COMPA¶
Description¶
Continuous time comparator
The COMPA peripheral is a high-performance continuous time comparator that supports:
- Positive input from:
- Analog-capable I/O pins
- Negative input/reference from:
- Analog-capable I/O pins
- Internal chip voltages (see the
COMPA_REF_XYZ
constants) - Reference DAC
The ISRC peripheral can be used to drive a current into the external node that the COMPA positive input signal is connected to.
The Reference DAC can be used to precharge the external node that the COMPA positive input signal is connected to. Note that any resistive load on this external node will affect the voltage generated by the Reference DAC. Also note that the Reference DAC can only drive one reference / external node at a time.
Capacitive Touch¶
For capacitive touch sensing, COMPA is used together with the ISRC and TDC peripherals. ISRC will then drive an internal reference voltage for COMPA, nominally 0.8 V, while charging the capacitance that is also connected to the COMPA input. The time from start of charging until the COMPA output goes high is measured using the TDC.
The 0.8 V internal reference voltage generated by ISRC can be changed by:
- Connecting the COMPA reference node through an external resistor to ground, in parallel with the internal 400 kOhm resistor
- Disconnecting the internal 400 kOhm resistor
Block Diagram¶
The block diagram shows signal routing for COMPA and ISRC (with I/O pins for 7x7 RGZ package):
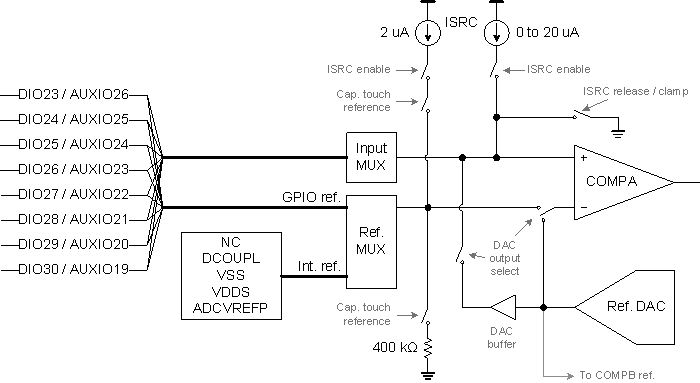
Examples¶
Reading COMPA Output¶
// Enable COMPA
compaEnable(COMPA_PWRMODE_ANY);
// Select COMPA reference and input
compaSelectGpioRef(AUXIO_A_REFERENCE);
compaSelectGpioInput(AUXIO_A_SIGNAL);
... Do other tasks while the COMPA output stabilizes
// Check the COMPA output
compaGetOutput(output.value);
// Disable COMPA
compaDisable();
Capacitive Touch Sensing¶
// Enable COMPA, including 2 uA through 400 kOhm = 0.8 V reference voltage for COMPA
compaEnableWithCapTouchRef();
// Enable ISRC (6.5 uA)
U16 current = BV_ISRC_CURR_2P0U | BV_ISRC_CURR_4P5U;
isrcEnable(current);
// Select 2 x 48 MHz from RCOSC_HF as TDC counter clock source
tdcSetCntSource(TDC_CNTSRC_96M_RCOSC);
// Enable the TDC with start trigger on ISRC reset release and stop trigger on COMPA
tdcSetTriggers(TDC_STARTTRIG_ISRC_RELEASE, TDC_STOPTRIG_COMPA_HIGH, 0);
tdcEnable();
// For each pin ...
