Code Examples¶
The code examples in this library shows basic usage of the library API’s. The example project implements the typical SW Flow in ultrasonic application as described in section Flow Diagram
Software Prerequisites¶
- Code Composer Studio version 8.2.0 or later
- Compiler version or TI v18.1.3.LTS
- IAR Embedded Workbench for MSP v 7.11.3 or later
Hardware Prerequisites¶
- EVM430-FR6047 (recommended to evaluate single tone ultrasonic applications)
- EVM430-FR6043 (recommended to evaluate multi tone ultrasonic applications)
- EVM430-FR6043-02 (recommended to evaluate single tone ultrasonic applications)
- MSP-TS430PZ100E
Device Resource Usage¶
The USS library requires access to different MSP430 modules based on the excitation type selected user selection to to control the external AFE.
Module | Default selection | File containing module defintion | Notes |
---|---|---|---|
Timer_A | Timer_A1 | ussSWLib/USS_HAL/USS_Lib_HAL.h | Used as background timer, to generate USS crystal/resonator settling times and to enable transitions to LPM3 during low power captures. |
LEA | Not configurable | Internally used by Algorithms API to accelerate vector based operations | |
Timer_A | Timer_A0 | ussSWLib/USS_HAL/USS_Lib_HAL.h | Use to generate AFE events. |
GPIO USSSWLIB_HAL_AFE_RXEN | P6.7 | ussSWLib/USS_HAL/USS_Lib_HAL.h | Only use when controlling external AFE |
GPIO USSSWLIB_HAL_AFE_RX_SEL1 | P3.6 | ussSWLib/USS_HAL/USS_Lib_HAL.h | Only use when controlling external AFE |
GPIO USSSWLIB_HAL_AFE_RX_SEL2 | P3.7 | ussSWLib/USS_HAL/USS_Lib_HAL.h | Only use when controlling external AFE |
GPIO USSSWLIB_HAL_AFE_RXPWR | P2.2 | ussSWLib/USS_HAL/USS_Lib_HAL.h | Only use when controlling external AFE |
GPIO USSSWLIB_HAL_AFE_TXPWR | P1.0 | ussSWLib/USS_HAL/USS_Lib_HAL.h | Only use when controlling external AFE |
DMA | DMA_Channel 2 | Not configurable | Only needed when using multitoned excitation pulses and running on device with USS_A module |
DMA_Channel 3 | Not configurable | Only needed when using multitoned excitation pulses and running on device with USS_A module |
Library configuration assumptions¶
The following modules are automatically configured by the library and don’t need to be initialized as part of the application:
- USS module
- Timer_A
- GPIOs use to control external circuitry
- DMA channel when multitoned generation is selected
In order to achieve the lowest power consumption and achieve the expected timing configuration, it is recommended that users configure the following modules.
- Clock System (CS)
- It is highly recommended that ACLK is sourced externally by a 32.768 KHz crystal
- Please refer to MSP430™ 32-KHz Crystal Oscillators Application Report for further information for crystal selection
- It is highly recommended that ACLK is sourced externally by a 32.768 KHz crystal
- Digital I/O
- It is highly recommended to terminate unused pins.
For further recommendations to achieve the lowest power consumption please refer to How to achieve the specified power consumption values as in the datasheet?
In addition, the clock module configuration must match LIBRARY CLOCK DEFINITION configuration defines in <USS_SW_LIB_ROOT>/examples/USSSWLib_template_example/USS_Config/USS_userConfig.h
.
Code section :
/*******************************************************************************
* LIBRARY CLOCK DEFINITION
*
* IMPORTANT: The following defines only specify MCLK and LFXT frequencies at
* which the application is configured to run. The library DOES NOT configure
* MCLK, SMCLK or LFXT.
*
* This parameter are also using in the derived parameter section to calculate
* HSPLL counts and LFXT counts.
*
* USS_MCLK_FREQ_IN_HZ valid options:
* - Valid device specific MCLK frequency options
*
* USS_LFXT_FREQ_IN_HZ valid options:
* - Valid device specific MCLK frequency options
*
*******************************************************************************/
#if (USS_PULSE_MODE == USS_PULSE_MODE_MULTI_TONE)
#define USS_MCLK_FREQ_IN_HZ 16000000
#else
#define USS_MCLK_FREQ_IN_HZ 8000000
#endif
#define USS_SMCLK_FREQ_IN_HZ 8000000
#define USS_LFXT_FREQ_IN_HZ 32768
Code Example Directory Structure¶
The USS SW Library contains a basic template example project. The intention of the example is showcase the typical ultrasonic
- CCS - Code composer specific project files this directory contains project configuration files (*_USSSWLib_template_example.projectspec) and linker command files.
