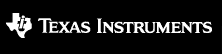 |
 |
Go to the documentation of this file. 36 #define _ANSI_TERM_GET_OVERRIDE(_1, _2, _3, _4, _5, NAME, ...) NAME 38 #define _ANSI_TERM_CONCAT_COLOR5(a, b, c, d, e) \ 39 "\x1b[" _ANSI_TERM_ ## a ";" _ANSI_TERM_ ## b ";" _ANSI_TERM_ ## c \ 40 ";" _ANSI_TERM_ ## d ";" _ANSI_TERM_ ## e "m" 41 #define _ANSI_TERM_CONCAT_COLOR4(a, b, c, d) \ 42 "\x1b[" _ANSI_TERM_ ## a ";" _ANSI_TERM_ ## b ";" _ANSI_TERM_ ## c \ 43 ";" _ANSI_TERM_ ## d "m" 44 #define _ANSI_TERM_CONCAT_COLOR3(a, b, c) \ 45 "\x1b[" _ANSI_TERM_ ## a ";" _ANSI_TERM_ ## b ";" _ANSI_TERM_ ## c "m" 46 #define _ANSI_TERM_CONCAT_COLOR2(a, b) \ 47 "\x1b[" _ANSI_TERM_ ## a ";" _ANSI_TERM_ ## b "m" 48 #define _ANSI_TERM_CONCAT_COLOR1(a) \ 49 "\x1b[" _ANSI_TERM_ ## a "m" 51 #define _ANSI_TERM_FG_BLACK "30" 52 #define _ANSI_TERM_FG_RED "31" 53 #define _ANSI_TERM_FG_GREEN "32" 54 #define _ANSI_TERM_FG_YELLOW "33" 55 #define _ANSI_TERM_FG_BLUE "34" 56 #define _ANSI_TERM_FG_MAGENTA "35" 57 #define _ANSI_TERM_FG_CYAN "36" 58 #define _ANSI_TERM_FG_WHITE "37" 60 #define _ANSI_TERM_BG_BLACK "40" 61 #define _ANSI_TERM_BG_RED "41" 62 #define _ANSI_TERM_BG_GREEN "42" 63 #define _ANSI_TERM_BG_YELLOW "43" 64 #define _ANSI_TERM_BG_BLUE "44" 65 #define _ANSI_TERM_BG_MAGENTA "45" 66 #define _ANSI_TERM_BG_CYAN "46" 67 #define _ANSI_TERM_BG_WHITE "47" 69 #define _ANSI_TERM_ATTR_RESET "0" 70 #define _ANSI_TERM_ATTR_BOLD "1" 71 #define _ANSI_TERM_ATTR_UNDERLINE "4" 72 #define _ANSI_TERM_ATTR_BLINK "5" 93 #define ANSI_COLOR(...) \ 94 _ANSI_TERM_GET_OVERRIDE(__VA_ARGS__, \ 95 _ANSI_TERM_CONCAT_COLOR5, _ANSI_TERM_CONCAT_COLOR4, _ANSI_TERM_CONCAT_COLOR3, \ 96 _ANSI_TERM_CONCAT_COLOR2, _ANSI_TERM_CONCAT_COLOR1) (__VA_ARGS__)
© Copyright 1995-2019, Texas Instruments Incorporated. All rights reserved.
Trademarks | Privacy policy | Terms of use | Terms of sale