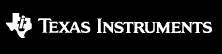 |
 |
Go to the documentation of this file. 47 #define UDMA_STAT 0x400FF000 // DMA Status 48 #define UDMA_CFG 0x400FF004 // DMA Configuration 49 #define UDMA_CTLBASE 0x400FF008 // DMA Channel Control Base Pointer 50 #define UDMA_ALTBASE 0x400FF00C // DMA Alternate Channel Control 52 #define UDMA_WAITSTAT 0x400FF010 // DMA Channel Wait-on-Request 54 #define UDMA_SWREQ 0x400FF014 // DMA Channel Software Request 55 #define UDMA_USEBURSTSET 0x400FF018 // DMA Channel Useburst Set 56 #define UDMA_USEBURSTCLR 0x400FF01C // DMA Channel Useburst Clear 57 #define UDMA_REQMASKSET 0x400FF020 // DMA Channel Request Mask Set 58 #define UDMA_REQMASKCLR 0x400FF024 // DMA Channel Request Mask Clear 59 #define UDMA_ENASET 0x400FF028 // DMA Channel Enable Set 60 #define UDMA_ENACLR 0x400FF02C // DMA Channel Enable Clear 61 #define UDMA_ALTSET 0x400FF030 // DMA Channel Primary Alternate 63 #define UDMA_ALTCLR 0x400FF034 // DMA Channel Primary Alternate 65 #define UDMA_PRIOSET 0x400FF038 // DMA Channel Priority Set 66 #define UDMA_PRIOCLR 0x400FF03C // DMA Channel Priority Clear 67 #define UDMA_ERRCLR 0x400FF04C // DMA Bus Error Clear 68 #define UDMA_CHASGN 0x400FF500 // DMA Channel Assignment 69 #define UDMA_CHMAP0 0x400FF510 // DMA Channel Map Select 0 70 #define UDMA_CHMAP1 0x400FF514 // DMA Channel Map Select 1 71 #define UDMA_CHMAP2 0x400FF518 // DMA Channel Map Select 2 72 #define UDMA_CHMAP3 0x400FF51C // DMA Channel Map Select 3 79 #define UDMA_STAT_DMACHANS_M 0x001F0000 // Available uDMA Channels Minus 1 80 #define UDMA_STAT_STATE_M 0x000000F0 // Control State Machine Status 81 #define UDMA_STAT_STATE_IDLE 0x00000000 // Idle 82 #define UDMA_STAT_STATE_RD_CTRL 0x00000010 // Reading channel controller data 83 #define UDMA_STAT_STATE_RD_SRCENDP \ 84 0x00000020 // Reading source end pointer 85 #define UDMA_STAT_STATE_RD_DSTENDP \ 86 0x00000030 // Reading destination end pointer 87 #define UDMA_STAT_STATE_RD_SRCDAT \ 88 0x00000040 // Reading source data 89 #define UDMA_STAT_STATE_WR_DSTDAT \ 90 0x00000050 // Writing destination data 91 #define UDMA_STAT_STATE_WAIT 0x00000060 // Waiting for uDMA request to 93 #define UDMA_STAT_STATE_WR_CTRL 0x00000070 // Writing channel controller data 94 #define UDMA_STAT_STATE_STALL 0x00000080 // Stalled 95 #define UDMA_STAT_STATE_DONE 0x00000090 // Done 96 #define UDMA_STAT_STATE_UNDEF 0x000000A0 // Undefined 97 #define UDMA_STAT_MASTEN 0x00000001 // Master Enable Status 98 #define UDMA_STAT_DMACHANS_S 16 105 #define UDMA_CFG_MASTEN 0x00000001 // Controller Master Enable 112 #define UDMA_CTLBASE_ADDR_M 0xFFFFFC00 // Channel Control Base Address 113 #define UDMA_CTLBASE_ADDR_S 10 120 #define UDMA_ALTBASE_ADDR_M 