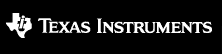 |
 |
Go to the documentation of this file.
33 #ifndef _IEC60730_USER_CONFIG_H_
34 #define _IEC60730_USER_CONFIG_H_
66 #define JUMP_TO_FAILSAFE 1
112 #define MAIN_CLOCK_FREQUENCY_12MHz
128 #define MAIN_CLOCK_DIVIDER 1
134 #define LFXT1_FREQUENCY 32768
135 #define LFXT1_FREQUENCY_DIVIDER 1
140 #define PERCENT_FREQUENCY_DRIFT 3
153 #if defined(__MSP430F5529__)
154 #define RAM_START_ADDRESS 0x2400
155 #define RAM_SIZE 0x1FF0
156 #define STACK_SIZE 160
157 #elif defined(__MSP430G2553__)
158 #define RAM_START_ADDRESS 0x0200
159 #define RAM_SIZE 0x01F0
160 #define STACK_SIZE 80
161 #elif defined(__MSP430FR5739__)
162 #define RAM_START_ADDRESS 0x1C00
163 #define RAM_SIZE 0x03F0
164 #define STACK_SIZE 160
169 #define RAM_START_ADDRESS
178 #define RAM_END_ADDRESS ((RAM_START_ADDRESS + RAM_SIZE)-STACK_SIZE)
193 #define NON_DESTRUCTIVE
198 #define RAM_TEST_BUFSIZE 8
214 #define CRC16_CCITT_SEED 0xFFFF
222 #ifndef __MSP430_HAS_CRC__
223 #define CRC16_CCITT_POLY 0x1021
235 #if defined(__MSP430F5529__)
236 #define CRC_CHECKSUM_LOCATION 0x1800
237 #elif defined(__MSP430G2553__)
238 #define CRC_CHECKSUM_LOCATION 0x1004
239 #elif defined(__MSP430FR5739__)
240 #define CRC_CHECKSUM_LOCATION 0x1880
242 #define CRC_CHECKSUM_LOCATION
254 #define MINIMUM_ADC_COUNT_DRIFT -50
255 #define MAXIMUM_ADC_COUNT_DRIFT 50
263 #define SIG_CPU_REG_TEST 0xCC
264 #define SIG_PC_REG_TEST 0xCD
265 #define SIG_CLOCK_TEST 0xCE
266 #define SIG_RAM_TEST 0xCF
267 #define SIG_NV_MEM_CRC_TEST 0xD0
268 #define SIG_ADC_TEST 0xD1
269 #define SIG_GPIO_TEST 0xD2
270 #define SIG_INTERRUPT_TEST 0xD3
279 #define TEST_FAILURE 0x00
Copyright 2015, Texas Instruments Incorporated