Thread Synchronization¶
The TI-RTOS kernel provides several modules for synchronizing tasks such as Semaphore, Event, and Queue. The following sections discuss these common TI-RTOS primitives.
Semaphores¶
Semaphores are commonly used for task synchronization and mutual exclusions
throughout TI-RTOS applications. Figure 58. shows the semaphore
functionality. Semaphores can be counting semaphores or binary semaphores.
Counting semaphores keep track of the number of times the semaphore is posted
with Semaphore_post()
. When a group of resources are shared between tasks,
this function is useful. Such tasks might call Semaphore_pend()
to see if a
resource is available before using one. Binary semaphores can have only two
states: available (count = 1) and unavailable (count = 0). Binary semaphores
can be used to share a single resource between tasks or for a basic-signaling
mechanism where the semaphore can be posted multiple times. Binary semaphores
do not keep track of the count; they track only whether the semaphore has been
posted.
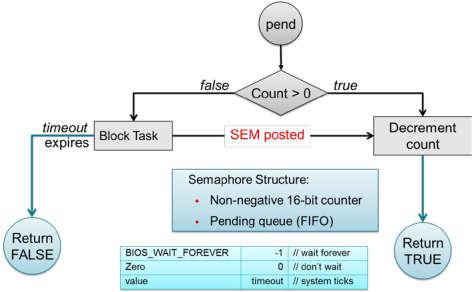
Figure 58. Semaphore Functionality¶
Initializing a Semaphore¶
The following code depicts how a semaphore is initialized in TI-RTOS. Semaphores can be created and constructed as explained in Creating vs. Constructing.
See Listing 2. on how to create a Semaphore.
See Listing 3. on how to construct a Semaphore.
Pending on a Semaphore¶
Semaphore_pend()
is a blocking function call. This call may only be called
from within a Task context. A task calling this function will allow lower
priority tasks to execute, if they are ready to run. A task calling
Semaphore_pend()
will block if its counter is 0, otherwise it will
decrement the counter by one. The task will remain blocked until another thread
calls Semaphore_post()
or if the supplied system tick timeout has occurred;
whichever comes first. By reading the return value of Semaphore_pend()
it
is possible to distinguish if a semaphore was posted or if it timed out.
1bool isSuccessful;
2uint32_t timeout = 1000 * (1000/Clock_tickPeriod);
3
4/* Pend (approximately) up to 1 second */
5isSuccessful = Semaphore_pend(sem, timeoutInTicks);
6
7if (isSuccessful)
8{
9 System_printf("Semaphore was posted");
10}
11else
12{
13 System_printf("Semaphore timed out");
14}
Note
The default TI-RTOS system tick period is 1 millisecond. This default is
reconfigured to 10 microseconds for CC13xx or CC26xx by
setting Clock.tickPeriod = 10
in the .cfg
file.
Given a system tick of 10 microseconds, timeout
in
Listing 4. will be approximately 1 second.
Posting a Semaphore¶
Posting a semaphore is accomplished via a call to Semaphore_post()
. A task
that is pending on a posted semaphore will transition from a blocked state to
a ready state. If no higher priority thread is ready to run, it will allow
the previously pending task to execute. If no task is pending on the semaphore,
a call to Semaphore_post()
will increment its counter. Binary semaphores
have a maximum count of 1.
1Semaphore_post(sem);
Event¶
Semaphores themselves provide rudimentary synchronization between threads. There are cases just the Semaphore itself is enough to understand on what process needs to be triggered. Often however, a specific causes for the synchronization need to be passed across threads as well. To help accomplish this, one can utilize the TI-RTOS Event module.
Events are similar to Semaphores in a sense that each instance of an Event object actually contains a Semaphore. The added advantage of using Events lie in the fact that tasks can be notified of specific events in a thread-safe manner.
Initializing an Event¶
Creating and constructing Events follow the same guidelines as explained in Creating vs. Constructing. Shown in Listing 6. is an example on how to construct an Event instance.
1Event_Handle event;
2Event_Params eventParams;
3Event_Struct structEvent; /* Memory allocated at build time */
4
5Event_Params_init(&eventParams);
6Event_construct(&structEvent, 0, &eventParams);
7
8/* It's optional to store the handle */
9event = Event_handle(&structEvent);
Pending on an Event¶
Similar to Semaphore_pend()
, a Task thread would typically block on an
Event_pend()
until an event is posted via an Event_post()
or if the
specified timeout expired. Shown in Listing 7. is a
snippet of a task pending on any of the 3 sample event IDs shown below.
BIOS_WAIT_FOREVER
is used to prevent a timeout from occurring. As a result,
Event_pend()
will have one or more events posted in the returned bit-masked
value.
Each event returned from Event_pend()
has been automatically cleared within
the event instance in a thread-safe manner. Therefore, it is only necessary to
keep a local copy of posted events. For full details on how to use
Event_pend()
, see the TI-RTOS Kernel (SYS/BIOS) User’s Guide.
1#define START_ADVERTISING_EVT Event_Id_00
2#define START_CONN_UPDATE_EVT Event_Id_01
3#define CONN_PARAM_TIMEOUT_EVT Event_Id_02
4
5void TaskFxn(..)
6{
7 /* Local copy of events that have been posted */
8 uint32_t events;
9
10 while(1)
11 {
12 /* Wait for an event to be posted */
13 events = Event_pend(event,
14 Event_Id_NONE,
15 START_ADVERTISING_EVT |
16 START_CONN_UPDATE_EVT |
17 CONN_PARAM_TIMEOUT_EVT,
18 BIOS_WAIT_FOREVER);
19
20 if (events & START_ADVERTISING_EVT)
21 {
22 /* Process this event */
23 }
24
25 if (events & START_CONN_UPDATE_EVT)
26 {
27 /* Process this event */
28 }
29
30 if (events & CONN_PARAM_TIMEOUT_EVT)
31 {
32 /* Process this event */
33 }
34 }
35}
Note
The default TI-RTOS system tick period is 1 millisecond. This default is
reconfigured to 10 microseconds for CC26xx and CC13xx devices by setting
Clock.tickPeriod = 10
in the .cfg
file.
Given a system tick of 10 microseconds, timeout
in
Listing 4. will be approximately 1 second.
Posting an Event¶
Events may be posted from any TI-RTOS kernel contexts and is simply done by
calling Event_post()
of the Event instance and the Event ID.
Listing 8. shows how a high priority thread such as a Swi
could post a specific event.
1#define START_ADVERTISING_EVT Event_Id_00
2#define START_CONN_UPDATE_EVT Event_Id_01
3#define CONN_PARAM_TIMEOUT_EVT Event_Id_02
4
5void SwiFxn(UArg arg)
6{
7 Event_post(event, START_ADVERTISING_EVT);
8}