0xFFFFFFFF // Alternate Channel Address 122 #define UDMA_ALTBASE_ADDR_S 0 129 #define UDMA_WAITSTAT_WAITREQ_M 0xFFFFFFFF // Channel [n] Wait Status 136 #define UDMA_SWREQ_M 0xFFFFFFFF // Channel [n] Software Request 144 #define UDMA_USEBURSTSET_SET_M 0xFFFFFFFF // Channel [n] Useburst Set 152 #define UDMA_USEBURSTCLR_CLR_M 0xFFFFFFFF // Channel [n] Useburst Clear 160 #define UDMA_REQMASKSET_SET_M 0xFFFFFFFF // Channel [n] Request Mask Set 168 #define UDMA_REQMASKCLR_CLR_M 0xFFFFFFFF // Channel [n] Request Mask Clear 175 #define UDMA_ENASET_SET_M 0xFFFFFFFF // Channel [n] Enable Set 182 #define UDMA_ENACLR_CLR_M 0xFFFFFFFF // Clear Channel [n] Enable Clear 189 #define UDMA_ALTSET_SET_M 0xFFFFFFFF // Channel [n] Alternate Set 196 #define UDMA_ALTCLR_CLR_M 0xFFFFFFFF // Channel [n] Alternate Clear 203 #define UDMA_PRIOSET_SET_M 0xFFFFFFFF // Channel [n] Priority Set 210 #define UDMA_PRIOCLR_CLR_M 0xFFFFFFFF // Channel [n] Priority Clear 217 #define UDMA_ERRCLR_ERRCLR 0x00000001 // uDMA Bus Error Status 224 #define UDMA_CHASGN_M 0xFFFFFFFF // Channel [n] Assignment Select 225 #define UDMA_CHASGN_PRIMARY 0x00000000 // Use the primary channel 227 #define UDMA_CHASGN_SECONDARY 0x00000001 // Use the secondary channel 235 #define UDMA_CHIS_M 0xFFFFFFFF // Channel [n] Interrupt Status 242 #define UDMA_CHMAP0_CH7SEL_M 0xF0000000 // uDMA Channel 7 Source Select 243 #define UDMA_CHMAP0_CH6SEL_M 0x0F000000 // uDMA Channel 6 Source Select 244 #define UDMA_CHMAP0_CH5SEL_M 0x00F00000 // uDMA Channel 5 Source Select 245 #define UDMA_CHMAP0_CH4SEL_M 0x000F0000 // uDMA Channel 4 Source Select 246 #define UDMA_CHMAP0_CH3SEL_M 0x0000F000 // uDMA Channel 3 Source Select 247 #define UDMA_CHMAP0_CH2SEL_M 0x00000F00 // uDMA Channel 2 Source Select 248 #define UDMA_CHMAP0_CH1SEL_M 0x000000F0 // uDMA Channel 1 Source Select 249 #define UDMA_CHMAP0_CH0SEL_M 0x0000000F // uDMA Channel 0 Source Select 250 #define UDMA_CHMAP0_CH7SEL_S 28 251 #define UDMA_CHMAP0_CH6SEL_S 24 252 #define UDMA_CHMAP0_CH5SEL_S 20 253 #define UDMA_CHMAP0_CH4SEL_S 16 254 #define UDMA_CHMAP0_CH3SEL_S 12 255 #define UDMA_CHMAP0_CH2SEL_S 8 256 #define UDMA_CHMAP0_CH1SEL_S 4 257 #define UDMA_CHMAP0_CH0SEL_S 0 264 #define UDMA_CHMAP1_CH15SEL_M 0xF0000000 // uDMA Channel 15 Source Select 265 #define UDMA_CHMAP1_CH14SEL_M 0x0F000000 // uDMA Channel 14 Source Select 266 #define UDMA_CHMAP1_CH13SEL_M 0x00F00000 // uDMA Channel 13 Source Select 267 #define UDMA_CHMAP1_CH12SEL_M 0x000F0000 // uDMA Channel 12 Source Select 268 #define UDMA_CHMAP1_CH11SEL_M 0x0000F000 // uDMA Channel 11 Source Select 269 #define UDMA_CHMAP1_CH10SEL_M 0x00000F00 // uDMA Channel 10 Source Select 270 #define UDMA_CHMAP1_CH9SEL_M 0x000000F0 // uDMA Channel 9 Source