Software Architecture¶
The TI royalty-free Bluetooth low energy protocol stack is a software component in the CC26x2 SDK for developing single-mode Bluetooth low energy (BLE) standalone and network processor applications. This component is based on the SimpleLink CC26x2 family of BLE enabled wireless MCUs. The CC26x2 combines a 2.4-GHz RF transceiver (some wireless MCUs may also contain a Sub-1GHz transceiver which is not used with BLE), 352 KB of in-system programmable flash memory, 80 KB of SRAM, and a full complement of peripherals. The CC26x2 wireless MCU is centered on an ARM® Cortex®-M4F series processor that handles the application layer and Bluetooth low energy protocol stack, and an autonomous radio core centered on an ARM Cortex-M0 processor that handles all the low-level radio control and processing associated with the physical layer (PHY). The Sensor Controller Engine (SCE) block provides additional flexibility by allowing autonomous data acquisition and processing independent of the Cortex-M4F processor, further extending the low-power capabilities of the CC26x2. For more information on the CC26x2, see the CC26x2 Technical Reference Manual.
The application developer interfaces with the protocol stack through a set of C APIs (ICall) to implement a Bluetooth low energy application. Although applications can be created using C++, all BLE protocol stack APIs must be accessed through the C context. The rest of this document intends to document application development on the CC26x2 using the Bluetooth low energy stack.
BLE5-Stack Protocol Stack and Application Configurations¶
The BLE stack platform supports two different protocol stack and application operational configurations:
- Single device: The BLE controller, host, profiles, and application are all implemented on the wireless MCU as a true single-chip solution. This configuration is the simplest and most common when using the CC26x2. This configuration is used by most of TI’s sample projects. This configuration is the most cost-effective technique and provides the lowest-power performance.
- Network processor: The BLE controller and host reside on the wireless MCU with the application and profile residing on an external application processer (AP). Communication with the wireless MCU occurs over a UART or SPI interface using the serialized Network Processor Interface (NPI) protocol. Using NPI, the AP controls the network processor with a combination of TI Vendor Specific Host Controller Interface (HCI) commands and Bluetooth HCI commands. The network processor option is ideal for adding BLE to an existing non-wireless application. It is important to note that the network processor is not a pure HCI LE controller-only implementation and the application must use TI Vendor Specific HCI commands for BLE Host operations.
Solution Platform¶
This section describes the various components that are installed with BLE5-Stack 1.01.01.00 and the directory structure of the protocol stack and any tools required for development.
Figure 1. shows the BLE5-Stack development system. Unless otherwise noted, BLE5-Stack applications must be built with component from this CC26x2 SDK.
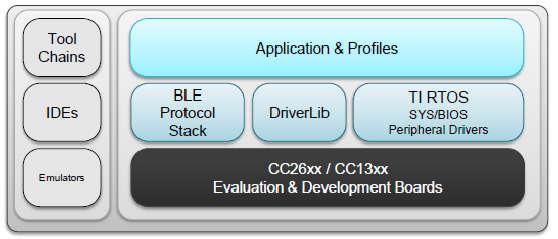
Figure 1. Bluetooth low energy Stack Development System
The solution platform includes the following components within the CC26x2 SDK:
- TI’s Real-Time Operating System (TI-RTOS) with the TI-RTOS SYS/BIOS kernel, optimized power management support, and peripheral drivers (SPI, UART, and so forth).
- DriverLib provides a device register abstraction layer and is used by software and drivers to control the CC26x2 at the lowest level.
- The Bluetooth low energy protocol stack is provided in library form with parts of the protocol stack in the CC26x2 ROM.
- Sample applications and profiles make starting development BLE application using both custom and adopted solutions easier.
The following integrated development environments (IDEs) are supported:
- IAR Embedded Workbench for ARM
- Code Composer Studio™ (CCS) using the TI ARM Compiler
Refer to the SDK release notes for the specific IDE & toolchain versions supported by this release.
