parameters¶
This section describes the connection parameters which are sent by the initiating device with the connection request and can be modified by either device when the connection is established. These parameters are as follows:
- Connection Interval - In Bluetooth Low Energy connections, a
frequency-hopping scheme is used. The two devices each send and
receive data from one another only on a specific channel at a
specific time. These devices meet a specific amount of time later
at a new channel (the link layer of the Bluetooth Low Energy
protocol stack handles the channel switching). This meeting is
where the two devices send and receive data is known as a
connection event
. If there is no application data to be sent or received, the two devices exchange link layer data to maintain the connection. The connection interval is the amount of time between two connection events in units of 1.25 ms. The connection interval can range from a minimum value of 6 (7.5 ms) to a maximum of 3200 (4.0 s). See Connection Event and Interval for more details.
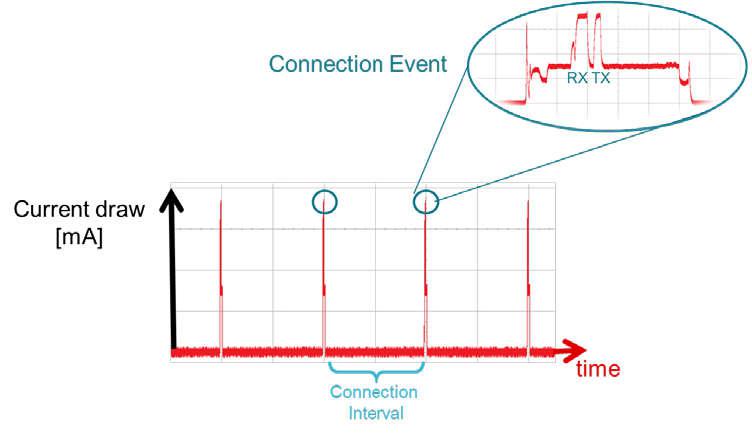
Figure 70. Connection Event and Interval
Different applications may require different connection intervals. As described in Connection Parameter Considerations, these requirements affect the power consumption of the device. For more detailed information on power consumption, see the Measuring Bluetooth Smart Power Consumption Application Report (SWRA478).
- Slave Latency - This parameter gives the slave (peripheral) device the option of skipping a number of connection events. This ability gives the peripheral device some flexibility. If the peripheral does not have any data to send, it can skip connection events, stay asleep, and save power. The peripheral device selects whether to wake or not on a per connection event basis. The peripheral can skip connection events but must not skip more than allowed by the slave latency parameter or the connection fails. See Slave Latency for more details.
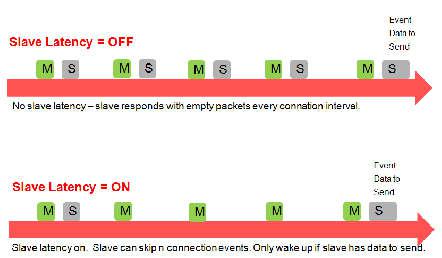
Figure 71. Slave Latency
- Supervision Time-out - This time-out is the maximum amount of time between two successful connection events. If this time passes without a successful connection event, the device terminates the connection and returns to an unconnected state. This parameter value is represented in units of 10 ms. The supervision time-out value can range from a minimum of 10 (100 ms) to 3200 (32.0 s). The time-out must be larger than the effective connection interval (see Effective Connection Interval for more details).
Effective Connection Interval¶
The effective connection interval is equal to the amount of time between two connection events, assuming that the slave skips the maximum number of possible events if slave latency is allowed (the effective connection interval is equal to the actual connection interval if slave latency is set to 0).
The slave latency value represents the maximum number of events that can be skipped. This number can range from a minimum value of 0 (meaning that no connection events can be skipped) to a maximum of 499. The maximum value must not make the effective connection interval (see the following formula) greater than 16 s. The interval can be calculated using the following formula:
Effective Connection Interval = (Connection Interval) * (1 + [Slave Latency])
Consider the following example:
- Connection Interval: 80 (100 ms)
- Slave Latency: 4
- Effective Connection Interval: (100 ms) * (1 + 4) = 500 ms
When no data is being sent from the slave to the master, the slave transmits during a connection event once every 500 ms.
