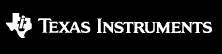 |
 |
Go to the documentation of this file. 46 #define SSI_O_CR0 0x00000000 // SSI Control 0 47 #define SSI_O_CR1 0x00000004 // SSI Control 1 48 #define SSI_O_DR 0x00000008 // SSI Data 49 #define SSI_O_SR 0x0000000C // SSI Status 50 #define SSI_O_CPSR 0x00000010 // SSI Clock Prescale 51 #define SSI_O_IM 0x00000014 // SSI Interrupt Mask 52 #define SSI_O_RIS 0x00000018 // SSI Raw Interrupt Status 53 #define SSI_O_MIS 0x0000001C // SSI Masked Interrupt Status 54 #define SSI_O_ICR 0x00000020 // SSI Interrupt Clear 55 #define SSI_O_DMACTL 0x00000024 // SSI DMA Control 56 #define SSI_O_PP 0x00000FC0 // SSI Peripheral Properties 57 #define SSI_O_CC 0x00000FC8 // SSI Clock Configuration 64 #define SSI_CR0_SCR_M 0x0000FF00 // SSI Serial Clock Rate 65 #define SSI_CR0_SPH 0x00000080 // SSI Serial Clock Phase 66 #define SSI_CR0_SPO 0x00000040 // SSI Serial Clock Polarity 67 #define SSI_CR0_FRF_M 0x00000030 // SSI Frame Format Select 68 #define SSI_CR0_FRF_MOTO 0x00000000 // Freescale SPI Frame Format 69 #define SSI_CR0_FRF_TI 0x00000010 // Synchronous Serial Frame Format 70 #define SSI_CR0_DSS_M 0x0000000F // SSI Data Size Select 71 #define SSI_CR0_DSS_4 0x00000003 // 4-bit data 72 #define SSI_CR0_DSS_5 0x00000004 // 5-bit data 73 #define SSI_CR0_DSS_6 0x00000005 // 6-bit data 74 #define SSI_CR0_DSS_7 0x00000006 // 7-bit data 75 #define SSI_CR0_DSS_8 0x00000007 // 8-bit data 76 #define SSI_CR0_DSS_9 0x00000008 // 9-bit data 77 #define SSI_CR0_DSS_10 0x00000009 // 10-bit data 78 #define SSI_CR0_DSS_11 0x0000000A // 11-bit data 79 #define SSI_CR0_DSS_12 0x0000000B // 12-bit data 80 #define SSI_CR0_DSS_13 0x0000000C // 13-bit data 81 #define SSI_CR0_DSS_14 0x0000000D // 14-bit data 82 #define SSI_CR0_DSS_15 0x0000000E // 15-bit data 83 #define SSI_CR0_DSS_16 0x0000000F // 16-bit data 84 #define SSI_CR0_SCR_S 8 91 #define SSI_CR1_EOM 0x00000800 // Stop Frame (End of Message) 92 #define SSI_CR1_FSSHLDFRM 0x00000400 // FSS Hold Frame 93 #define SSI_CR1_HSCLKEN 0x00000200 // High Speed Clock Enable 94 #define SSI_CR1_DIR 0x00000100 // SSI Direction of Operation 95 #define SSI_CR1_MODE_M 0x000000C0 // SSI Mode 96 #define SSI_CR1_MODE_LEGACY 0x00000000 // Legacy SSI mode 97 #define SSI_CR1_MODE_BI 0x00000040 // Bi-SSI mode 98 #define SSI_CR1_MODE_QUAD 0x00000080 // Quad-SSI Mode 99 #define SSI_CR1_MODE_ADVANCED 0x000000C0 // Advanced SSI Mode with 8-bit 101 #define SSI_CR1_MS 0x00000004 // SSI Master/Slave Select 102 #define SSI_CR1_SSE 0x00000002 // SSI Synchronous Serial Port 104 #define SSI_CR1_LBM 0x00000001 // SSI Loopback Mode 111 #define SSI_DR_DATA_M 0x0000FFFF // SSI Receive/Transmit Data 112 #define SSI_DR_DATA_S 0 119 #define SSI_SR_BSY 0x00000010 // SSI Busy Bit 120 #define SSI_SR_RFF 0x00000008 // SSI Receive FIFO Full 121 #define SSI_SR_RNE 0x00000004 // SSI Receive FIFO Not Empty 