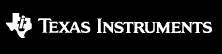 |
 |
Go to the documentation of this file. 38 #ifndef __HW_TIMER_H__ 39 #define __HW_TIMER_H__ 46 #define TIMER_O_CFG 0x00000000 // GPTM Configuration 47 #define TIMER_O_TAMR 0x00000004 // GPTM Timer A Mode 48 #define TIMER_O_TBMR 0x00000008 // GPTM Timer B Mode 49 #define TIMER_O_CTL 0x0000000C // GPTM Control 50 #define TIMER_O_SYNC 0x00000010 // GPTM Synchronize 51 #define TIMER_O_IMR 0x00000018 // GPTM Interrupt Mask 52 #define TIMER_O_RIS 0x0000001C // GPTM Raw Interrupt Status 53 #define TIMER_O_MIS 0x00000020 // GPTM Masked Interrupt Status 54 #define TIMER_O_ICR 0x00000024 // GPTM Interrupt Clear 55 #define TIMER_O_TAILR 0x00000028 // GPTM Timer A Interval Load 56 #define TIMER_O_TBILR 0x0000002C // GPTM Timer B Interval Load 57 #define TIMER_O_TAMATCHR 0x00000030 // GPTM Timer A Match 58 #define TIMER_O_TBMATCHR 0x00000034 // GPTM Timer B Match 59 #define TIMER_O_TAPR 0x00000038 // GPTM Timer A Prescale 60 #define TIMER_O_TBPR 0x0000003C // GPTM Timer B Prescale 61 #define TIMER_O_TAPMR 0x00000040 // GPTM TimerA Prescale Match 62 #define TIMER_O_TBPMR 0x00000044 // GPTM TimerB Prescale Match 63 #define TIMER_O_TAR 0x00000048 // GPTM Timer A 64 #define TIMER_O_TBR 0x0000004C // GPTM Timer B 65 #define TIMER_O_TAV 0x00000050 // GPTM Timer A Value 66 #define TIMER_O_TBV 0x00000054 // GPTM Timer B Value 67 #define TIMER_O_RTCPD 0x00000058 // GPTM RTC Predivide 68 #define TIMER_O_TAPS 0x0000005C // GPTM Timer A Prescale Snapshot 69 #define TIMER_O_TBPS 0x00000060 // GPTM Timer B Prescale Snapshot 70 #define TIMER_O_TAPV 0x00000064 // GPTM Timer A Prescale Value 71 #define TIMER_O_TBPV 0x00000068 // GPTM Timer B Prescale Value 72 #define TIMER_O_DMAEV 0x0000006C // GPTM DMA Event 73 #define TIMER_O_ADCEV 0x00000070 // GPTM ADC Event 74 #define TIMER_O_PP 0x00000FC0 // GPTM Peripheral Properties 75 #define TIMER_O_CC 0x00000FC8 // GPTM Clock Configuration 82 #define TIMER_CFG_M 0x00000007 // GPTM Configuration 83 #define TIMER_CFG_32_BIT_TIMER 0x00000000 // For a 16/32-bit timer, this 86 #define TIMER_CFG_32_BIT_RTC 0x00000001 // For a 16/32-bit timer, this 90 #define TIMER_CFG_16_BIT 0x00000004 // For a 16/32-bit timer, this 99 #define TIMER_TAMR_TCACT_M 0x0000E000 // Timer Compare Action Select 100 #define TIMER_TAMR_TCACT_NONE 0x00000000 // Disable compare operations 101 #define TIMER_TAMR_TCACT_TOGGLE 0x00002000 // Toggle State on Time-Out 102 #define TIMER_TAMR_TCACT_CLRTO 0x00004000 // Clear CCP on Time-Out 103 #define TIMER_TAMR_TCACT_SETTO 0x00006000 // Set CCP on Time-Out 104 #define TIMER_TAMR_TCACT_SETTOGTO \ 105 0x00008000 // Set CCP immediately and toggle 107 #define TIMER_TAMR_TCACT_CLRTOGTO \ 108 0x0000A000 // Clear CCP immediately and toggle 110 #define TIMER_TAMR_TCACT_SETCLRTO \ 111 0x0000C000 // Set CCP immediately and clear on 113 #define TIMER_TAMR_TCACT_CLRSETTO \ 114 0x0000E000 // Clear CCP immediately and set on 116 #define TIMER_TAMR_TACINTD 0x00001000 // One-shot/Periodic Interrupt 118 #define TIMER_TAMR_TAPLO 0x00000800 // GPTM Timer A PWM Legacy 120 #define TIMER_TAMR_TAMRSU 0x00000400 // GPTM Timer A Match Register 122 #define TIMER_TAMR_TAPWMIE 0x00000200 // GPTM Timer A PWM Interrupt 124 #define TIMER_TAMR_TAILD 0x00000100 // GPTM Timer A Interval Load Write 125 #define TIMER_TAMR_TASNAPS 0x00000080 // GPTM Timer A Snap-Shot Mode 126 #define TIMER_TAMR_TAWOT 0x00000040 // GPTM Timer A Wait-on-Trigger 127 #define TIMER_TAMR_TAMIE 0x00000020 // GPTM Timer A Match Interrupt 129 #define TIMER_TAMR_TACDIR 0x00000010 // GPTM Timer A Count Direction 130 #define TIMER_TAMR_TAAMS 0x00000008 // GPTM Timer A Alternate Mode 132 #define TIMER_TAMR_TACMR 0x00000004 // GPTM Timer A Capture Mode 133 #define TIMER_TAMR_TAMR_M 0x00000003 // GPTM Timer A Mode 134 #define TIMER_TAMR_TAMR_1_SHOT 0x00000001 // One-Shot Timer mode 135 #define TIMER_TAMR_TAMR_PERIOD 0x00000002 // Periodic Timer mode 136 #define TIMER_TAMR_TAMR_CAP 0x00000003 // Capture mode 143 #define TIMER_TBMR_TCACT_M 0x0000E000 // Timer Compare Action Select 144 #define TIMER_TBMR_TCACT_NONE 0x00000000 // Disable compare operations 145 #define TIMER_TBMR_TCACT_TOGGLE 0x00002000 // Toggle State on Time-Out 146 #define TIMER_TBMR_TCACT_CLRTO 0x00004000 // Clear CCP on Time-Out 147 #define TIMER_TBMR_TCACT_SETTO 0x00006000 // Set CCP on Time-Out 148 #define TIMER_TBMR_TCACT_SETTOGTO \ 149 0x00008000 // Set CCP immediately and toggle 151 #define TIMER_TBMR_TCACT_CLRTOGTO \ 152 0x0000A000 // Clear CCP immediately and toggle 154 #define TIMER_TBMR_TCACT_SETCLRTO \ 155 0x0000C000 // Set CCP immediately and clear on 157 #define TIMER_TBMR_TCACT_CLRSETTO \ 158 0x0000E000 // Clear CCP immediately and set on 160 #define TIMER_TBMR_TBCINTD 0x00001000 // One-Shot/Periodic Interrupt 162 #define TIMER_TBMR_TBPLO 0x00000800 // GPTM Timer B PWM Legacy 164 #define TIMER_TBMR_TBMRSU 0x00000400 // GPTM Timer B Match Register 166 #define TIMER_TBMR_TBPWMIE 0x00000200 // GPTM Timer B PWM Interrupt 168 #define TIMER_TBMR_TBILD 0x00000100 // GPTM Timer B Interval Load Write 169 #define TIMER_TBMR_TBSNAPS 0x00000080 // GPTM Timer B Snap-Shot Mode 170 #define TIMER_TBMR_TBWOT 0x00000040 // GPTM Timer B Wait-on-Trigger 171 #define TIMER_TBMR_TBMIE 0x00000020 // GPTM Timer B Match Interrupt 173 #define TIMER_TBMR_TBCDIR 0x00000010 // GPTM Timer B Count Direction 174 #define TIMER_TBMR_TBAMS 0x00000008 // GPTM Timer B Alternate Mode 176 #define TIMER_TBMR_TBCMR 0x00000004 // GPTM Timer B Capture Mode 177 #define TIMER_TBMR_TBMR_M 0x00000003 // GPTM Timer B Mode 178 #define TIMER_TBMR_TBMR_1_SHOT 0x00000001 // One-Shot Timer mode 179 #define TIMER_TBMR_TBMR_PERIOD 0x00000002 // Periodic Timer mode 180 #define TIMER_TBMR_TBMR_CAP 0x00000003 // Capture mode 187 #define TIMER_CTL_TBPWML 0x00004000 // GPTM Timer B PWM Output Level 188 #define TIMER_CTL_TBOTE 0x00002000 // GPTM Timer B Output Trigger 190 #define TIMER_CTL_TBEVENT_M 0x00000C00 // GPTM Timer B Event Mode 191 #define TIMER_CTL_TBEVENT_POS 0x00000000 // Positive edge 192 #define TIMER_CTL_TBEVENT_NEG 0x00000400 // Negative edge 193 #define TIMER_CTL_TBEVENT_BOTH 0x00000C00 // Both edges 194 #define TIMER_CTL_TBSTALL 0x00000200 // GPTM Timer B Stall Enable 195 #define TIMER_CTL_TBEN 0x00000100 // GPTM Timer B Enable 196 #define TIMER_CTL_TAPWML 0x00000040 // GPTM Timer A PWM Output Level 197 #define TIMER_CTL_TAOTE 0x00000020 // GPTM Timer A Output Trigger 199 #define TIMER_CTL_RTCEN 0x00000010 // GPTM RTC Stall Enable 200 #define TIMER_CTL_TAEVENT_M 0x0000000C // GPTM Timer A Event Mode 201 #define TIMER_CTL_TAEVENT_POS 0x00000000 // Positive edge 202 #define TIMER_CTL_TAEVENT_NEG 0x00000004 // Negative edge 203 #define TIMER_CTL_TAEVENT_BOTH 0x0000000C // Both edges 204 #define TIMER_CTL_TASTALL 0x00000002 // GPTM Timer A Stall Enable 205 #define TIMER_CTL_TAEN 0x00000001 // GPTM Timer A Enable 212 #define TIMER_SYNC_SYNCT7_M 0x0000C000 // Synchronize GPTM Timer 7 213 #define TIMER_SYNC_SYNCT7_NONE 0x00000000 // GPT7 is not affected 214 #define TIMER_SYNC_SYNCT7_TA 0x00004000 // A timeout event for Timer A of 216 #define TIMER_SYNC_SYNCT7_TB 0x00008000 // A timeout event for Timer B of 218 #define TIMER_SYNC_SYNCT7_TATB 0x0000C000 // A timeout event for both Timer A 221 #define TIMER_SYNC_SYNCT6_M 0x00003000 // Synchronize GPTM Timer 6 222 #define TIMER_SYNC_SYNCT6_NONE 0x00000000 // GPTM6 is not affected 223 #define TIMER_SYNC_SYNCT6_TA 0x00001000 // A timeout event for Timer A of 225 #define TIMER_SYNC_SYNCT6_TB 0x00002000 // A timeout event for Timer B of 227 #define TIMER_SYNC_SYNCT6_TATB 0x00003000 // A timeout event for both Timer A 230 #define TIMER_SYNC_SYNCT5_M 0x00000C00 // Synchronize GPTM Timer 5 231 #define TIMER_SYNC_SYNCT5_NONE 0x00000000 // GPTM5 is not affected 232 #define TIMER_SYNC_SYNCT5_TA 0x00000400 // A timeout event for Timer A of 234 #define TIMER_SYNC_SYNCT5_TB 0x00000800 // A timeout event for Timer B of 236 #define TIMER_SYNC_SYNCT5_TATB 0x00000C00 // A timeout event for both Timer A 239 #define TIMER_SYNC_SYNCT4_M 0x00000300 // Synchronize GPTM Timer 4 240 #define TIMER_SYNC_SYNCT4_NONE 0x00000000 // GPTM4 is not affected 241 #define TIMER_SYNC_SYNCT4_TA 0x00000100 // A timeout event for Timer A of 243 #define TIMER_SYNC_SYNCT4_TB 0x00000200 // A timeout event for Timer B of 245 #define TIMER_SYNC_SYNCT4_TATB 0x00000300 // A timeout event for both Timer A 248 #define TIMER_SYNC_SYNCT3_M 0x000000C0 // Synchronize GPTM Timer 3 249 #define TIMER_SYNC_SYNCT3_NONE 0x00000000 // GPTM3 is not affected 250 #define TIMER_SYNC_SYNCT3_TA 0x00000040 // A timeout event for