- IAR - IAR specific project files and IAR linker command files.
- USS_Config - Default library configuration files. For more information regarding modifying the library configuration file please refer to Modifying USS SW Library Configuration files
Code Composer Studio¶
Importing the Project¶
- In CCS, select File -> Import…
- In the Import window, select C/C++ -> CCS Projects and select the Next button
- In the Import CCS Eclipse Projects window, hit the Browse button to browse to
<USS_SW_LIB_EXAMPLES_DIR>
and then select Refresh to update the window. Select the checkbox next to the project and click Finish. This will load the project into CCS workspace.
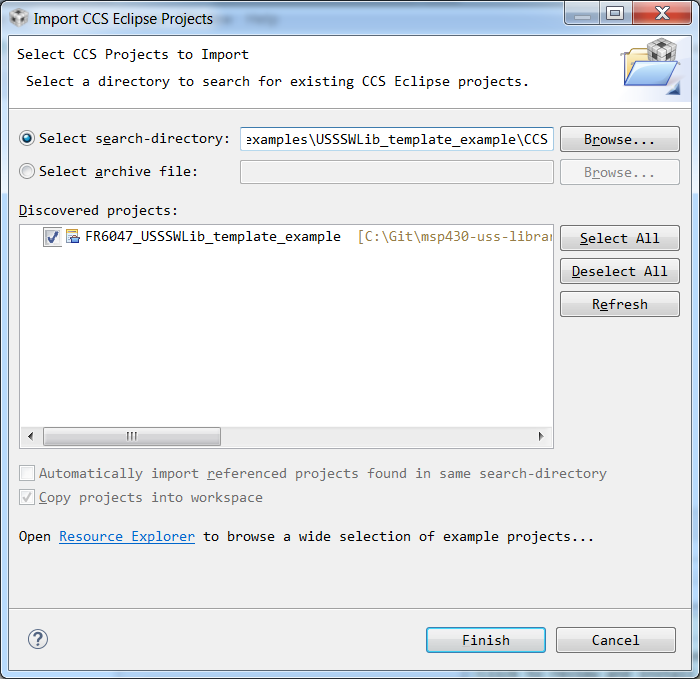
Fig. 2 Importing in CCS
Building the Project¶
To build the project, right-click on the project name in the Project Explorer and select Build Project OR click on the Build icon in the top toolbar.
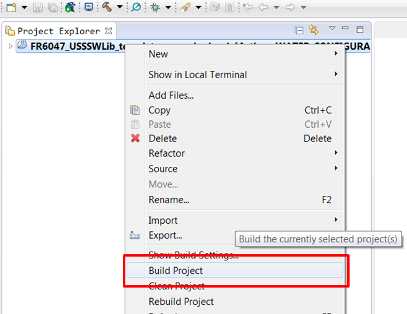
Fig. 3 Building in CCS
IAR Embedded Workbench for MSP430¶
Importing the Project¶
All code examples that are shipped with the USS SW Library come with a .eww IAR workspace file. To import a project into IAR, navigate to the project’s IAR directory <USS_SW_LIBRARY_INSTALL_DIR>\examples\USSSWLib_template_example>
and double-click on the .eww workspace file for that project.
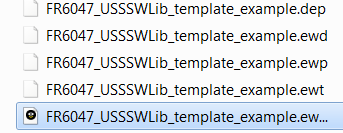
Fig. 4 Importing in IAR
Building the Project¶
Make / Build the project by right-clicking on the project in your Workspace and selecting Make or by clicking Shortcut F7 on your keyboard.
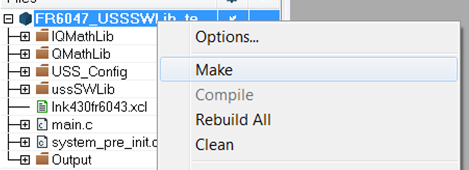
Fig. 5 Building in IAR
Recommended USS SW Library usage¶
Recommended measurement and algorithms initialization¶
Users can decide to use to use the provided Absolute Time Of Flight, Delta Time of Flight and Volume Flow as part of the USS SW Library or implement custom algorithms using the sampled waveforms.