122 #define SSI_SR_TNF 0x00000002 // SSI Transmit FIFO Not Full 123 #define SSI_SR_TFE 0x00000001 // SSI Transmit FIFO Empty 130 #define SSI_CPSR_CPSDVSR_M 0x000000FF // SSI Clock Prescale Divisor 131 #define SSI_CPSR_CPSDVSR_S 0 138 #define SSI_IM_EOTIM 0x00000040 // End of Transmit Interrupt Mask 139 #define SSI_IM_DMATXIM 0x00000020 // SSI Transmit DMA Interrupt Mask 140 #define SSI_IM_DMARXIM 0x00000010 // SSI Receive DMA Interrupt Mask 141 #define SSI_IM_TXIM 0x00000008 // SSI Transmit FIFO Interrupt Mask 142 #define SSI_IM_RXIM 0x00000004 // SSI Receive FIFO Interrupt Mask 143 #define SSI_IM_RTIM 0x00000002 // SSI Receive Time-Out Interrupt 145 #define SSI_IM_RORIM 0x00000001 // SSI Receive Overrun Interrupt 153 #define SSI_RIS_EOTRIS 0x00000040 // End of Transmit Raw Interrupt 155 #define SSI_RIS_DMATXRIS 0x00000020 // SSI Transmit DMA Raw Interrupt 157 #define SSI_RIS_DMARXRIS 0x00000010 // SSI Receive DMA Raw Interrupt 159 #define SSI_RIS_TXRIS 0x00000008 // SSI Transmit FIFO Raw Interrupt 161 #define SSI_RIS_RXRIS 0x00000004 // SSI Receive FIFO Raw Interrupt 163 #define SSI_RIS_RTRIS 0x00000002 // SSI Receive Time-Out Raw 165 #define SSI_RIS_RORRIS 0x00000001 // SSI Receive Overrun Raw 173 #define SSI_MIS_EOTMIS 0x00000040 // End of Transmit Masked Interrupt 175 #define SSI_MIS_DMATXMIS 0x00000020 // SSI Transmit DMA Masked 177 #define SSI_MIS_DMARXMIS 0x00000010 // SSI Receive DMA Masked Interrupt 179 #define SSI_MIS_TXMIS 0x00000008 // SSI Transmit FIFO Masked 181 #define SSI_MIS_RXMIS 0x00000004 // SSI Receive FIFO Masked 183 #define SSI_MIS_RTMIS 0x00000002 // SSI Receive Time-Out Masked 185 #define SSI_MIS_RORMIS 0x00000001 // SSI Receive Overrun Masked 193 #define SSI_ICR_EOTIC 0x00000040 // End of Transmit Interrupt Clear 194 #define SSI_ICR_DMATXIC 0x00000020 // SSI Transmit DMA Interrupt Clear 195 #define SSI_ICR_DMARXIC 0x00000010 // SSI Receive DMA Interrupt Clear 196 #define SSI_ICR_RTIC 0x00000002 // SSI Receive Time-Out Interrupt 198 #define SSI_ICR_RORIC 0x00000001 // SSI Receive Overrun Interrupt 206 #define SSI_DMACTL_TXDMAE 0x00000002 // Transmit DMA Enable 207 #define SSI_DMACTL_RXDMAE 0x00000001 // Receive DMA Enable 214 #define SSI_PP_FSSHLDFRM 0x00000008 // FSS Hold Frame Capability 215 #define SSI_PP_MODE_M 0x00000006 // Mode of Operation 216 #define SSI_PP_MODE_LEGACY 0x00000000 // Legacy SSI mode 217 #define SSI_PP_MODE_ADVBI 0x00000002 // Legacy mode, Advanced SSI mode 219 #define SSI_PP_MODE_ADVBIQUAD 0x00000004 // Legacy mode, Advanced mode, 221 #define SSI_PP_HSCLK 0x00000001 // High Speed Capability 228 #define SSI_CC_CS_M 0x0000000F // SSI Baud Clock Source 229 #define SSI_CC_CS_SYSPLL 0x00000000 // System clock (based on clock 231 #define SSI_CC_CS_ALTCLK 0x00000005 // Alternate clock source based on 234 #endif // __HW_SSI_H__
Copyright 2018, Texas Instruments Incorporated