Timer A of 252 #define TIMER_SYNC_SYNCT3_TB 0x00000080 // A timeout event for Timer B of 254 #define TIMER_SYNC_SYNCT3_TATB 0x000000C0 // A timeout event for both Timer A 257 #define TIMER_SYNC_SYNCT2_M 0x00000030 // Synchronize GPTM Timer 2 258 #define TIMER_SYNC_SYNCT2_NONE 0x00000000 // GPTM2 is not affected 259 #define TIMER_SYNC_SYNCT2_TA 0x00000010 // A timeout event for Timer A of 261 #define TIMER_SYNC_SYNCT2_TB 0x00000020 // A timeout event for Timer B of 263 #define TIMER_SYNC_SYNCT2_TATB 0x00000030 // A timeout event for both Timer A 266 #define TIMER_SYNC_SYNCT1_M 0x0000000C // Synchronize GPTM Timer 1 267 #define TIMER_SYNC_SYNCT1_NONE 0x00000000 // GPTM1 is not affected 268 #define TIMER_SYNC_SYNCT1_TA 0x00000004 // A timeout event for Timer A of 270 #define TIMER_SYNC_SYNCT1_TB 0x00000008 // A timeout event for Timer B of 272 #define TIMER_SYNC_SYNCT1_TATB 0x0000000C // A timeout event for both Timer A 275 #define TIMER_SYNC_SYNCT0_M 0x00000003 // Synchronize GPTM Timer 0 276 #define TIMER_SYNC_SYNCT0_NONE 0x00000000 // GPTM0 is not affected 277 #define TIMER_SYNC_SYNCT0_TA 0x00000001 // A timeout event for Timer A of 279 #define TIMER_SYNC_SYNCT0_TB 0x00000002 // A timeout event for Timer B of 281 #define TIMER_SYNC_SYNCT0_TATB 0x00000003 // A timeout event for both Timer A 290 #define TIMER_IMR_DMABIM 0x00002000 // GPTM Timer B DMA Done Interrupt 292 #define TIMER_IMR_TBMIM 0x00000800 // GPTM Timer B Match Interrupt 294 #define TIMER_IMR_CBEIM 0x00000400 // GPTM Timer B Capture Mode Event 296 #define TIMER_IMR_CBMIM 0x00000200 // GPTM Timer B Capture Mode Match 298 #define TIMER_IMR_TBTOIM 0x00000100 // GPTM Timer B Time-Out Interrupt 300 #define TIMER_IMR_DMAAIM 0x00000020 // GPTM Timer A DMA Done Interrupt 302 #define TIMER_IMR_TAMIM 0x00000010 // GPTM Timer A Match Interrupt 304 #define TIMER_IMR_RTCIM 0x00000008 // GPTM RTC Interrupt Mask 305 #define TIMER_IMR_CAEIM 0x00000004 // GPTM Timer A Capture Mode Event 307 #define TIMER_IMR_CAMIM 0x00000002 // GPTM Timer A Capture Mode Match 309 #define TIMER_IMR_TATOIM 0x00000001 // GPTM Timer A Time-Out Interrupt 317 #define TIMER_RIS_DMABRIS 0x00002000 // GPTM Timer B DMA Done Raw 319 #define TIMER_RIS_TBMRIS 0x00000800 // GPTM Timer B Match Raw Interrupt 320 #define TIMER_RIS_CBERIS 0x00000400 // GPTM Timer B Capture Mode Event 322 #define TIMER_RIS_CBMRIS 0x00000200 // GPTM Timer B Capture Mode Match 324 #define TIMER_RIS_TBTORIS 0x00000100 // GPTM Timer B Time-Out Raw 326 #define TIMER_RIS_DMAARIS 0x00000020 // GPTM Timer A DMA Done Raw 328 #define TIMER_RIS_TAMRIS 0x00000010 // GPTM Timer A Match Raw Interrupt 329 #define TIMER_RIS_RTCRIS 0x00000008 // GPTM RTC Raw Interrupt 330 #define TIMER_RIS_CAERIS 0x00000004 // GPTM Timer A Capture Mode Event 332 #define TIMER_RIS_CAMRIS 0x00000002 // GPTM Timer A Capture Mode Match 334 #define TIMER_RIS_TATORIS 