for (U16 n = 0; n < PIN_COUNT; n++) {
// Select COMPA input/ISRC output
compaSelectGpioInput(cfg.pAuxioAxdCapTouch[n]);
// Prepare the TDC and trigger start of measurement
tdcArm(TDC_START_ASYNC);
isrcRelease(cfg.pAuxioAxdCapTouch[n]);
// Wait for the TDC stop trigger for 80 us
tdcWaitUs(80);
// Re-clamp the pin to ground
isrcClamp(cfg.pAuxioAxdCapTouch[n]);
// Get the TDC counter value
tdcGetValue(output.pTdcValueH[n], output.pTdcValueL[n]);
}
// Disable COMPA, ISRC and TDC
tdcDisable();
isrcDisable();
compaDisable();
Output Signal Observation¶
// Connect the COMPA output to a GPIO pin
obsSelectSignal(OBS_AUX_COMPA);
obsConnectSignalToGpio(AUXIO_O_OBS);
Procedures Overview¶
Name | Brief description |
compaConnectCapTouchRefIntRes() |
Connects the internal 400 kOhm resistor for the COMPA 0.8 V capactive touch reference. More … |
compaDisable() |
Disables the COMPA peripheral. More … |
compaDisconnectCapTouchRefIntRes() |
Disconnects the internal 400 kOhm resistor for the COMPA 0.8 V capactive touch reference. More … |
compaEnable() |
Enables the COMPA peripheral. More … |
compaEnableWithCapTouchRef() |
Enables the COMPA peripheral with 0.8 V (nominal) reference generated by ISRC. More … |
compaGetOutput() |
Returns whether the COMPA output is low (input below reference) or high (input above reference). More … |
compaSelectGpioInput() |
Selects a GPIO pin as input for COMPA. More … |
compaSelectGpioRef() |
Selects a GPIO pin as reference for COMPA. More … |
compaSelectIntRef() |
Selects an internal voltage source as reference for COMPA. More … |
Constants¶
Name | Description |
COMPA_PWRMODE_ACTIVE |
Power mode support: COMPA can be used in active power mode only |
COMPA_PWRMODE_ANY |
Power mode support: COMPA can be used in any power mode |
COMPA_REF_ADC_REF |
Use the ADC reference as COMPA reference |
COMPA_REF_DCOUPL |
Use the DCOUPL pin as COMPA reference |
COMPA_REF_NONE |
Use no COMPA reference |
COMPA_REF_VDDS |
Use the VDDS pin as COMPA reference |
COMPA_REF_VSS |
Use the VSS/GND pin as COMPA reference |
Global Variables¶
None.
Procedures¶
compaConnectCapTouchRefIntRes¶
Prototype: compaConnectCapTouchRefIntRes()
Connects the internal 400 kOhm resistor for the COMPA 0.8 V capactive touch reference.
If another resistor is connected to an analog GPIO, this allows for switching between two COMPA reference voltages.
compaDisconnectCapTouchRefIntRes¶
Prototype: compaDisconnectCapTouchRefIntRes()
Disconnects the internal 400 kOhm resistor for the COMPA 0.8 V capactive touch reference.
If another resistor is connected to an analog GPIO, this allows for switching between two COMPA reference voltages.
compaEnable¶
Prototype: compaEnable(#pwrModeSupport)
Enables the COMPA peripheral.
Parameter value(s)¶
- #pwrModeSupport : Support use of COMPA in any power mode (COMPA_PWRMODE_ANY) or active power mode only (COMPA_PWRMODE_ACTIVE)
compaEnableWithCapTouchRef¶
Prototype: compaEnableWithCapTouchRef()
Enables the COMPA peripheral with 0.8 V (nominal) reference generated by ISRC. This is used in capacitive touch sensing.
Because of the ISRC dependency, this functionality can only be used in active mode.
compaGetOutput¶
Prototype: compaGetOutput(value)
Returns whether the COMPA output is low (input below reference) or high (input above reference).
Return value(s)¶
- value : The comparator output value, 0 if low, 1 if high
compaSelectGpioInput¶
Prototype: compaSelectGpioInput(auxio)
Selects a GPIO pin as input for COMPA.
The same pin is used as output for ISRC.
Parameter value(s)¶
- auxio : External input selection (index of AUX I/O pin)
compaSelectGpioRef¶
Prototype: compaSelectGpioRef(auxio)
Selects a GPIO pin as reference for COMPA.
Any previous reference source, including the Reference DAC, is disconnected before the GPIO pin is connected.
Parameter value(s)¶
- auxio : External reference selection (index of AUX I/O pin)
compaSelectIntRef¶
Prototype: compaSelectIntRef(intRef)
Selects an internal voltage source as reference for COMPA.
Any previous reference source, including the Reference DAC, is disconnected before the internal voltage source is connected.
Parameter value(s)¶
- intRef : Internal reference selection (COMPA_REF_XYZ).