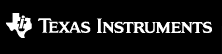 |
 |
Go to the documentation of this file. 46 #define GPIO_O_DATA 0x00000000 // GPIO Data 47 #define GPIO_O_DIR 0x00000400 // GPIO Direction 48 #define GPIO_O_IS 0x00000404 // GPIO Interrupt Sense 49 #define GPIO_O_IBE 0x00000408 // GPIO Interrupt Both Edges 50 #define GPIO_O_IEV 0x0000040C // GPIO Interrupt Event 51 #define GPIO_O_IM 0x00000410 // GPIO Interrupt Mask 52 #define GPIO_O_RIS 0x00000414 // GPIO Raw Interrupt Status 53 #define GPIO_O_MIS 0x00000418 // GPIO Masked Interrupt Status 54 #define GPIO_O_ICR 0x0000041C // GPIO Interrupt Clear 55 #define GPIO_O_AFSEL 0x00000420 // GPIO Alternate Function Select 56 #define GPIO_O_DR2R 0x00000500 // GPIO 2-mA Drive Select 57 #define GPIO_O_DR4R 0x00000504 // GPIO 4-mA Drive Select 58 #define GPIO_O_DR8R 0x00000508 // GPIO 8-mA Drive Select 59 #define GPIO_O_ODR 0x0000050C // GPIO Open Drain Select 60 #define GPIO_O_PUR 0x00000510 // GPIO Pull-Up Select 61 #define GPIO_O_PDR 0x00000514 // GPIO Pull-Down Select 62 #define GPIO_O_SLR 0x00000518 // GPIO Slew Rate Control Select 63 #define GPIO_O_DEN 0x0000051C // GPIO Digital Enable 64 #define GPIO_O_LOCK 0x00000520 // GPIO Lock 65 #define GPIO_O_CR 0x00000524 // GPIO Commit 66 #define GPIO_O_AMSEL 0x00000528 // GPIO Analog Mode Select 67 #define GPIO_O_PCTL 0x0000052C // GPIO Port Control 68 #define GPIO_O_ADCCTL 0x00000530 // GPIO ADC Control 69 #define GPIO_O_DMACTL 0x00000534 // GPIO DMA Control 70 #define GPIO_O_SI 0x00000538 // GPIO Select Interrupt 71 #define GPIO_O_DR12R 0x0000053C // GPIO 12-mA Drive Select 72 #define GPIO_O_WAKEPEN 0x00000540 // GPIO Wake Pin Enable 73 #define GPIO_O_WAKELVL 0x00000544 // GPIO Wake Level 74 #define GPIO_O_WAKESTAT 0x00000548 // GPIO Wake Status 75 #define GPIO_O_PP 0x00000FC0 // GPIO Peripheral Property 76 #define GPIO_O_PC 0x00000FC4 // GPIO Peripheral Configuration 83 #define GPIO_IM_DMAIME 0x00000100 // GPIO uDMA Done Interrupt Mask 85 #define GPIO_IM_GPIO_M 0x000000FF // GPIO Interrupt Mask Enable 86 #define GPIO_IM_GPIO_S 0 93 #define GPIO_RIS_DMARIS 0x00000100 // GPIO uDMA Done Interrupt Raw 95 #define GPIO_RIS_GPIO_M 0x000000FF // GPIO Interrupt Raw Status 96 #define GPIO_RIS_GPIO_S 0 103 #define GPIO_MIS_DMAMIS 0x00000100 // GPIO uDMA Done Masked Interrupt 105 #define GPIO_MIS_GPIO_M 0x000000FF // GPIO Masked Interrupt Status 106 #define GPIO_MIS_GPIO_S 0 113 #define GPIO_ICR_DMAIC 0x00000100 // GPIO uDMA Interrupt Clear 114 #define GPIO_ICR_GPIO_M 0x000000FF // GPIO Interrupt Clear 115 #define GPIO_ICR_GPIO_S 0 122 #define GPIO_LOCK_M 0xFFFFFFFF // GPIO Lock 123 #define GPIO_LOCK_UNLOCKED 0x00000000 // The GPIOCR register is unlocked 125 #define GPIO_LOCK_LOCKED 0x00000001 // The GPIOCR register is locked 127 #define GPIO_LOCK_KEY 0x4C4F434B // Unlocks the GPIO_CR register 134 #define GPIO_SI_SUM 0x00000001 // Summary Interrupt 141 #define GPIO_DR12R_DRV12_M 0x000000FF // Output Pad 12-mA Drive Enable 142 #define GPIO_DR12R_DRV12_12MA 0x00000001 // The corresponding GPIO pin has 154 #define GPIO_WAKEPEN_WAKEP4 0x00000010 // P[4] Wake Enable 161 #define GPIO_WAKELVL_WAKELVL4 0x00000010 // P[4] Wake Level 169 #define GPIO_WAKESTAT_STAT4 0x00000010 // P[4] Wake Status 176 #define GPIO_PP_EDE 0x00000001 // Extended Drive Enable 183 #define GPIO_PC_EDM7_M 0x0000C000 // Extended Drive Mode Bit 7 184 #define GPIO_PC_EDM6_M 0x00003000 // Extended Drive Mode Bit 6 185 #define GPIO_PC_EDM5_M 0x00000C00 // Extended Drive Mode Bit 5 186 #define GPIO_PC_EDM4_M 0x00000300 // Extended Drive Mode Bit 4 187 #define GPIO_PC_EDM3_M 0x000000C0 // Extended Drive Mode Bit 3 188 #define GPIO_PC_EDM2_M 0x00000030 // Extended Drive Mode Bit 2 189 #define GPIO_PC_EDM1_M 0x0000000C // Extended Drive Mode Bit 1 190 #define GPIO_PC_EDM0_M 0x00000003 // Extended Drive Mode Bit 0 191 #define GPIO_PC_EDM0_DISABLE 0x00000000 // Drive values of 2, 4 and 8 mA 195 #define GPIO_PC_EDM0_6MA 0x00000001 // An additional 6 mA option is 197 #define GPIO_PC_EDM0_PLUS2MA 0x00000003 // A 2 mA driver is always enabled; 203 #define GPIO_PC_EDM7_S 14 204 #define GPIO_PC_EDM6_S 12 205 #define GPIO_PC_EDM5_S 10 206 #define GPIO_PC_EDM4_S 8 207 #define GPIO_PC_EDM3_S 6 208 #define GPIO_PC_EDM2_S 4 209 #define GPIO_PC_EDM1_S 2 211 #endif // __HW_GPIO_H__
Copyright 2018, Texas Instruments Incorporated