8.3. Run-Time Initialization¶
After the load image is in place, the program can run. The subsections that follow describe bootstrap initialization of a C/C++ program. An assembly-only program may not need to perform all of these steps.
8.3.1. The _c_int00 Function¶
The function _c_int00 is the startup routine (also called the boot routine) for C/C++ programs. It performs all the steps necessary for a C/C++ program to initialize itself.
The name _c_int00 means that it is the interrupt handler for interrupt number 0, RESET, and that it sets up the C environment. Its name need not be exactly _c_int00, but the linker sets _c_int00 as the entry point for C programs by default. The compiler’s run-time-support library provides a default implementation of _c_int00.
The startup routine is responsible for performing the following actions:
Switch to user mode and sets up the user mode stack
Set up status and configuration registers
Set up the stack
Process special binit copy table, if present.
Process the run-time initialization table to autoinitialize global variables (when using the --rom_model option)
Call all global constructors
Call the function main
Call exit when main returns
8.3.2. RAM Model vs. ROM Model¶
Choose a startup model based on the needs of your application. The ROM model performs more work during the boot routine. The RAM model performs more work while loading the application.
If your application is likely to need frequent RESETs or is a standalone application, the ROM model may be a better choice, because the boot routine has all the data it needs to initialize RAM variables. However, for a system with an operating system, it may be better to use the RAM model.
In the EABI ROM model, the C boot routine copies data from the .cinit section to the run-time location of the variables to be initialized.
In the EABI RAM model, no .cinit records are generated at startup.
Note that no default startup model is specified to the linker when the tiarmclang compiler runs the linker. Therefore, either the --rom_model
(-c
) or --ram_model
(-cr
) option must be passed to the linker on the tiarmclang command line or in the linker command file. For example:
tiarmclang -mcpu=cortex-m4 hello.c -o hello.out -Wl,-c,-llnk.cmd,-mhello.map
If neither the -c
or -cr
option is specified to tiarmclang when running the linker, the linker expects an entry point for the linked application to be identified (using the -e=<symbol>
linker option). If -c
or -cr
is specified, then the linker assumes that the program entry point is _c_int00, which performs any needed auto-initialization and system setup, then calls the user’s main() function.
8.3.2.1. Autoinitializing Variables at Run Time (--rom_model)¶
Autoinitializing variables at run time is the most common method of autoinitialization. To use this method, invoke the linker with the --rom_model
option.
The ROM model allows initialization data to be stored in slow non-volatile memory and copied to fast memory each time the program is reset. Use this method if your application runs from code burned into slow memory or needs to survive a reset.
For the ROM model, the .cinit section is loaded into memory along with all the other initialized sections. The linker defines a special symbol called __TI_CINIT_Base that points to the beginning of the initialization tables in memory. When the program begins running, the C boot routine copies data from the tables (pointed to by .cinit) into the run-time location of the variables.
The following figure illustrates autoinitialization at run time using the ROM model.
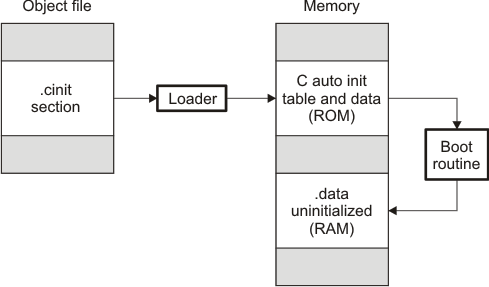
Figure 8.3 Autoinitialization at Run Time¶
8.3.2.2. Initializing Variables at Load Time (--ram_model)¶
The RAM model Initializes variables at load time. To use this method, invoke the linker with the --ram_model option.
This model may reduce boot time and save memory used by the initialization tables.
When you use the --ram_model linker option, the linker sets the STYP_COPY bit in the .cinit section’s header. This tells the loader not to load the .cinit section into memory. (The .cinit section occupies no space in the memory map.)
The linker sets __TI_CINIT_Base equal to __TI_CINIT_Limit to indicate there are no .cinit records.
The loader copies values directly from the .data section to memory.
The following figure illustrates the initialization of variables at load time.
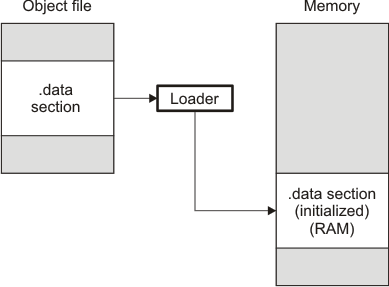
Figure 8.4 Initialization at Load Time¶
8.3.2.3. The --rom_model and --ram_model Linker Options¶
The following list outlines what happens when you invoke the linker with the --ram_model or --rom_model option.
The symbol _c_int00 is defined as the program entry point. The _c_int00 symbol is the start of the C boot routine in
boot.c.o
. Referencing _c_int00 ensures thatboot.c.o
is automatically linked in from the appropriate run-time-support library.If you use the ROM model to autoinitialize at run time (--rom_model option), the linker defines a special symbol, __TI_CINIT_Base, to point to the beginning of the initialization tables in memory. When the program begins running, the C boot routine copies data from the tables (pointed to by .cinit) into the run-time location of the variables.
If you use the RAM model to initialize at load time (--ram_model option), the linker sets __TI_CINIT_Base equal to __TI_CINIT_Limit to indicate there are no .cinit records.
8.3.3. About Linker-Generated Copy Tables¶
The RTS function copy_in can be used at run-time to move code and data around, usually from its load address to its run address. This function reads size and location information from copy tables. The linker automatically generates several kinds of copy tables. Refer to Using Linker-Generated Copy Tables.
You can create and control code overlays with copy tables. See Generating Copy Tables With the table() Operator for details and examples.
Copy tables can be used by the linker to implement run-time relocations as described in Run-Time Relocation, however copy tables require a specific table format.
8.3.3.1. BINIT¶
The BINIT (boot-time initialization) copy table is special in that the target automatically performs the copying at auto-initialization time. Refer to Boot-Time Copy Tables for more about the BINIT copy table name. The BINIT copy table is copied before .cinit processing.
8.3.3.2. CINIT¶
EABI .cinit tables are special kinds of copy tables. Refer to Autoinitializing Variables at Run Time (--rom_model) for more about using the .cinit section with the ROM model and Initializing Variables at Load Time (--ram_model) for more using it with the RAM model.