The following diagrams shows the recommended measurement configuration sequence.
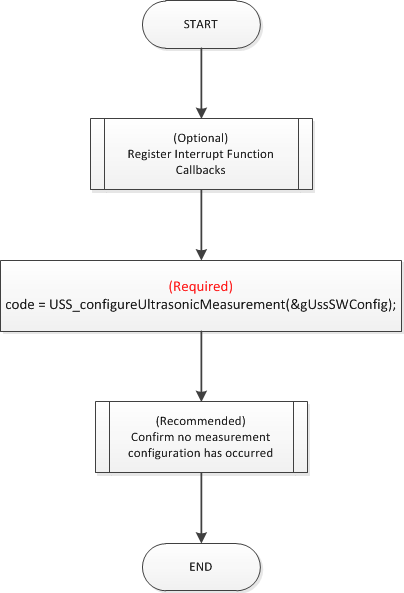
Fig. 6 Measurement Configuration
Before invoking USS_runAlgorithms or USS_runAlgorithmsFixedPoint it is highly recommended to follow the configuration sequence below.
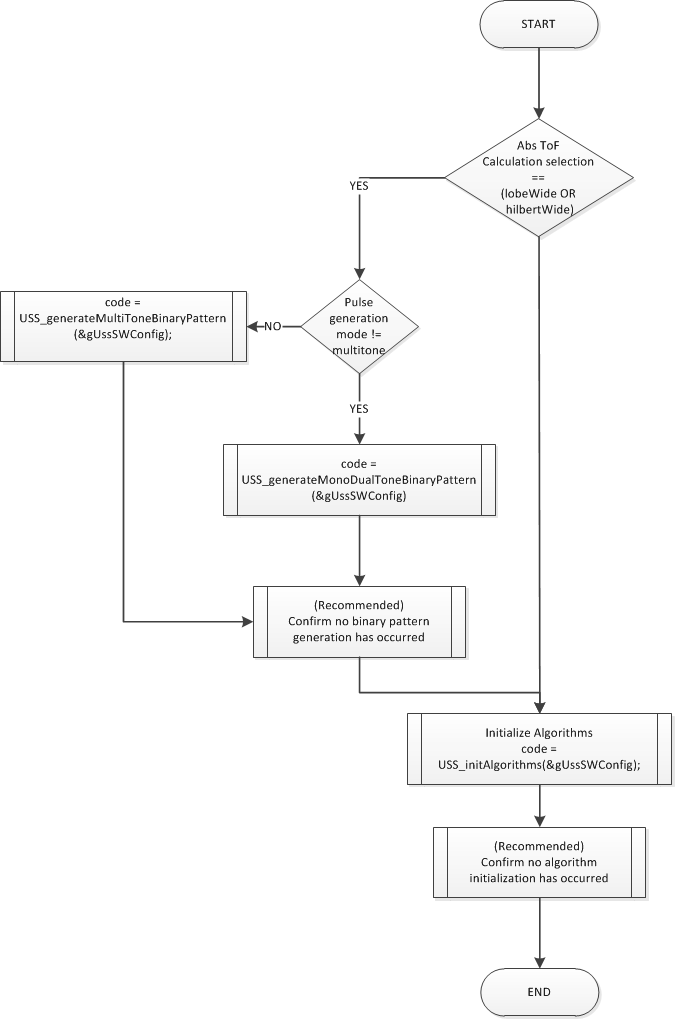
Fig. 7 Measurement Configuration
Most API supported by the library will return an error code back to the application which the user can use to determine if the there was an error detected when invoking an API. All measurement configurations and algorithm initialization APIs will return USS_message_code_no_error
if the API ran successfully.
Modifying Library Configuration Files¶
All modifications to USS SW Library are done via <USS_userConfig.h>
file. This header file in four main sections:
- Library memory optimization configuration
- Library basic configuration parameters
- Clock Definition
- Diagnostic Configuration
- Meter Configuration
- Ultrasonic Firing/Capture Configuration
- Calibration Configuration
- Algorithm Configuration
- Library advanced configuration parameters
- Advanced Algorithm Configuration
- Advanced Ultrasonic Firing/Capture Configuration
- Library Derived parameter
Library memory optimization configuration¶
This section allows user to optimize LEA RAM, RAM and FRAM library memory usage by allowing users to modify various temporary buffers sizes during compilation time.