0x00000001 // GPTM Timer A Time-Out Raw 342 #define TIMER_MIS_DMABMIS 0x00002000 // GPTM Timer B DMA Done Masked 344 #define TIMER_MIS_TBMMIS 0x00000800 // GPTM Timer B Match Masked 346 #define TIMER_MIS_CBEMIS 0x00000400 // GPTM Timer B Capture Mode Event 348 #define TIMER_MIS_CBMMIS 0x00000200 // GPTM Timer B Capture Mode Match 350 #define TIMER_MIS_TBTOMIS 0x00000100 // GPTM Timer B Time-Out Masked 352 #define TIMER_MIS_DMAAMIS 0x00000020 // GPTM Timer A DMA Done Masked 354 #define TIMER_MIS_TAMMIS 0x00000010 // GPTM Timer A Match Masked 356 #define TIMER_MIS_RTCMIS 0x00000008 // GPTM RTC Masked Interrupt 357 #define TIMER_MIS_CAEMIS 0x00000004 // GPTM Timer A Capture Mode Event 359 #define TIMER_MIS_CAMMIS 0x00000002 // GPTM Timer A Capture Mode Match 361 #define TIMER_MIS_TATOMIS 0x00000001 // GPTM Timer A Time-Out Masked 369 #define TIMER_ICR_DMABINT 0x00002000 // GPTM Timer B DMA Done Interrupt 371 #define TIMER_ICR_TBMCINT 0x00000800 // GPTM Timer B Match Interrupt 373 #define TIMER_ICR_CBECINT 0x00000400 // GPTM Timer B Capture Mode Event 375 #define TIMER_ICR_CBMCINT 0x00000200 // GPTM Timer B Capture Mode Match 377 #define TIMER_ICR_TBTOCINT 0x00000100 // GPTM Timer B Time-Out Interrupt 379 #define TIMER_ICR_DMAAINT 0x00000020 // GPTM Timer A DMA Done Interrupt 381 #define TIMER_ICR_TAMCINT 0x00000010 // GPTM Timer A Match Interrupt 383 #define TIMER_ICR_RTCCINT 0x00000008 // GPTM RTC Interrupt Clear 384 #define TIMER_ICR_CAECINT 0x00000004 // GPTM Timer A Capture Mode Event 386 #define TIMER_ICR_CAMCINT 0x00000002 // GPTM Timer A Capture Mode Match 388 #define TIMER_ICR_TATOCINT 0x00000001 // GPTM Timer A Time-Out Raw 396 #define TIMER_TAILR_M 0xFFFFFFFF // GPTM Timer A Interval Load 398 #define TIMER_TAILR_S 0 405 #define TIMER_TBILR_M 0xFFFFFFFF // GPTM Timer B Interval Load 407 #define TIMER_TBILR_S 0 415 #define TIMER_TAMATCHR_TAMR_M 0xFFFFFFFF // GPTM Timer A Match Register 416 #define TIMER_TAMATCHR_TAMR_S 0 424 #define TIMER_TBMATCHR_TBMR_M 0xFFFFFFFF // GPTM Timer B Match Register 425 #define TIMER_TBMATCHR_TBMR_S 0 432 #define TIMER_TAPR_TAPSRH_M 0x0000FF00 // GPTM Timer A Prescale High Byte 433 #define TIMER_TAPR_TAPSR_M 0x000000FF // GPTM Timer A Prescale 434 #define TIMER_TAPR_TAPSRH_S 8 435 #define TIMER_TAPR_TAPSR_S 0 442 #define TIMER_TBPR_TBPSRH_M 0x0000FF00 // GPTM Timer B Prescale High Byte 443 #define TIMER_TBPR_TBPSR_M 0x000000FF // GPTM Timer B Prescale 444 #define TIMER_TBPR_TBPSRH_S 8 445 #define TIMER_TBPR_TBPSR_S 0 452 #define TIMER_TAPMR_TAPSMRH_M 0x0000FF00 // GPTM Timer A Prescale Match High 454 #define TIMER_TAPMR_TAPSMR_M 0x000000FF // GPTM TimerA Prescale Match 455 #define TIMER_TAPMR_TAPSMRH_S 8 456 #define TIMER_TAPMR_TAPSMR_S 0 463 #define TIMER_TBPMR_TBPSMRH_M 0x0000FF00 // GPTM Timer B Prescale Match High 465 #define TIMER_TBPMR_TBPSMR_M 0x000000FF // GPTM TimerB