BLE Software Architecture¶
The CC26x2 Bluetooth low energy software environment consists of the following parts:
- An application image with the TI-RTOS kernel, drivers and Bluetooth profile
- A stack library that implements Bluetooth low energy Host and Controller protocol
TI-RTOS is a real-time, pre-emptive, multithreaded operating system that runs the software solution with task synchronization. Both the application and Bluetooth low energy protocol stack exist as separate tasks within the RTOS. The Bluetooth low energy protocol stack has the highest priority. A messaging framework called indirect call (ICall) is used for thread-safe synchronization between the application and stack. It is mandatory to use TI-RTOS and ICall when developing an application with the BLE Stack.
- The stack image includes the lower layers of the Bluetooth low energy protocol stack from the Link Layer up to and including the GAP, GATT and Security Manager (SM) layers. Most of the Bluetooth low energy protocol stack code is provided as a library.
- The application image includes the RTOS, profiles/services, application code, drivers, and the ICall module.
Stack Library Configuration¶
In BLE5-Stack 1.01.01.00, stack is built as a library that is statically linked to the application. Using this build configuration yields additional flash footprint optimizations by the linker since the application and stack can share contiguous flash pages. See the example project’s README file for the available project build configurations.
Stack library projects have the following properties:
- Stack project generates a static library (.lib)
- Application project will link the stack in as a library
- There is no explicit app/stack boundary. The application’s link step decides the memory locations of the code within the stack library. There are some exceptions to this such as SNV.
- This architecture saves flash by allowing the linker work more efficiently.
- These projects used the improved ICall architecture
Standard Project Task Hierarchy¶
Bluetooth low energy sample applications use the following task priority structure. A higher task number corresponds to a higher priority task:
- Priority 5: Bluetooth low energy protocol stack task (must be highest priority)
- Priority 2: NPI task (network processor configurations only)
- Priority 1: Application task (e.g., simple_peripheral)
In addition, Software Interrupts (SWIs) are used for RF Driver operations. Additional tasks may be inroducted but the relative priority structure above must be preserved.
TI-RTOS Overview introduces TI-RTOS tasks. Overview describes interfacing with the Bluetooth low energy protocol stack. Pre-main initialization describes the application task.
Working With Hex and Binary Files¶
BLE5-Stack projects within this SDK are configured to produce an Intel-extended hex file in their respective output folders. These hex files can be programmed individually with a compatible flash programming tool, such as UniFlash. To simplify the flash programming process, you can combine the application with other Intel-extended hex files into a super hex file manually or using freely available tools provided the individual hex files lack overlapping memory regions. Information on the Intel Hex standard.
One method for creating the super hex file is with the IntelHex python script hex_merge.py, available at the IntelHEX Canonical page. To merge the hex files, install Python® 2.7.x and add it to your system path environment variables.
Warning
Note that when using any python script, you must use a compatible version of Python. Refer to the tool documentation or contact the developer to verify compatibility.
If conversion of the super hex to a binary file is desired, this can be accomplished with the “hex2bin.py” or similar tools that support the hex standard.
1 2 3 | C:\Python27\Scripts>python hex2bin.py \
simple_peripheral_super.hex \
simple_peripheral_super.bin
|
Programming Internal Flash with the ROM Bootloader¶
The CC26x2 internal flash memory can be programmed using the bootloader in the ROM of the device. Both UART and SPI protocols are supported. For more details on the programming protocol and requirements, see the Bootloader chapter of the CC26x2 Technical Reference Manual.
Note
Because the ROM bootloader uses predefined DIO pins for internal flash programming, allocate these pins in the layout of your board. For details on the pins allocated to the bootloader based on the chip package type, see CC26x2 Technical Reference Manual.
Resetting the CC26x2 via software¶
Use only system (hard) resets to reset the device from software. From software, a reset can occur through one of the following.
HCI_EXT_ResetSystemCmd(HCI_EXT_RESET_SYSTEM_HARD);
HAL_SYSTEM_RESET();