Optimizations¶
While debugging, turn off or lower optimizations to ease single-stepping through code. Optimization level can be configured at the project, file and function levels.
Project-Wide Optimizations¶
For debugging, ideally, the project-wide settings for optimization should be as low as possible. There may not be enough available space on the target to do this, but lowering just a couple of levels can be helpful.
In IAR
Project Options –> C/C++ Compiler –> Optimizations
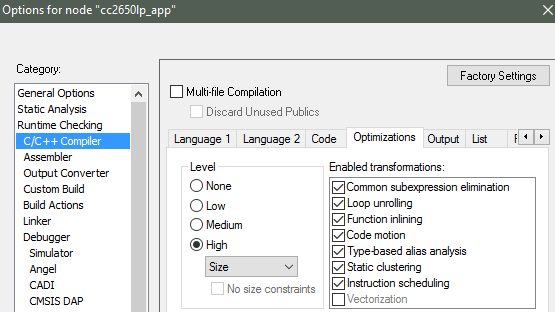
Figure 120. Project-level optimization setting in IAR
In CCS
Project Properties –> CCS Build –> ARM Compiler –> Optimization
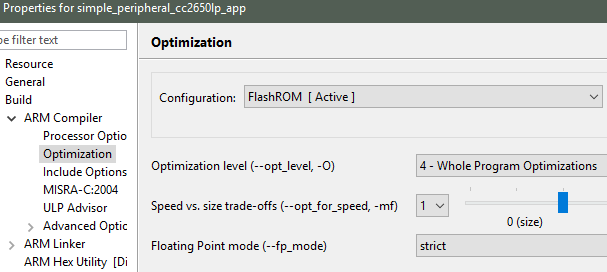
Figure 121. Project-level optimization setting in CCS
Single-File Optimizations¶
Note
Do single-file optimizations with care because this also overrides the project-wide preprocessor symbols.
In IAR
- Right-click on the file in the Workspace pane.
- Choose Options.
- Check Override inherited Settings.
- Choose the optimization level.
In CCS
- Right-click on the file in the Workspace pane.
- Choose Properties.
- Change the optimization level of the file using the same menu in the CCS project-wide optimization menu.
Single-Function Optimizations¶
Using compiler directives, you can control the optimization level of a single function.
In IAR
Use #pragma optimize=none before the function definition to deoptimize the entire function, that is, as follows.
#pragma optimize=none
static void myFunction(int number)
{
// ...
return yourFunction(other_number);
}
In CCS
#pragma FUNCTION_OPTIONS(myFunction, "--opt_level=0")
static void myFunction(int number)
{
// ...
return yourFunction(other_number);
}
Loading TI-RTOS in ROM Symbols¶
Some of the TI-RTOS kernel modules are included in ROM, and executed from ROM in order to save Flash space for the application. This can lead to some confusion, when only addresses are shown in the disassembly view and the call-stack view.
All TI-RTOS kernel code in ROM starts with address 0x1001xxxx
. In order to
make sense of the ROM’ed code, you need to include the symbol files in your
debug session.
In IAR
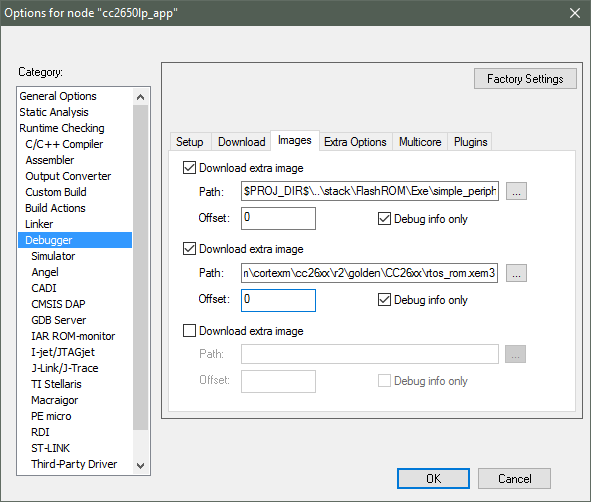
Figure 122. Adding symbol information for the BIOS kernel in ROM
- In the project options, go to
Debugger
andImages
, then add the image <SDK_INSTALL_DIR>\kernel\tirtos\packages\ti\sysbios\rom\cortexm\cc26xx\r2\golden\CC26xx\rtos_rom.xem3
- Check the box for ‘Debug info only’, and use Offset = 0.
In CCS
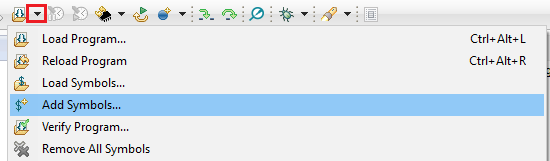
Figure 123. Adding symbol information for the BIOS kernel in ROM, via Add Symbols.
- While in debug mode, click the drop-down button next to the Load Program icon
- Select Add Symbols..
- Select Browse.. and find
<SDK_INSTALL_DIR>\kernel\tirtos\packages\ti\sysbios\rom\cortexm\cc26xx\r2\golden\CC26xx\rtos_rom.xem3