Prescale Match 466 #define TIMER_TBPMR_TBPSMRH_S 8 467 #define TIMER_TBPMR_TBPSMR_S 0 474 #define TIMER_TAR_M 0xFFFFFFFF // GPTM Timer A Register 475 #define TIMER_TAR_S 0 482 #define TIMER_TBR_M 0xFFFFFFFF // GPTM Timer B Register 483 #define TIMER_TBR_S 0 490 #define TIMER_TAV_M 0xFFFFFFFF // GPTM Timer A Value 491 #define TIMER_TAV_S 0 498 #define TIMER_TBV_M 0xFFFFFFFF // GPTM Timer B Value 499 #define TIMER_TBV_S 0 506 #define TIMER_RTCPD_RTCPD_M 0x0000FFFF // RTC Predivide Counter Value 507 #define TIMER_RTCPD_RTCPD_S 0 514 #define TIMER_TAPS_PSS_M 0x0000FFFF // GPTM Timer A Prescaler Snapshot 515 #define TIMER_TAPS_PSS_S 0 522 #define TIMER_TBPS_PSS_M 0x0000FFFF // GPTM Timer A Prescaler Value 523 #define TIMER_TBPS_PSS_S 0 530 #define TIMER_TAPV_PSV_M 0x0000FFFF // GPTM Timer A Prescaler Value 531 #define TIMER_TAPV_PSV_S 0 538 #define TIMER_TBPV_PSV_M 0x0000FFFF // GPTM Timer B Prescaler Value 539 #define TIMER_TBPV_PSV_S 0 546 #define TIMER_DMAEV_TBMDMAEN 0x00000800 // GPTM B Mode Match Event DMA 548 #define TIMER_DMAEV_CBEDMAEN 0x00000400 // GPTM B Capture Event DMA Trigger 550 #define TIMER_DMAEV_CBMDMAEN 0x00000200 // GPTM B Capture Match Event DMA 552 #define TIMER_DMAEV_TBTODMAEN 0x00000100 // GPTM B Time-Out Event DMA 554 #define TIMER_DMAEV_TAMDMAEN 0x00000010 // GPTM A Mode Match Event DMA 556 #define TIMER_DMAEV_RTCDMAEN 0x00000008 // GPTM A RTC Match Event DMA 558 #define TIMER_DMAEV_CAEDMAEN 0x00000004 // GPTM A Capture Event DMA Trigger 560 #define TIMER_DMAEV_CAMDMAEN 0x00000002 // GPTM A Capture Match Event DMA 562 #define TIMER_DMAEV_TATODMAEN 0x00000001 // GPTM A Time-Out Event DMA 570 #define TIMER_ADCEV_TBMADCEN 0x00000800 // GPTM B Mode Match Event ADC 572 #define TIMER_ADCEV_CBEADCEN 0x00000400 // GPTM B Capture Event ADC Trigger 574 #define TIMER_ADCEV_CBMADCEN 0x00000200 // GPTM B Capture Match Event ADC 576 #define TIMER_ADCEV_TBTOADCEN 0x00000100 // GPTM B Time-Out Event ADC 578 #define TIMER_ADCEV_TAMADCEN 0x00000010 // GPTM A Mode Match Event ADC 580 #define TIMER_ADCEV_RTCADCEN 0x00000008 // GPTM RTC Match Event ADC Trigger 582 #define TIMER_ADCEV_CAEADCEN 0x00000004 // GPTM A Capture Event ADC Trigger 584 #define TIMER_ADCEV_CAMADCEN 0x00000002 // GPTM A Capture Match Event ADC 586 #define TIMER_ADCEV_TATOADCEN 0x00000001 // GPTM A Time-Out Event ADC 594 #define TIMER_PP_ALTCLK 0x00000040 // Alternate Clock Source 595 #define TIMER_PP_SYNCCNT 0x00000020 // Synchronize Start 596 #define TIMER_PP_CHAIN 0x00000010 // Chain with Other Timers 597 #define TIMER_PP_SIZE_M 0x0000000F // Count Size 598 #define TIMER_PP_SIZE_16 0x00000000 // Timer A and Timer B counters are 601 #define TIMER_PP_SIZE_32 0x00000001 // Timer A and Timer B counters are 610 #define TIMER_CC_ALTCLK 0x00000001 // Alternate Clock Source 612 #endif // __HW_TIMER_H__
Copyright 2018, Texas Instruments Incorporated