Connection Parameter Considerations¶
In many applications, the slave skips the maximum number of connection events. Consider the effective connection interval when selecting or requesting connection parameters. Selecting the correct group of connection parameters plays an important role in power optimization of the Bluetooth Low Energy device. The following list gives a general summary of the trade-offs in connection parameter settings.
Reducing the connection interval does as follows:
- Increases the power consumption for both devices
- Increases the throughput in both directions
- Reduces the time for sending data in either direction
Increasing the connection interval does as follows:
- Reduces the power consumption for both devices
- Reduces the throughput in both directions
- Increases the time for sending data in either direction
Reducing the slave latency (or setting it to zero) does as follows:
- Increases the power consumption for the peripheral device
- Reduces the time for the peripheral device to receive the data sent from a central device
Increasing the slave latency does as follows:
- Reduces power consumption for the peripheral during periods when the peripheral has no data to send to the central device
- Increases the time for the peripheral device to receive the data sent from the central device
Connection Parameter Limitations with Multiple Connections¶
There are additional constraints that exist when connected to multiple devices or performing multiple GAP roles simultaneously. See the multi_role example in BLE5-Stack 2.01.03.00.
Connection Parameter Update¶
In some cases, the central device requests a connection with a
peripheral device containing connection parameters that are
unfavorable to the peripheral device. In other cases, a peripheral
device might have the desire to change connection parameters in the middle of a
connection, based on the peripheral application. The peripheral
device can request the central device to change the connection
parameters by sending a Connection Parameter Update Request
.
For Bluetooth 4.1, 4.2, 5.0 and 5.1-capable devices, this request is handled directly by
the Link Layer. For Bluetooth 4.0 devices, the L2CAP layer of the
protocol stack handles the request. The Bluetooth Low Energy stack
automatically selects the update method.
The Connection Parameter Update Request
contains four parameters:
- minimum connection interval
- maximum connection interval
- slave latency
- connection time-out.
These values represent the parameters that the peripheral device wants for
the connection. The connection interval is given as a range. When
the central device receives the Connection Parameter Update Request
request,
it can accept or reject the new parameters.
Sending a Connection Parameter Update Request
is optional and it is not
required for the central device to accept or apply the requested
parameters. Some applications try to establish a connection at a
faster connection interval to allow for a faster service discovery
and initial setup. These applications later request a longer
(slower) connection interval for optimal power usage.
Regardless of the role (peripheral or central), connection parameter updates can
be sent asynchronously with the GAP_UpdateLinkParamReq() command. The
simple_peripheral application can be configured to automatically send a
parameter update a certain amount of time after establishing a connection. For
example, the simple_peripheral application uses the following symbols, defined
in simple_peripheral.c
:
#define DEFAULT_DESIRED_MIN_CONN_INTERVAL 80
#define DEFAULT_DESIRED_MAX_CONN_INTERVAL 800
#define DEFAULT_DESIRED_SLAVE_LATENCY 0
#define DEFAULT_DESIRED_CONN_TIMEOUT 1000
#define SP_SEND_PARAM_UPDATE_DELAY 6000
In simple_peripheral, six seconds after a connection is established the application automatically sends a GAP Update Link Parameter Request:
1 2 3 4 5 6 7 8 9 10 11 | static void SimplePeripheral_processParamUpdate(uint16_t connHandle)
{
gapUpdateLinkParamReq_t req;
req.connectionHandle = connHandle;
req.connLatency = DEFAULT_DESIRED_SLAVE_LATENCY;
req.connTimeout = DEFAULT_DESIRED_CONN_TIMEOUT;
req.intervalMin = DEFAULT_DESIRED_MIN_CONN_INTERVAL;
req.intervalMax = DEFAULT_DESIRED_MAX_CONN_INTERVAL;
// Send parameter update
bStatus_t status = GAP_UpdateLinkParamReq(&req);
|
When the peer device receives this update request, the GAP parameter GAP_PARAM_LINK_UPDATE_DECISION determines how it responds. See GAP Peripheral for an explanation of how the parameters are configured.
Connection Termination¶
Either the master or the slave can terminate a connection for any reason. One side initiates termination and the other side must respond before both devices exit the connected state. Use the GAP_TerminateLinkReq() command to terminate an existing connection.