Select 271 #define UDMA_CHMAP1_CH8SEL_M 0x0000000F // uDMA Channel 8 Source Select 272 #define UDMA_CHMAP1_CH15SEL_S 28 273 #define UDMA_CHMAP1_CH14SEL_S 24 274 #define UDMA_CHMAP1_CH13SEL_S 20 275 #define UDMA_CHMAP1_CH12SEL_S 16 276 #define UDMA_CHMAP1_CH11SEL_S 12 277 #define UDMA_CHMAP1_CH10SEL_S 8 278 #define UDMA_CHMAP1_CH9SEL_S 4 279 #define UDMA_CHMAP1_CH8SEL_S 0 286 #define UDMA_CHMAP2_CH23SEL_M 0xF0000000 // uDMA Channel 23 Source Select 287 #define UDMA_CHMAP2_CH22SEL_M 0x0F000000 // uDMA Channel 22 Source Select 288 #define UDMA_CHMAP2_CH21SEL_M 0x00F00000 // uDMA Channel 21 Source Select 289 #define UDMA_CHMAP2_CH20SEL_M 0x000F0000 // uDMA Channel 20 Source Select 290 #define UDMA_CHMAP2_CH19SEL_M 0x0000F000 // uDMA Channel 19 Source Select 291 #define UDMA_CHMAP2_CH18SEL_M 0x00000F00 // uDMA Channel 18 Source Select 292 #define UDMA_CHMAP2_CH17SEL_M 0x000000F0 // uDMA Channel 17 Source Select 293 #define UDMA_CHMAP2_CH16SEL_M 0x0000000F // uDMA Channel 16 Source Select 294 #define UDMA_CHMAP2_CH23SEL_S 28 295 #define UDMA_CHMAP2_CH22SEL_S 24 296 #define UDMA_CHMAP2_CH21SEL_S 20 297 #define UDMA_CHMAP2_CH20SEL_S 16 298 #define UDMA_CHMAP2_CH19SEL_S 12 299 #define UDMA_CHMAP2_CH18SEL_S 8 300 #define UDMA_CHMAP2_CH17SEL_S 4 301 #define UDMA_CHMAP2_CH16SEL_S 0 308 #define UDMA_CHMAP3_CH31SEL_M 0xF0000000 // uDMA Channel 31 Source Select 309 #define UDMA_CHMAP3_CH30SEL_M 0x0F000000 // uDMA Channel 30 Source Select 310 #define UDMA_CHMAP3_CH29SEL_M 0x00F00000 // uDMA Channel 29 Source Select 311 #define UDMA_CHMAP3_CH28SEL_M 0x000F0000 // uDMA Channel 28 Source Select 312 #define UDMA_CHMAP3_CH27SEL_M 0x0000F000 // uDMA Channel 27 Source Select 313 #define UDMA_CHMAP3_CH26SEL_M 0x00000F00 // uDMA Channel 26 Source Select 314 #define UDMA_CHMAP3_CH25SEL_M 0x000000F0 // uDMA Channel 25 Source Select 315 #define UDMA_CHMAP3_CH24SEL_M 0x0000000F // uDMA Channel 24 Source Select 316 #define UDMA_CHMAP3_CH31SEL_S 28 317 #define UDMA_CHMAP3_CH30SEL_S 24 318 #define UDMA_CHMAP3_CH29SEL_S 20 319 #define UDMA_CHMAP3_CH28SEL_S 16 320 #define UDMA_CHMAP3_CH27SEL_S 12 321 #define UDMA_CHMAP3_CH26SEL_S 8 322 #define UDMA_CHMAP3_CH25SEL_S 4 323 #define UDMA_CHMAP3_CH24SEL_S 0 330 #define UDMA_O_SRCENDP 0x00000000 // DMA Channel Source Address End 332 #define UDMA_O_DSTENDP 0x00000004 // DMA Channel Destination Address 334 #define UDMA_O_CHCTL 0x00000008 // DMA Channel Control Word 341 #define UDMA_SRCENDP_ADDR_M 0xFFFFFFFF // Source Address End Pointer 342 #define UDMA_SRCENDP_ADDR_S 0 349 #define UDMA_DSTENDP_ADDR_M 0xFFFFFFFF // Destination Address End Pointer 350 #define UDMA_DSTENDP_ADDR_S 0 357 #define UDMA_CHCTL_DSTINC_M 0xC0000000 // Destination Address Increment 358 #define UDMA_CHCTL_DSTINC_8 0x00000000 // Byte 359 #define UDMA_CHCTL_DSTINC_16 0x40000000 // Half-word 360 #define UDMA_CHCTL_DSTINC_32 0x80000000 // Word 361 #define UDMA_CHCTL_DSTINC_NONE 0xC0000000 // No increment 362 #define UDMA_CHCTL_DSTSIZE_M 0x30000000 // Destination Data Size 363 #define UDMA_CHCTL_DSTSIZE_8 0x00000000 // Byte 364 #define UDMA_CHCTL_DSTSIZE_16 0x10000000 // Half-word 365 #define UDMA_CHCTL_DSTSIZE_32 0x20000000 // Word 366 #define UDMA_CHCTL_SRCINC_M 0x0C000000 // Source Address Increment 367 #define UDMA_CHCTL_SRCINC_8 0x00000000 // Byte 368 #define UDMA_CHCTL_SRCINC_16 0x04000000 // Half-word 369 #define UDMA_CHCTL_SRCINC_32 0x08000000 // Word 370 #define UDMA_CHCTL_SRCINC_NONE 0x0C000000 // No increment 371 #define UDMA_CHCTL_SRCSIZE_M 0x03000000 // Source Data Size 372 #define UDMA_CHCTL_SRCSIZE_8 0x00000000 // Byte 373 #define UDMA_CHCTL_SRCSIZE_16 0x01000000 // Half-word 374 #define UDMA_CHCTL_SRCSIZE_32 0x02000000 // Word 375 #define UDMA_CHCTL_DSTPROT0 0x00200000 // Destination Privilege Access 376 #define UDMA_CHCTL_SRCPROT0 0x00040000 // Source Privilege Access 377 #define UDMA_CHCTL_ARBSIZE_M 0x0003C000 // Arbitration Size 378 #define UDMA_CHCTL_ARBSIZE_1 0x00000000 // 1 Transfer 379 #define UDMA_CHCTL_ARBSIZE_2 0x00004000 // 2 Transfers 380 #define UDMA_CHCTL_ARBSIZE_4 0x00008000 // 4 Transfers 381 #define UDMA_CHCTL_ARBSIZE_8 0x0000C000 // 8 Transfers 382 #define UDMA_CHCTL_ARBSIZE_16 0x00010000 // 16 Transfers 383 #define UDMA_CHCTL_ARBSIZE_32 0x00014000 // 32 Transfers 384 #define UDMA_CHCTL_ARBSIZE_64 0x00018000 // 64 Transfers 385 #define UDMA_CHCTL_ARBSIZE_128 0x0001C000 // 128 Transfers 386 #define UDMA_CHCTL_ARBSIZE_256 0x00020000 // 256 Transfers 387 #define UDMA_CHCTL_ARBSIZE_512 0x00024000 // 512 Transfers 388 #define UDMA_CHCTL_ARBSIZE_1024 0x00028000 // 1024 Transfers 389 #define UDMA_CHCTL_XFERSIZE_M 0x00003FF0 // Transfer Size (minus 1) 390 #define UDMA_CHCTL_NXTUSEBURST 0x00000008 // Next Useburst 391 #define UDMA_CHCTL_XFERMODE_M 0x00000007 // uDMA Transfer Mode 392 #define UDMA_CHCTL_XFERMODE_STOP \ 394 #define UDMA_CHCTL_XFERMODE_BASIC \ 396 #define UDMA_CHCTL_XFERMODE_AUTO \ 397 0x00000002 // Auto-Request 398 #define UDMA_CHCTL_XFERMODE_PINGPONG \ 399 0x00000003 // Ping-Pong 400 #define UDMA_CHCTL_XFERMODE_MEM_SG \ 401 0x00000004 // Memory Scatter-Gather 402 #define UDMA_CHCTL_XFERMODE_MEM_SGA \ 403 0x00000005 // Alternate Memory Scatter-Gather 404 #define UDMA_CHCTL_XFERMODE_PER_SG \ 405 0x00000006 // Peripheral Scatter-Gather 406 #define UDMA_CHCTL_XFERMODE_PER_SGA \ 407 0x00000007 // Alternate Peripheral 409 #define UDMA_CHCTL_XFERSIZE_S 4 411 #endif // __HW_UDMA_H__
Copyright 2018, Texas Instruments Incorporated