USS SW Library Macro Name |
---|
USS_SW_LIB_APP_MAX_CAPTURE_SIZE |
USS_SW_LIB_APP_MAX_FILTER_LENGTH |
USS_SW_LIB_APP_MAX_FILTER_OPTIONS |
USS_SW_LIB_APP_MAX_HILBERT_FILTER_OPTIONS |
USS_SW_LIB_APP_MAX_HILBERT_FILTER_LENGTH |
USS_BINARY_ARRAY_MAX_SIZE |
USS_NUM_OF_MAX_TRILL_PULSES |
USS_NUM_OF_MAX_ADDTL_TRILL_PULSES |
The sections below list all temporary buffer used by the library and the. The buffers listed below are defined in <USS_userConfig.c>
LEA RAM Buffers¶
LEA RAM Buffer Name | Data type | Default Configuration Buffer size | Comment |
---|---|---|---|
gUSSLEATempMemBlock | int16_t | [2*(USS_SW_LIB_APP_MAX_CAPTURE_SIZE +USS_SW_LIB_APP_MAX_FILTER_LENGTH)] | Required for Firing/Capture Process |
gUSSLEARAMReservedBlock1 | int16_t | For dtoFCalcOption = USS_Alg_dToF_Calculation_Option_water,
|
Used by windowing algorithm based on dtoFCalcOption:
|
gUSSLEARAMReservedBlock2 | int16_t | [USS_SW_LIB_APP_MAX_CAPTURE_SIZE + USS_SW_LIB_APP_MAX_FILTER_LENGTH] | Used by algorithm absToFOption:
|
gUSSBinaryPattern | int16_t | USS_BINARY_ARRAY_MAX_SIZE | Used by algorithm absToFOption:
|
FRAM Buffers¶
FRAM Buffer Name | Data type | Default Configuration Buffer size | Comment |
---|---|---|---|
gNVMemBlock | int16_t | [USS_SW_LIB_APP_MAX_CAPTURE_SIZE * 2] | Used by all absToFOption computation options |
gUSSFilterCoeffs | int16_t | [USS_SW_LIB_APP_MAX_FILTER_OPTIONS] * [USS_SW_LIB_APP_MAX_FILTER_LENGTH] | Used by USS_runAlgortihms APIs when signal filtering is enabled |
gUSSHilbertCoeffs | int16_t | [USS_SW_LIB_APP_MAX_HILBERT_FILTER_OPTIONS] * [USS_SW_LIB_APP_MAX_HILBERT_FILTER_LENGTH] | Used by algorithm absToFOption:
|
|
uint16_t | [USS_NUM_OF_MAX_TRILL_PULSES] | Required when using multitone generation functionality on devices with SAPH_A module. To determine is your device has SAPH or SAPH_A module please refer to the device datasheet. |
|
uint16_t | [USS_NUM_OF_ADDTL_TRILL_PULSES] | Required when using multitone generation functionality on devices with SAPH_A module and (USS_NUM_OF_ADDTL_TRILL_PULSES > 0). To determine is your device has SAPH or SAPH_A module please refer to the device datasheet. |
additionalBinarySequence | int16_t | [USS_NUM_OF_ADDTL_TRILL_PULSES] | Required when (USS_NUM_OF_ADDTL_TRILL_PULSES > 0) |
RAM Buffers¶
RAM Buffer Name | Data type | Default Configuration Buffer size | Comment |
---|---|---|---|
RAM_XE | uint16_t | [(USS_NUM_OF_MAX_TRILL_PULSES + USS_NUM_OF_ADDTL_TRILL_PULSES) * 6] | Required when using multitone generation functionality on devices with SAPH_A module. To determine is your device has SAPH or SAPH_A module please refer to the device datasheet. |
RAM_DMA_CONFIG | uint16_t | [(USS_NUM_OF_MAX_TRILL_PULSES + USS_NUM_OF_ADDTL_TRILL_PULSES) * 18] | Required when using multitone generation functionality on devices with SAPH_A module. To determine is your device has SAPH or SAPH_A module please refer to the device datasheet. |